Table of Contents
Programming is not just about writing code; it’s about solving problems and turning ideas into reality. Today, I want to share a personal journey and show you some exciting programs I’ve created using Python. This post is part of the Code series, designed to inspire and help you learn Python in a practical and enjoyable way.
My Journey Into Programming
When I was in my second year of college, I knew C and C++ quite well. I could write conditional programs and had developed some cool projects. Despite my efforts, I struggled to land a role in the tech industry, likely because I couldn’t find projects aligned with my skills in C or C++.
Then, I discovered Python—a language that changed my perspective on programming. Python opened doors to freelancing opportunities, including web scraping, GUI development, and creating Flask applications. Python not only taught me the practical uses of programming but also why it’s so valued in the industry.
From College Projects to Industry Challenges
When I was in my second year of college, I was well-versed in C and C++, languages that provided a strong foundation in programming. I had created numerous programs and developed products, especially conditional-related applications, using these languages. Despite my efforts, I found myself unable to secure a place in the tech industry. It wasn’t because C or C++ lacked power—far from it—but because I struggled to find relevant projects or opportunities that aligned with these languages.
Discovering Python: A Turning Point
Everything changed when I was introduced to Python. Python didn’t just teach me a new syntax or a set of libraries—it showed me the real-world applications of programming. Through Python, I ventured into freelancing, tackling projects like web scraping, building GUIs, and developing Flask applications. It was a revelation.
Python answered questions I had long pondered:
- Why is programming so valuable in the industry?
- What makes a language widely adopted across domains?
Amazing Python Programs You’ll Learn in This Series
In today’s post, I’ll introduce you to five Python programs that showcase the power and versatility of programming. These projects are fun, practical, and sure to amaze you!
The Magic of Python: Practical and Fun Programs
In today’s blog, I’ll introduce you to five programs I created using Python. These programs are practical, fun, and demonstrate the immense potential of programming.
1. Jarvis: A Virtual Assistant
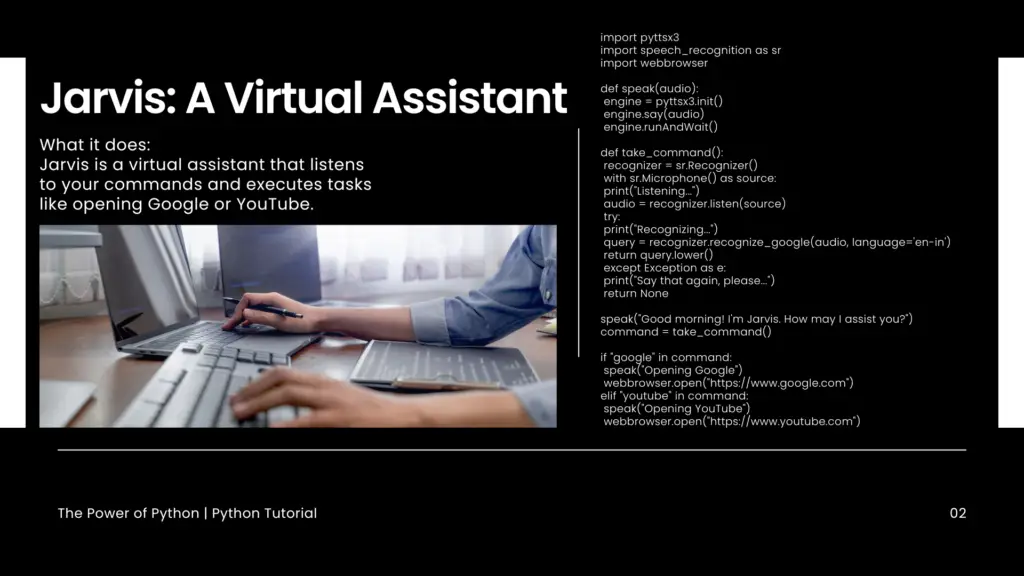
Jarvis is a virtual assistant that takes your voice commands and executes tasks like opening websites or applications. For example:
- “Jarvis, open Google”
- “Jarvis, open YouTube”
What it does:
Jarvis is a virtual assistant that listens to your commands and executes tasks like opening Google or YouTube.
