Python is one of the most popular and versatile programming languages in the world. It can be used for web development, data analysis, machine learning, game development, and more. But how does Python work? How can you use it to create amazing applications? In this blog post, we will explore some of the basic concepts of Python, such as pips and modules, and how they can help you write better code.
Table of Contents
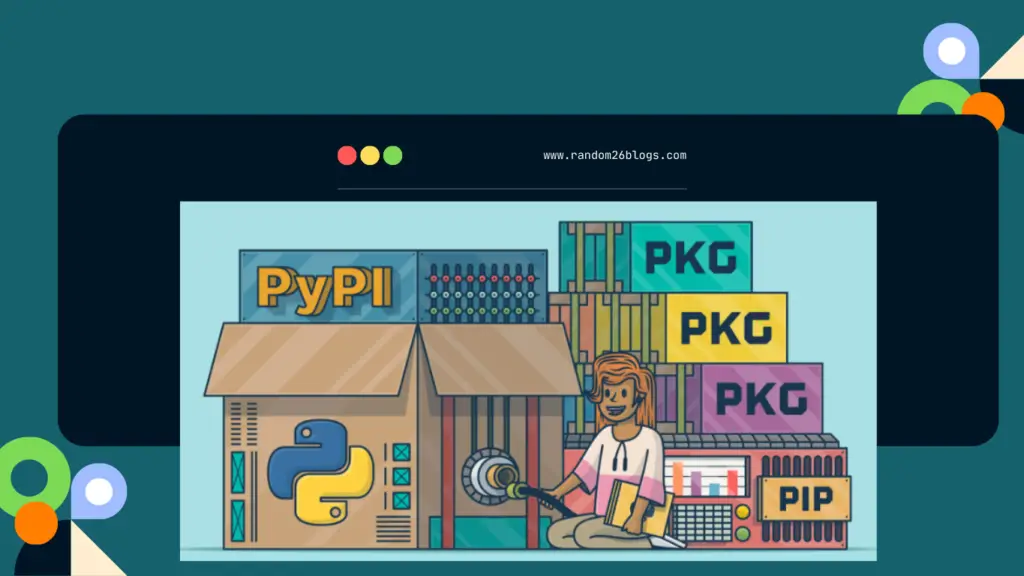
What is Python?
Python is an interpreted, high-level, general-purpose programming language. It was created by Guido van Rossum in 1991 and named after the comedy show Monty Python’s Flying Circus. Python has a simple and elegant syntax that makes it easy to read and write. It also supports multiple programming paradigms, such as object-oriented, procedural, functional, and imperative. Python has a large and comprehensive standard library that provides built-in modules for common tasks, such as file handling, networking, math, and more.
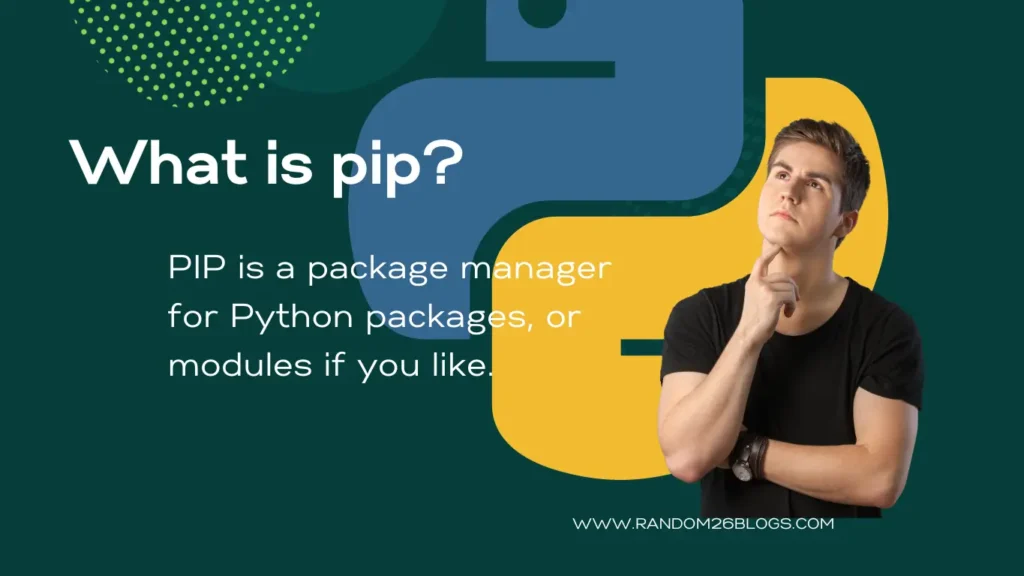
What are Pips?
Well, pips are also the name of a tool that helps you install and manage additional packages or libraries for Python. Pips stands for Pip Installs Packages or Pip Installs Python. Pips are not part of the standard library, but they are widely used by Python developers to extend the functionality of their programs.
