Python is a popular and powerful programming language that can be used for various purposes, such as web development, data analysis, machine learning, and more. In this blog post, we will learn about some basic concepts of Python that are essential for beginners: comments, escape sequences, and print statement.
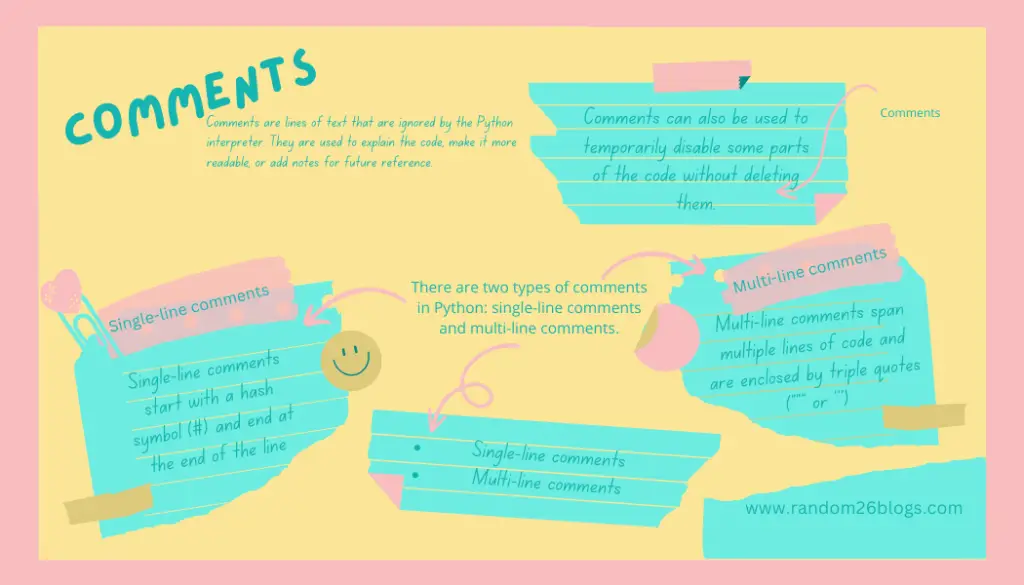
Comments
Comments are lines of text that are ignored by the Python interpreter. They are used to explain the code, make it more readable, or add notes for future reference. Comments can also be used to temporarily disable some parts of the code without deleting them.
There are two types of comments in Python: single-line comments and multi-line comments.
Single-line comments
Single-line comments start with a hash symbol (#) and end at the end of the line. For example:
# This is a single-line comment
print("Hello, world!") # This is another single-line comment
Multi-line comments
Multi-line comments span multiple lines of code and are enclosed by triple quotes (“”” or ‘’’). For example:
"""
This is a multi-line comment
It can have multiple lines of text
"""
print("Hello, world!")
Escape Sequences
Escape sequences are special characters that have a different meaning when preceded by a backslash (). They are used to represent characters that are not printable or have a special function in Python, such as quotes, newlines, tabs, etc.
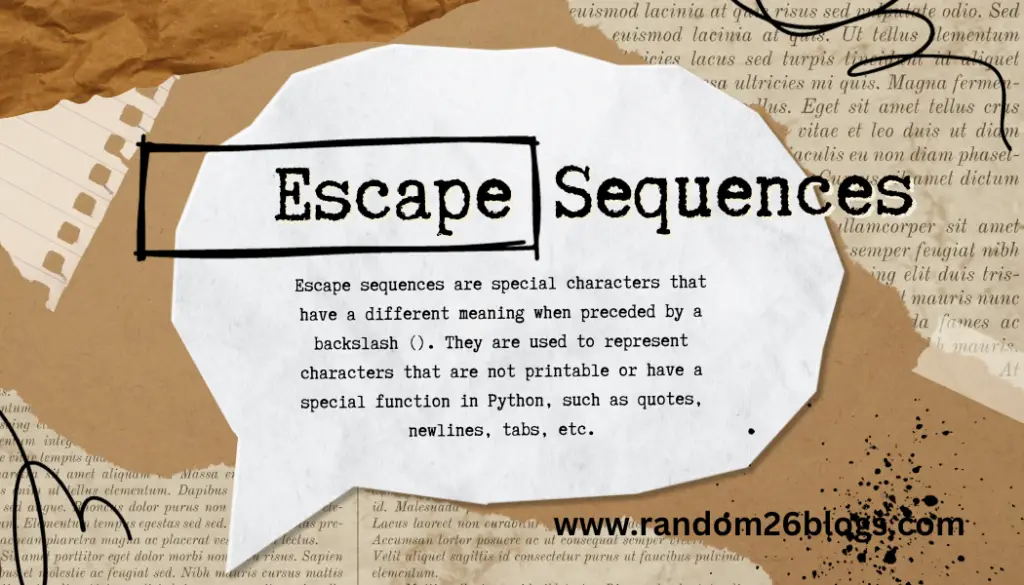
Some common escape sequences in Python are:
\\
: Backslash\'
: Single quote\"
: Double quote\n
: Newline\t
: Tab\b
: Backspace
For example:
print("This is a \"double quote\" inside a string")
print('This is a \'single quote\' inside a string')
print("This is a \nnewline")
print("This is a \ttab")
print("This is a \bbackspace")
The output of the above code is:
This is a "double quote" inside a string
This is a 'single quote' inside a string
This is a
newline
This is a tab
This is abackspace
We can also use the raw string notation to print escape sequences in Python. We just need to add the letter r
before the opening quote of the string. For example:
print(r"This is a \nnewline")
The output of the above code is:
Hello world !
The print statement can also take some optional parameters, such as sep
, end
, and file
. These parameters can be used to customize the output format and destination.
- The
sep
parameter specifies the separator between the arguments. The default value is a space. For example:
print("Hello", "world", "!", sep="-")
The output of the above code is:
Hello-world-!
- The
end
parameter specifies what to print at the end of the output. The default value is a newline character (\n). For example:
print("Hello", end=" ")
print("world", end="!")
print()
The output of the above code is:
Hello world!
- The
file
parameter specifies where to print the output. The default value issys.stdout
, which is the standard output device. We can also use other file objects, such assys.stderr
or custom files. For example:
import sys
print("This goes to stdout", file=sys.stdout)
print("This goes to stderr", file=sys.stderr)
f = open("output.txt", "w")
print("This goes to output.txt", file=f)
f.close()
The output of the above code is:
This goes to stdout
This goes to stderr (in red color)
(output.txt contains "This goes to output.txt")
That’s all for this blog post!!!! I hope you have learned something new and useful about comments, escape sequences, and print statement in Python. If you have any questions or feedback, please feel free to leave a comment below. Thank you for reading!
[…] embark on a Force business, you’ll need USDT on the POLYGON network, a stablecoin always equal to the dollar. Simple instructions can guide you, and blockchain wallets […]
Wow, marvelous blog layout! How long have you ever been running
a blog for? you make blogging glance easy. The overall look of your web site is excellent,
as smartly as the content! You can see similar: najlepszy sklep and here sklep internetowy
Wow, superb weblog structure! How lengthy have you ever been blogging for?
you make running a blog glance easy. The whole look of your web site is wonderful,
as well as the content! You can see similar: sklep and here
sklep online