import pyttsx3
import speech_recognition as sr
import webbrowser
def speak(audio):
engine = pyttsx3.init()
engine.say(audio)
engine.runAndWait()
def take_command():
recognizer = sr.Recognizer()
with sr.Microphone() as source:
print("Listening...")
audio = recognizer.listen(source)
try:
print("Recognizing...")
query = recognizer.recognize_google(audio, language='en-in')
return query.lower()
except Exception as e:
print("Say that again, please...")
return None
speak("Good morning! I'm Jarvis. How may I assist you?")
command = take_command()
if "google" in command:
speak("Opening Google")
webbrowser.open("https://www.google.com")
elif "youtube" in command:
speak("Opening YouTube")
webbrowser.open("https://www.youtube.com")
With a simple command, Jarvis gets the job done. It’s built using Python libraries that integrate with Windows APIs, showcasing how programming can make daily tasks easier and more interactive.
2. Love Calculator
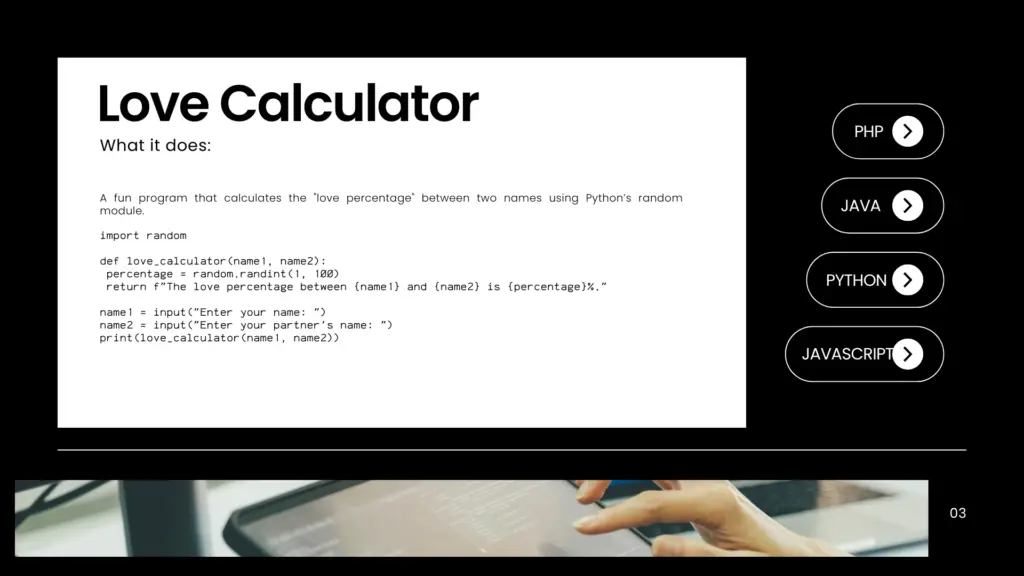
A fun project that calculates the “love percentage” between two names using Python’s random
module. While it’s not a scientific tool, it’s a delightful program that brings a smile to users. Enter two names, and it generates a love score based on randomness. A playful way to explore programming logic and randomness in Python.
What it does:
A fun program that calculates the “love percentage” between two names using Python’s random module.
import random
def love_calculator(name1, name2):
percentage = random.randint(1, 100)
return f"The love percentage between {name1} and {name2} is {percentage}%."