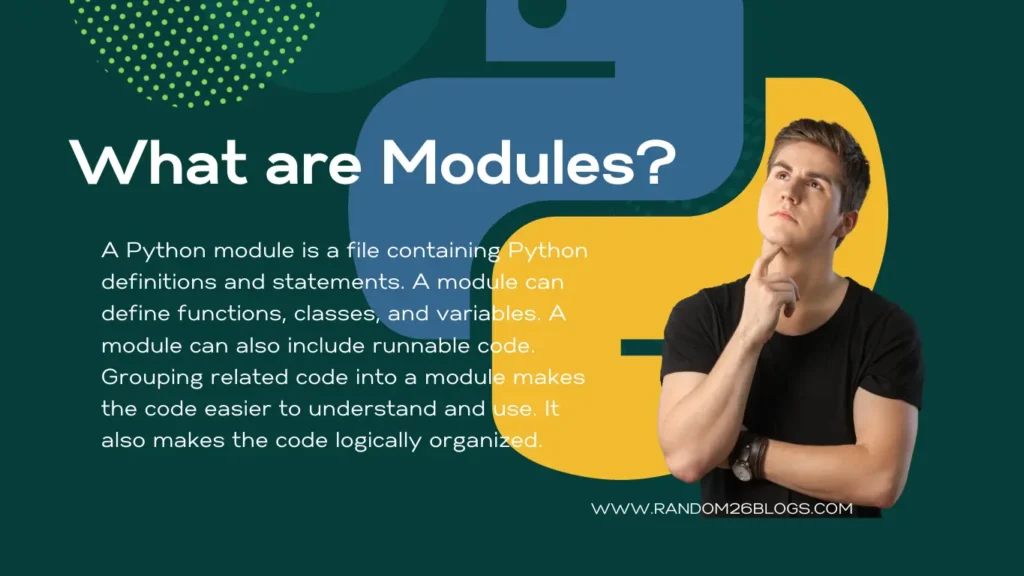
What are Modules?
Modules are files that contain Python code. They can define functions, classes, variables, and other objects that can be imported and used by other modules or scripts. For example, if you have a file called hello.py that contains the following code:
def say_hello(name):
print(f"Hello, {name}!")
You can import this module in another file or in the interactive interpreter and use the say_hello function:
import hello
hello.say_hello("Alice")
# Output: Hello, Alice!
Modules are useful because they allow you to organize your code into reusable and maintainable units. You can also use modules to avoid name conflicts between different variables or functions that have the same name.
How to Use Pips to Install Modules?
One of the advantages of using pips is that you can easily install modules from online repositories, such as PyPI (Python Package Index). PyPI is a website that hosts thousands of open-source projects for Python. You can find modules for almost any purpose, such as web development, data analysis, machine learning, game development, and more.
To use pips to install modules from PyPI, you need to have pips installed on your computer. You can check if you have pips by typing pip --version
in your terminal or command prompt. If you don’t have pips, you can follow the instructions on how to install it from [here].
Once you have pips installed, you can use the following command to install any module from PyPI:
pip install module_name
For example, if you want to install a module called requests that allows you to make HTTP requests in Python, you can type:
pip install requests
This will download and install the requests module and its dependencies on your computer. You can then import and use it in your Python code:
import requests
response = requests.get("https://www.bing.com")
print(response.status_code)
# Output: 200
How to Use Modules in Your Python Code?
Once you have installed a module using pips or any other method, you can use it in your Python code by importing it. There are different ways to import a module in Python:
- You can import the whole module by using
import module_name
. This will make all the objects defined in the module available under the module name. For example:
import math
print(math.pi)
# Output: 3.141592653589793
- You can import specific objects from a module by using
from module_name import object_name
. This will make only those objects available without the module name. For example:
from math import pi
print(pi)
# Output: 3.141592653589793
- You can import all objects from a module by using
from module_name import *
. This will make all the objects available without the module name. However, this is not recommended, as it can cause name conflicts and make your code less readable. For example:
from math import *
print(pi)
# Output: 3.141592653589793
- You can rename a module or an object by using
import module_name as new_name
orfrom module_name import object_name as new_name
. This can help you avoid name conflicts or make your code more concise. For example:
import math as m
print(m.pi)
# Output: 3.141592653589793
How to Create Your Own Modules?
You can also create your own modules in Python by writing your own code in a file with a .py extension. For example, if you have a file called my_module.py that contains the following code:
def add(a, b):
return a + b
def subtract(a, b):
return a - b
You can import and use this module in another file or in the interactive interpreter:
import my_module
print(my_module.add(2, 3))
# Output: 5
print(my_module.subtract(5, 2))
# Output: 3
You can also use the if __name__ == "__main__"
statement to execute some code only when the module is run as a script, and not when it is imported by another module. For example, if you modify the my_module.py file as follows:
def add(a, b):
return a + b
def subtract(a, b):
return a - b
if __name__ == "__main__":
print("This is my module")
print(add(2, 3))
print(subtract(5, 2))
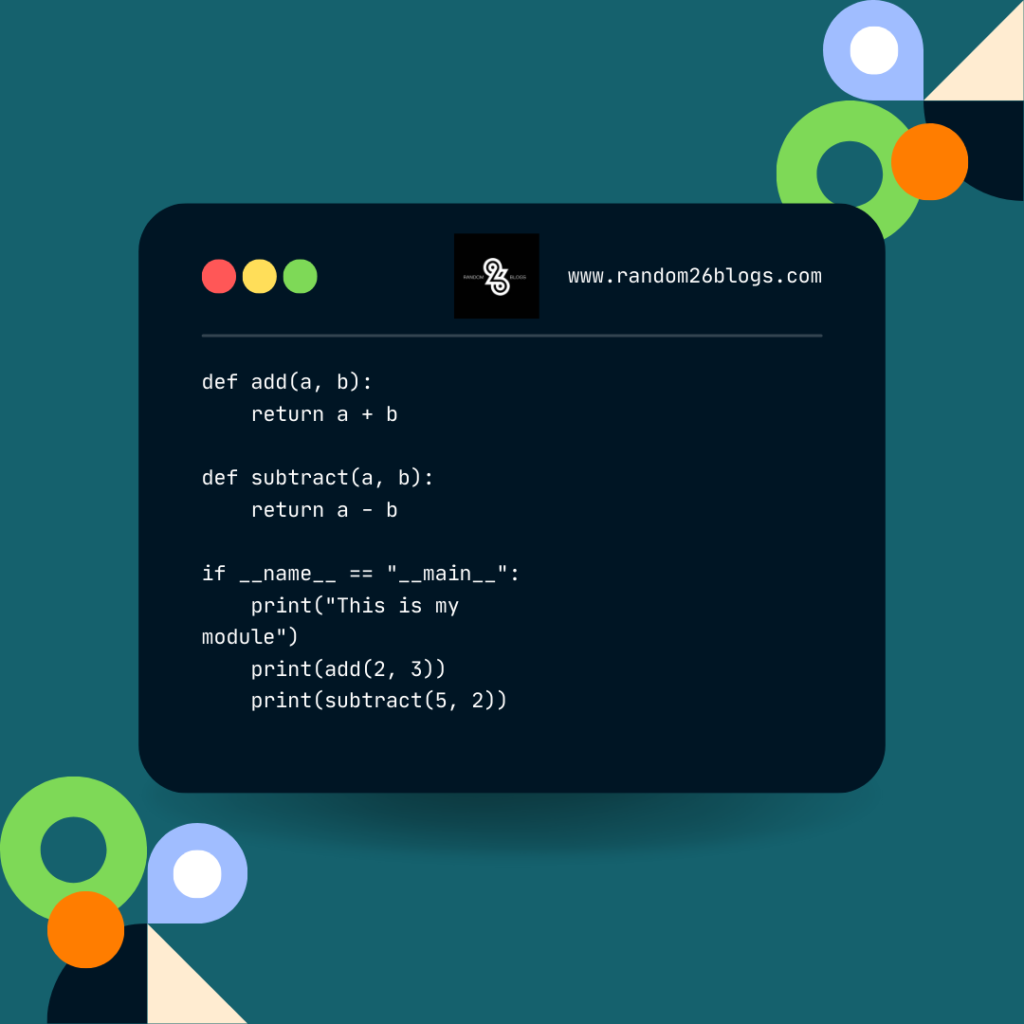
Then, if you run this file as a script, you will see the following output:
python my_module.py
# Output:
This is my module
5
3
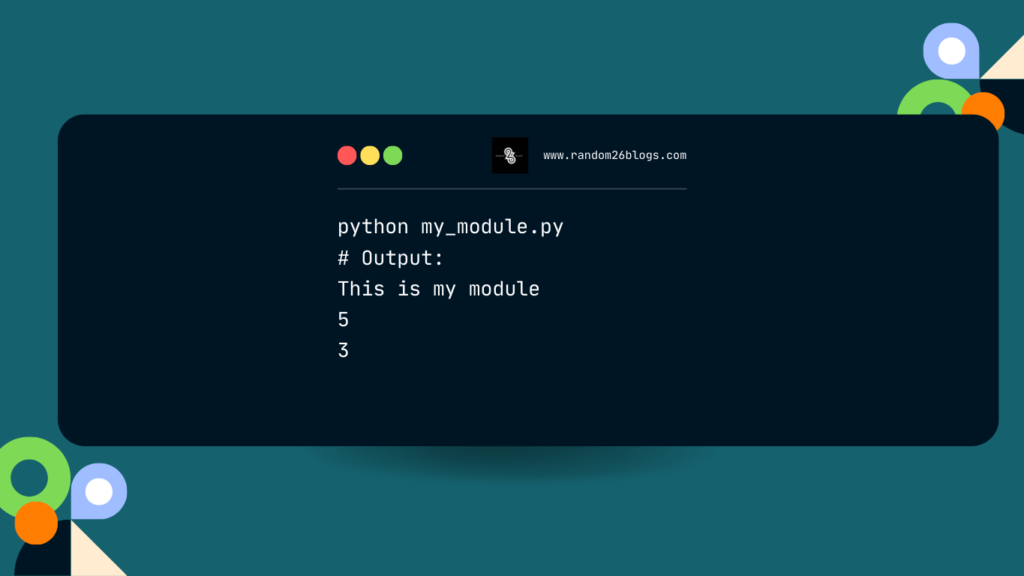
But if you import this module in another file or in the interactive interpreter, you will not see the output:
import my_module
# No output
Key point
In this blog post, we have learned some of the basic concepts of Python, such as pips and modules, and how they can help you write better code. We have seen how to use pips to install modules from online repositories, such as PyPI, and how to import and use modules in our Python code. We have also seen how to create our own modules and execute some code only when the module is run as a script.
We hope that this blog post has been helpful and informative for you. If you have any questions or feedback, please feel free to leave a comment below. Happy coding!
[…] Contact Apple Support: If the issue persists despite trying the above solutions, don’t hesitate to reach out to Apple’s customer support. They can provide personalized assistance and guidance based on your specific situation. […]
First of all I would like to say great blog! I had a quick question which I’d like to ask if you do not mind. I was interested to know how you center yourself and clear your thoughts before writing. I’ve had a difficult time clearing my thoughts in getting my ideas out there. I do enjoy writing however it just seems like the first 10 to 15 minutes are wasted just trying to figure out how to begin. Any suggestions or tips? Many thanks!