name1 = input("Enter your name: ")
name2 = input("Enter your partner's name: ")
print(love_calculator(name1, name2))
3. Face Recognition Program
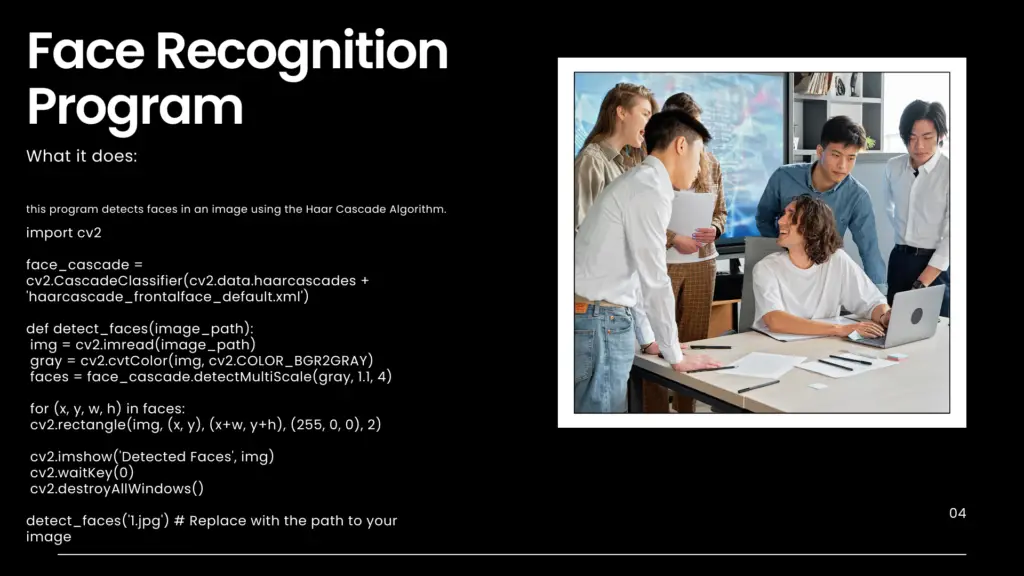
Using the Haar Cascade Algorithm, this program detects faces in images. For instance, when tested with an image of Robert Downey Jr., it accurately identified all the faces. This project demonstrates the power of Python in computer vision and image processing, opening doors to fields like artificial intelligence and machine learning.
What it does:
This program detects faces in an image using the Haar Cascade Algorithm.
import cv2
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
def detect_faces(image_path):
img = cv2.imread(image_path)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
for (x, y, w, h) in faces:
cv2.rectangle(img, (x, y), (x+w, y+h), (255, 0, 0), 2)
cv2.imshow('Detected Faces', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
detect_faces('1.jpg') # Replace with the path to your image
4. Flappy Bird Game
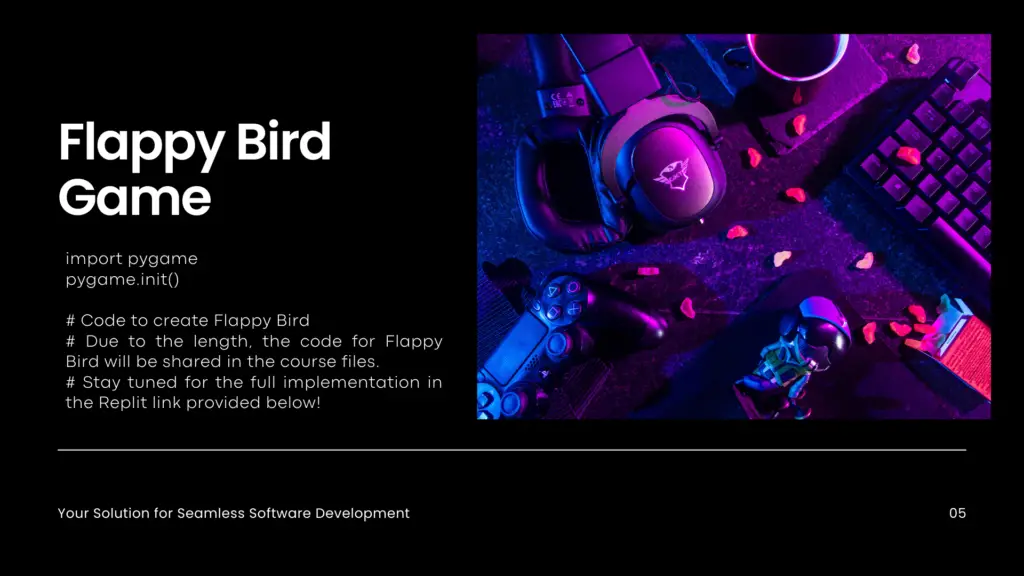
What it does:
Recreate the nostalgic Flappy Bird game from scratch using Python’s pygame library.
import pygame
pygame.init()
# Code to create Flappy Bird
# Due to the length, the code for Flappy Bird will be shared in the course files.
# Stay tuned for the full implementation in the Replit link provided below!
Who doesn’t love Flappy Bird? This classic game, recreated in Python, allows you to enjoy gaming while understanding the logic behind its design. It’s a fresh perspective—playing a game you built yourself is immensely satisfying and educational.