[url=https://avana.cfd/]buy dapoxetine cheap[/url]
[url=https://albuterol.cyou/]albuterol uk[/url]
[url=https://baclofen.cfd/]baclofen 10mg tablet[/url]
[url=http://propranolol.cyou/]propranolol order online[/url]
[url=https://synthroid.cyou/]synthroid 25 mg price[/url]
[url=https://finasteride.cyou/]propecia without prescription[/url]
[url=http://amoxicillin.cyou/]amoxicillin drug[/url]
[url=https://retina.cfd/]buy retin a over the counter[/url]
[url=https://albuterol.cfd/]ventolin 50 mg[/url]
[url=http://accutane.cyou/]can you buy accutane over the counter[/url]
[url=https://sildenafil.cyou/]female viagra south africa[/url]
[url=https://doxycycline.guru/]doxycycline hyclate capsules[/url]
[url=http://zithromax.cyou/]buy azithromycin online fast shipping[/url]
[url=http://prednisolone.directory/]can i buy prednisolone over the counter in uk[/url]
[url=https://levitra.cfd/]vardenafil price comparison[/url]
[url=http://tadalafil.cyou/]cialis price comparison[/url]
[url=https://clonidine.cyou/]clonidine buy uk[/url]
[url=https://amoxicillin.boutique/]amoxicillin 325[/url]
[url=http://xenical.cfd/]orlistat online pharmacy[/url]
[url=https://azithromycin.digital/]buy azithromycin usa[/url]
[url=http://fluoxetine.cyou/]fluoxetine 60 mg brand name[/url]
[url=http://furosemide.cyou/]furosemide canada[/url]
[url=https://lisinopril.cfd/]lisinopril 80 mg daily[/url]
[url=http://clonidine.cfd/]clonidine 0.2 mg tablets[/url]
[url=https://trazodone.best/]trazadone[/url]
[url=https://vardenafil.cfd/]buy levitra australia[/url]
[url=http://propecia.cyou/]cheap propecia[/url]
[url=https://albuterol.cfd/]ventolin usa over the counter[/url]
[url=http://clonidine.cyou/]buy clonidine australia[/url]
[url=https://wellbutrin.cfd/]wellbutrin brand cost[/url]
[url=https://robaxin.cyou/]robaxin v[/url]
[url=https://tadalafil.cyou/]can i order cialis online[/url]
[url=https://suhagra.cyou/]suhagra 100 mg[/url]
[url=http://accutane.cyou/]accutane online[/url]
[url=http://augmentin.cfd/]augmentin 875 mg 125 mg tablet[/url]
[url=https://diflucan.cyou/]diflucan 150 price[/url]
[url=https://finasteride.best/]buy generic propecia online canada[/url]
[url=https://retina.directory/]tretinoin 20 mg[/url]
[url=http://ivermectin.guru/]ivermectin cream uk[/url]
[url=https://propecia.cyou/]buy propecia australia[/url]
[url=http://lyrica.cfd/]buy lyrica from canada[/url]
[url=https://albuterol.guru/]ventolin proventil[/url]
[url=http://paxil.cfd/]paroxetine 12.5 price[/url]
[url=http://doxycycline.cfd/]doxycycline 40 mg cost[/url]
[url=https://augmentin.cfd/]amoxil 500mg capsules[/url]
[url=https://azithromycin.digital/]zithromax online pharmacy[/url]
[url=https://doxycycline.directory/]doxycycline order online canada[/url]
[url=http://trazodone.cfd/]trazodone online no prescription[/url]
[url=http://sildenafil.cfd/]buy viagra online in south africa[/url]
[url=http://finasteride.cyou/]propecia online pharmacy usa[/url]
[url=http://augmentin.guru/]augmentin 625[/url]
[url=http://sildenafil.cyou/]cheap canadian viagra online[/url]
[url=https://sildenafil.cyou/]viagra price india[/url]
[url=https://wellbutrin.cfd/]wellbutrin generic price[/url]
[url=https://lisinopril.cfd/]lisinopril 2mg tablet[/url]
[url=http://lexapro.cfd/]lexapro cheap price[/url]
tsmavic.wordpress.com – приворот женщины по фото
отворот сына от невестки, приворот в домашних условиях кто делал отзывы
http://www.magecam.ru – бесплатный приворот мужчины
[url=https://bactrim.cyou/]can you buy bactrim over the counter[/url]
[url=https://hydroxychloroquine.guru/]plaquenil medication[/url]
Hello everybody!
Have you ever heard of X-GPT Writer: a unique keyword content generator based on the ChatGPT neural network?
I also haven’t, until I was advised to automate routine tasks with this software, I want to say one thing! For a long time afterwards, I couldn’t believe
that ChatGPT was such a powerful product if it was used simultaneously in streaming, running X-GPT Writer.
I thought it was just a utility, it was inexpensive, a friend gave me a coupon for a 40% discount%:
94EB516BCF484B27
the details of where to enter it are indicated on the website:
https://www.xtranslator.ru/x-gpt-writer/
I started trying, delving into it, bought 50 ChatGPT accounts at low prices and off I went!
Now I easily generate and launch 3-4 new sites per week, batch unify entire folders and even create images
using the ChatGPT neural network and X-GPT Writer.
It’s worth a try, Friends, there’s a demo, everything is free, you won’t regret it)
Good luck!
ChatGPT и X-GPTWriter для уникальных текстов
ChatGPT как инструмент для генерации контента
Где найти промо коды на X-GPTWriter
Создание контента с помощью X-GPTWriter
Синонимизация текста с X-GPTWriter и ChatGPT
Автоматизированное создание текстов с помощью ChatGPT
X-GPTWriter: мощный инструмент для контент-креации
Создание оригинальных текстов с помощью синонимайзера на базе ChatGPT
автоматическое создание текстов через ChatGPT
Создание уникальных статей с ChatGPT
[url=https://lisinopril.cfd/]cost for 20 mg lisinopril[/url]
[url=http://baclofen.cfd/]baclofen[/url]
[url=https://lisinopril.cfd/]lisinopril tabs[/url]
[url=https://levitra.cfd/]buy levitra generic online[/url]
[url=https://vermox.cyou/]vermox canada otc[/url]
ритуал черного приворота – Обратится к магу – dzen.ru/id/653538d7d6100f7a6fee8469
приворот черная магия на вещи
[url=https://neurontin.cfd/]neurontin 400 mg capsules[/url]
[url=https://lyrica.cfd/]lyrica 125 mg[/url]
Hello
I want to tell you about my experience, how games have turned into real income and help me live!
One day a friend told me about a rather simple but exciting game, thanks to which, after training on a demo account, you can put funds for real bets.
At first I was skeptical about this, but now I have a different opinion!
Lucky jet 1Win – A simple and entertaining game, the guy with the satchel must fly as high as possible and achieve his goals, and these are your bets.
It’s corny, but after a detailed study, I started to win a little and found my stable style of play, the official website has both statistical calculations and classic strategies and hacks!
By the way, anyone who played the legendary Aviator will be delighted with Lucky jet 1Win,there is still the possibility of increased profits!
All information about the gameLucky jet,on the official multilingual website:
English – https://lucky-jetcash.com/en/
Spanish – https://lucky-jetcash.com/es/
Azerbaijanian – https://lucky-jetcash.com/az/
Dutch – https://lucky-jetcash.com/de/
French – https://lucky-jetcash.com/fr/
Portuguese – https://lucky-jetcash.com/pt/
Turkish – https://lucky-jetcash.com/tr/
Kazakh – https://lucky-jetcash.com/kk/
Rus – https://lucky-jetcash.com/
I wish you positivity and winnings in the coming 2024!
See you in the communityLucky jet 1Win!
lucky jet sinais
1win lucky jet
lucky jet crash game
lucky jet reviews
[url=https://lucky-jetcash.com]lucky jet statistic[/url]
[url=https://lucky-jetcash.com]lucky jet official site[/url]
[url=https://lucky-jetcash.com]lucky jet crash game[/url]
[url=https://lucky-jetcash.com]lucky jet signals[/url]
[url=http://hydroxychloroquine.guru/]plaquenil 300 mg[/url]
[url=https://wellbutrin.cfd/]zyban canada[/url]
Hi everyone
I want to tell you about my experience, how games have turned into real income and help me live!
One day a friend told me about a rather simple but exciting game, thanks to which, after training on a demo account, you can put funds for real bets.
At first I was skeptical about this, but now I have a different opinion!
Lucky jet 1Win – A simple and entertaining game, the guy with the satchel must fly as high as possible and achieve his goals, and these are your bets.
It’s corny, but after a detailed study, I started to win a little and found my stable style of play, the official website has both statistical calculations and classic strategies and hacks!
By the way, who played the legendary Aviator will be delighted with Lucky jet 1Win,there is still the possibility of increased profits!
All information about the gameLucky jet,on the official multilingual website:
English – https://lucky-jetcash.com/en/
Spanish – https://lucky-jetcash.com/es/
Azerbaijanian – https://lucky-jetcash.com/az/
Dutch – https://lucky-jetcash.com/de/
French – https://lucky-jetcash.com/fr/
Portuguese – https://lucky-jetcash.com/pt/
Turkish – https://lucky-jetcash.com/tr/
Kazakh – https://lucky-jetcash.com/kk/
Rus – https://lucky-jetcash.com/
I wish you positivity and winnings in the coming 2024!
See you in the communityLucky jet 1Win!
1 win lucky jet
lucky jet 1win
lucky jet играть
lucky jet signals
[url=https://lucky-jetcash.com]lucky jet offers[/url]
[url=https://lucky-jetcash.com]lucky jet reviews[/url]
[url=https://lucky-jetcash.com]lucky jet 1 win[/url]
[url=https://lucky-jetcash.com]lucky jet hack[/url]
[url=http://neurontin.cfd/]gabapentin online uk[/url]
[url=https://albuterol.cyou/]albuterol generic otc[/url]
[url=http://budesonide.cyou/]budesonide 64 mcg[/url]
[url=https://robaxin.cyou/]robaxin 750 cost[/url]
[url=http://onlinepharmacy.cyou/]overseas pharmacy no prescription[/url]
Hello everybody!
How do you feel about computer graphics and design?
Once I started looking at the works of leading designers and it became my hobby, computer graphics allows you to do
beautiful works, design solutions and advertising creatives.
By the way, I noticed that it broadens my horizons, and brings peace to my life!
I recommend profiles of these masters:
https://dprofile.ru/sh_alsina/collection/3728/aidentika
https://dprofile.ru/case/35919/function%20()%20%20%5Bnative%20code%5D%20
https://dprofile.ru/shev/story/3
https://dprofile.ru/case/49060/function%20()%20%20%5Bnative%20code%5D%20
https://dprofile.ru/lunarnia/story/3
https://dprofile.ru/sasheeme/cv
https://dprofile.ru/cases/search?City=1&hasAchievement=all&sort=date&city=984
https://dprofile.ru/yous/collection/4672/3d
https://dprofile.ru/case/33326/function%20()%20%20%5Bnative%20code%5D%20
https://dprofile.ru/anya_dobrota/collection/4443/followers
https://dprofile.ru/users/search?type=personal&sort=followers&filter=3&Filter=107
https://dprofile.ru/users/search?type=personal&sort=followers&Filter=7&city=831
https://dprofile.ru/vetcogray/cv
https://dprofile.ru/case/37490/function%20()%20%20%5Bnative%20code%5D%20
https://dprofile.ru/m00nshadow88/cv
https://dprofile.ru/kurikota/story/3
https://dprofile.ru/case/6094/function%20()%20%20%5Bnative%20code%5D%20
https://dprofile.ru/vostro/cv
https://dprofile.ru/krisplazun/cv
https://dprofile.ru/vicious/story/3
Good luck!
[url=http://vardenafil.directory/]price of levitra 10 mg[/url]
[url=http://bactrim.cyou/]bactrim pill[/url]
[url=http://onlinepharmacy.cyou/]canada pharmacy world[/url]
[url=https://prozac.cfd/]best price for generic prozac[/url]
[url=http://paxil.cfd/]buy paroxetine[/url]
[url=http://fluconazole.cyou/]diflucan usa[/url]
[url=http://fluoxetine.cyou/]fluoxetine 20 mg australia[/url]
[url=http://sildenafil.cfd/]prices for viagra prescription[/url]
[url=https://allopurinol.cfd/]allopurinol 100[/url]
Hello everybody!