5. Snake Game
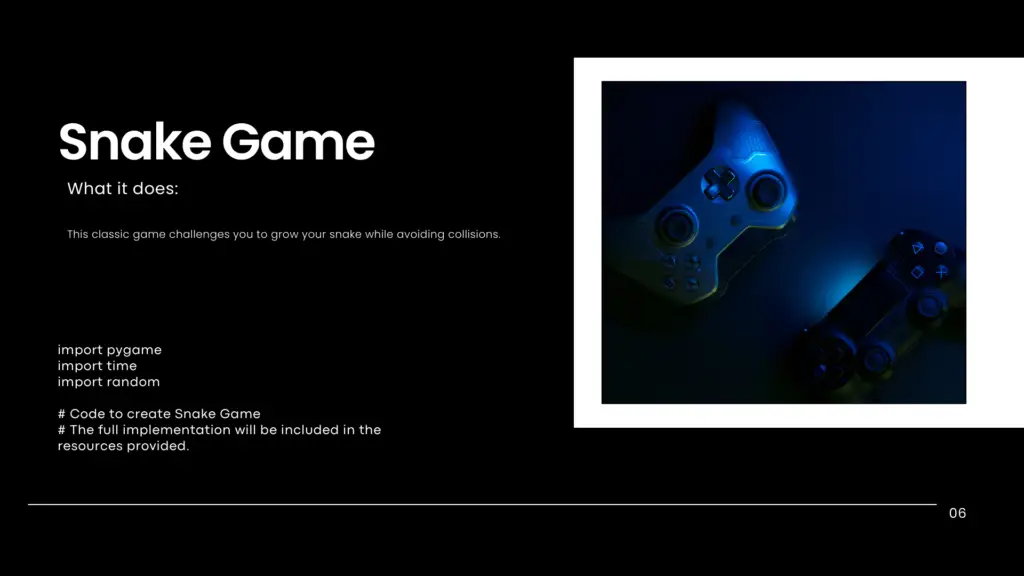
A timeless classic, the Snake game is another project I developed. The black dot represents the snake, and the red dot is the food. While the visuals are basic, the logic behind it is robust. I plan to enhance it further with better graphics. Creating and playing this game taught me the joy of building something from scratch and improving upon it.
What it does:
This classic game challenges you to grow your snake while avoiding collisions.
import pygame
import time
import random
# Code to create Snake Game
# The full implementation will be included in the resources provided.
Learning and Progressing Together
One of the biggest objectives of this Code series is to introduce you to Python’s latest features and approaches. Python is constantly evolving, and we’ll explore these advancements together. For instance:
- The Walrus Operator (
:=
) allows for assignment expressions, making code cleaner and more efficient. - New Python features simplify tasks that were previously cumbersome.
This course will guide you step by step, ensuring you not only learn Python but also master it like a snake charmer hypnotizes a snake.
Your Turn to Code
I’m sharing all five programs with you so you can run them on your system. Even if you encounter issues, don’t worry. As we progress through this course, you’ll gain the skills to fix errors, install necessary modules, and understand the inner workings of each program.
A few tips:
- Install dependencies using
pip install
. - Don’t rush to recreate the programs from scratch—it can be frustrating without a solid foundation.
- Bookmark this series and follow it daily to ensure you don’t miss a step.
A Journey Worth Taking
Python is like a snake charmer’s flute—it lets you control and create amazing programs. Whether it’s building a virtual assistant, creating games, or solving real-world problems, Python makes it all possible. Stick with this series, and together, we’ll turn your ideas into reality.