How do you feel about computer graphics and design?
Once I started looking at the works of leading designers and it became my hobby, computer graphics allows you to do
beautiful works, design solutions and advertising creatives.
By the way, I noticed that it broadens my horizons, and brings peace to my life!
I recommend profiles of these masters:
https://dprofile.ru/sofya0829/info
https://dprofile.ru/kiselyuxa/info
https://dprofile.ru/n.kungurova.1995/following
https://dprofile.ru/mariinasolopova
https://dprofile.ru/dariamadai
https://dprofile.ru/petrovalz/story/2
https://dprofile.ru/egorov.d.nikita/story/2
https://dprofile.ru/anastasiaden/info
https://dprofile.ru/regina91/story/2
https://dprofile.ru/marta/followers
https://dprofile.ru/dinapetova/following
https://dprofile.ru/cldlne/info
https://dprofile.ru/anastasiya_derevnina/story/2
https://dprofile.ru/maksim_888/followers
https://dprofile.ru/iammkhv/followers
https://dprofile.ru/carrot_witch
https://dprofile.ru/ivmwtd/info
https://dprofile.ru/marinaus/followers
https://dprofile.ru/nastyasue/story/2
https://dprofile.ru/rusotoon/info
Good luck!
Забота о резиденции – это забота о спокойствии. Изоляция наружных поверхностей – это не только модный облик, но и обеспечение сохранения тепла в вашем уютном уголке. Наша бригада, коллектив экспертов, предлагаем вам сделать ваш дом в прекрасное место для жизни.
Наши работы – это не просто утепление, это искусство с каждым слоем. Мы предпочитаем гармонии между внешним видом и практической ценностью, чтобы ваш дом преобразился не только теплым и уютным, но и роскошным.
И самое важное – разумная цена! Мы уверены, что высококачественные услуги не должны быть дорогим удовольствием. [url=https://ppu-prof.ru/]Утепление дома снаружи стоимость[/url] начинается всего от 1250 рублей за квадратный метр.
Использование современных технологий и высококачественных материалов позволяют нам создавать утепление, которое долговечно и надежно. Забудьте о холодных стенах и дополнительных расходах на отопление – наше утепление станет вашим надежным экраном от холода.
Подробнее на [url=https://ppu-prof.ru/]https://www.ppu-prof.ru[/url]
Не откладывайте на потом заботу о удовольствии в вашем доме. Обращайтесь к квалифицированным специалистам, и ваше жилье станет настоящим художественным творением, которое принесет вам тепло и удовлетворение. Вместе мы создадим дом, в котором вам будет по-настоящему удобно!
[url=http://prednisolone.directory/]prednisolone 5mg price uk[/url]
->>>>>>>>>>>>>как приворожить парня чтоб любил<<<<<<<<<-
______________чтобы любимый позвонил заговор _____________
снять наговор самостоятельно с мужчины
как убрать приворот с мужчины, а также:
->>>>>>заклинания любви на девушку
->>>>>>как снять с себя любовный приворот
->>>>>>приворот на пороге читать
->>>>>>заговор на любимую
еврейский приворот
🚀 Wow, this blog is like a cosmic journey launching into the universe of endless possibilities! 💫 The mind-blowing content here is a captivating for the imagination, sparking awe at every turn. 🌟 Whether it’s lifestyle, this blog is a goldmine of exciting insights! #AdventureAwaits 🚀 into this exciting adventure of knowledge and let your mind roam! 🚀 Don’t just explore, savor the excitement! #BeyondTheOrdinary Your mind will be grateful for this exciting journey through the dimensions of endless wonder! 🌍
[url=http://albuterol.cyou/]cheap allbuterol[/url]
[url=https://fluconazole.cyou/]where can i buy diflucan online[/url]
[url=https://neurontin.cyou/]neurontin 900[/url]
[url=https://gabapentin.cfd/]neurontin 500 mg[/url]
[url=https://zoloft.cfd/]generic zoloft 40 mg[/url]
[url=http://doxycycline.cyou/]how to get doxycycline prescription[/url]
[url=https://ivermectin.guru/]stromectol tab[/url]
[url=https://augmentin.best/]cost of augmentin tablets[/url]
Привет всем!
Новинки интим-товаров: обзоры и отзывы!
Хотите узнать о последних тенденциях в мире интим-товаров? https://satisfucktor.ru предлагает вам самые свежие обзоры и
рейтинги секс-игрушек, эротического белья, лубрикантов и многого другого. Наши эксперты тщательно изучают каждый товар,
чтобы помочь вам сделать осознанный выбор. Подробные обзоры и сравнительные характеристики помогут вам найти идеальный
продукт для ваших интимных удовольствий. Посетите Satisfucktor.ru и окунитесь в мир страсти и удовольствия!
[url=https://satisfucktor.ru/obzory/vibr/obzor-pokupatelya-na-vibropulyu-perezaryazhaemuyu-glitz-royal-gems-roy004-shots-media-osnovnye-harakteristiki-instrukcziya-i-uhod/]Обзор покупателя на Надувной вибратор Inflatable G-wand – основные характеристики, инструкция и уход[/url]
[url=https://satisfucktor.ru/obzory/obzor-telesnogo-falloimitatora-mr-strong-19-sm-osobennosti-ispolzovaniya-instruktsiya-preimushestva-i-nedostatki/]Потребительский обзор – “Приятные мелочи Презервативы. Классические презервативы Contex Classic – 3 шт.” – инструкция, преимущества и недостатки – полный разбор от покупателя[/url]
[url=https://satisfucktor.ru/obzory/vibr/obzor-pokupatelya-na-vibrator-realistik-iz-kiberkozhi-s-moshonkoj-%e2%84%9659-osnovnye-harakteristiki-instrukcziya-i-uhod-za-vibrator-realistik-iz-kiberkozhi-s-moshonkoj-%e2%84%9659/]Обзор покупателя на Вибратор-реалистик из киберкожи с мошонкой №59. Основные характеристики, инструкция и уход за Вибратор-реалистик из киберкожи с мошонкой №59[/url]
[url=https://satisfucktor.ru/obzory/bdsm/obzor-na-stek-krasnyj-kozhanyj-instrukcziya-osobennosti-ispolzovaniya-dlya-bdsm-igr-sovety-po-ismolzovani-stek-krasnyj-kozhanyj-dlya-czenitelej-bdsm/]Обзор на Стек с хлопушкой петлей Sitabella – инструкция, особенности использования для БДСМ игр, советы по использованию. Стек с хлопушкой петлей Sitabella для ценителей BDSM[/url]
[url=https://satisfucktor.ru/obzory/bdsm/naruchniki-maya-instrukcziya-osobennosti-ispolzovaniya-sovety-dlya-bdsm-igrnikov/]Обзор на Наручники однослойные кожа Pecado BDSM – инструкция, особенности использования для БДСМ игр, советы по использованию. Наручники однослойные кожа Pecado BDSM для ценителей BDSM[/url]
[url=https://satisfucktor.ru/obzory/vibr/obzor-pokupatelya-na-klassicheskij-silikonovyj-vibrator-4sexdream-osnovnye-harakteristiki-instrukcziya-i-uhod/]Мастурбатор-вагина в колбе для мужчин – обзоры, отзывы и особенности использования реалистичного вибромастурбатора[/url]
[url=https://satisfucktor.ru/masturbator/masturbator-vagina-diana-dlya-muzhchin-realistichnoe-ustrojstvo-dlya-udovletvoreniya-proverennye-obzory-i-otzyvy/]Ролевые костюмы для секса – Мужской костюм Официанта La Blinque. Обзор ролевого белья из магазина для взрослых[/url]
*****
https://satisfucktor.ru/obzory/bdsm/obzor-na-krasnyj-oshejnik-s-kolechkom-instrukcziya-osobennosti-ispolzovaniya-dlya-bdsm-igr-sovety-po-ispolzovaniyu-krasnyj-oshejnik-s-kolechkom-dlya-czenitelej-bdsm/
https://satisfucktor.ru/obzory/lubrikanty/obzor-na-shipuchuyu-sol-dlya-vann-saharnyj-arbuz-candy-bath-bar-laboratoriya-katrin-volshebstvo-dlya-relaksaczii-i-uhoda/
https://satisfucktor.ru/obzory/vibr/obzor-pokupatelya-na-abbott-vibronasadka-na-2-palcza-s-rebristoj-poverhnostyu-baile-pretty-love-osnovnye-harakteristiki-instrukcziya-i-uhod/
https://satisfucktor.ru/obzory/obzor-i-instrukcziya-po-ispolzovaniyu-steklyannyh-i-metallicheskih-analnyh-igrushek-bolshoj-stalnoj-plag-s-chernym-kristallom-black-rave-95-sm-preimushhestva-i-nedostatki/
https://satisfucktor.ru/obzory/lubrikanty/chestnyj-obzor-na-analnuyu-probku-colorful-joy-medium-s-fioletovym-kristallom-instrukcziya-ispolzovaniya-i-sovety-po-uhodu/
https://satisfucktor.ru/obzory/strapon/preimushhestva-i-nedostatki-realistichnoj-nasadki-7-realistic-perfect-erect-cock-185-sm-osobennosti-ispolzovaniya-i-kratkaya-instrukcziya/
https://satisfucktor.ru/obzory/lubrikanty/chestnyj-obzor-na-nabor-analnyh-probok-silicone-curve-anal-kit-kak-ispolzovat-instrukcziya-i-sovety-po-uhodu/
Удачи!
[url=http://retina.directory/]retin a cheapest price[/url]
[url=https://robaxin.cyou/]robaxin online canada[/url]
[url=https://bactrim.cyou/]bactrim 800 160 mg tablet[/url]
[url=https://vardenafil.cyou/]buying levitra[/url]
[url=https://semaglutidewegovy.online/]rybelsus for sale[/url]
Hi everyone
One day my friend showed me an interesting and colorful game, just like from My childhood, fruit combinations, very fascinating and most importantly develops logic and luck, it does not oblige to anything and helps to brighten up the time!
Once you understand how the internal mechanism of the slot works, it’s time to answer the question: “how to win?” Since each round of the game is completely dependent on the random number generator and is unpredictable, it is important to understand which strategy can be most effective and start testing it, preferably in demo mode, so as not to risk real funds. Despite the fact that the mathematical expectation remains negative in general, the chances of winning still exist for each player.
If you want to find out how to win in this wonderful slot, then one of the real solutions may be to set the bet until your balance doubles. It is important not to continue playing if you are unlucky and the money starts to run out.
The best site for playing sweet bonanza – https://sweet-bonanza-game.ru !
In the game, it is important to aim for 4 or more lollipop symbols, as this activates free spins, sweet bombs and multiplayer!
Good luck friends!
[url=https://sweet-bonanza-game.ru]sweet bonanza great.com[/url]
[url=https://sweet-bonanza-game.ru]max win sweet bonanza[/url]
[url=https://sweet-bonanza-game.ru]free spins sweet bonanza[/url]
[url=https://sweet-bonanza-game.ru]sweet bonanxa[/url]
[url=https://sweet-bonanza-game.ru]1xbet sweet bonanza[/url]
casino-bonanza-online
sweet bonanza рубли
sweet bonzana online
sweet bonanza slots
situs sweet bonanza candyland
sweet bonanza casino
play slot bonanza online
[url=https://rybelsus.best/]buy semaglutide online canada[/url]
[url=http://ozempic.quest/]buy ozempic online from india[/url]
[url=https://semaglutide.quest/]wegovy medication[/url]
[url=https://semaglutidewegovy.shop/]rybelsus diabetes medication[/url]
[url=http://semaglutidewegovy.online/]rybelsus tab 7mg[/url]
Hello
One day my friend showed me an interesting and colorful game, just like from My childhood, fruit combinations, very fascinating and most importantly develops logic and luck, it does not oblige to anything and helps brighten up the time!
Once you understand how the internal mechanism of the slot works, it’s time to answer the question: “how to win?” Since each round of the game is completely dependent on the random number generator and is unpredictable, it is important to understand which strategy can be most effective and start testing it, preferably in demo mode, so as not to risk real funds. Despite the fact that the mathematical expectation remains negative in general, the chances of winning still exist for each player.
If you want to find out how to win in this wonderful slot, then one of the real solutions may be to set the bet until your balance doubles. It is important not to continue playing if you are unlucky and the money starts to run out.
The best site for playing sweet bonanza – https://sweet-bonanza-game.ru !
In the game, it is important to aim for 4 or more lollipop symbols, as this activates free spins, sweet bombs and multiplayer!
Good luck friends!
[url=https://sweet-bonanza-game.ru]how to play sweet bonanza in us[/url]
[url=https://sweet-bonanza-game.ru]sweet bonanza real money australia[/url]
[url=https://sweet-bonanza-game.ru]sweetbonanta demo[/url]
[url=https://sweet-bonanza-game.ru]slot sweet bonanza[/url]
[url=https://sweet-bonanza-game.ru]бонанза демо версия[/url]
sweet bonanza slot free play
free spins sweet bonanza
slots sweet bonanza
swetbonanza
play sweet bonanza demo
sweet bonanza app
bonanza slot oyna
Привет всем!
Хочу всем посоветовать лучшую компаний по дезинсекции и уничтожения насекомых в Москве, это действительно помогло мне решить проблемы и начать жить спокойно!
Уничтожение тараканов: эффективные методы, безопасность и профессиональные услуги
Тараканы в доме могут вызывать не только физическое недомогание, но и создавать проблемы с гигиеной и комфортом. Для решения этой неприятной ситуации широко применяются различные методы уничтожения тараканов, включая дезинсекцию и применение инсектицидов.
Профессиональная дезинсекция:
Один из самых эффективных способов избавления от тараканов — профессиональная дезинсекция. Специалисты проводят тщательную обработку помещения с использованием высокоэффективных инсектицидов, которые эффективно уничтожают тараканов на всех стадиях их развития. Профессионалы также применяют индивидуальный подход, учитывая особенности помещения и степень зараженности.
Вот сайт профессионалов дезинсекции: https://dezinfekciya-mcd.ru
[url=https://dezinfekciya-mcd.ru/tarakan/]уничтожение тараканов в магазине[/url]
[url=https://dezinfekciya-mcd.ru/unichtozhenie/plesen/]уничтожение грибка в квартире[/url]
[url=https://dezinfekciya-mcd.ru/unichtozhenie/muravi/]уничтожение муравьев[/url]
Безопасность и гарантия результата:
При проведении уничтожения тараканов специалисты обеспечивают полную безопасность для здоровья жильцов и домашних животных. Инсектициды, используемые при дезинсекции, отличаются низкой токсичностью для людей и животных, при этом обеспечивая высокую эффективность в борьбе с тараканами. Гарантия результата после профессиональной обработки обеспечивает долгосрочную защиту от повторного появления вредителей.
Удачи!
услуги дезинсекции
уничтожение мышей в москве
поморить тараканов
служба травления тараканов
дезинсекция в москве
[url=https://ozempic.company/]ozempic tab 7mg[/url]
[url=http://semaglutide.download/]buy semaglutide[/url]
[url=http://rybelsus.cyou/]semaglutide online uk[/url]
[url=http://rybelsus.best/]buy ozempic online no script[/url]
[url=https://semaglutideozempic.online/]brand semaglutide[/url]
[url=https://semaglutiderybelsus.shop/]buy ozempic online no script needed[/url]
Привет всем!
Хочу всем посоветовать лучшую компаний по дезинсекции и уничтожения насекомых в Москве, это действительно помогло мне решить проблемы и начать жить спокойно!
Уничтожение тараканов: эффективные методы, безопасность и профессиональные услуги
Тараканы в доме могут вызывать не только физическое недомогание, но и создавать проблемы с гигиеной и комфортом. Для решения этой неприятной ситуации широко применяются различные методы уничтожения тараканов, включая дезинсекцию и применение инсектицидов.
Профессиональная дезинсекция:
Один из самых эффективных способов избавления от тараканов — профессиональная дезинсекция. Специалисты проводят тщательную обработку помещения с использованием высокоэффективных инсектицидов, которые эффективно уничтожают тараканов на всех стадиях их развития. Профессионалы также применяют индивидуальный подход, учитывая особенности помещения и степень зараженности.
Вот сайт профессионалов дезинсекции: https://dezinfekciya-mcd.ru
[url=https://dezinfekciya-mcd.ru/unichtozhenie/klopov/]избавиться от клопов в москве[/url]
[url=https://dezinfekciya-mcd.ru/unichtozhenie/plesen/]уничтожение плесени[/url]
[url=https://dezinfekciya-mcd.ru/unichtozhenie/klesch/]обработка участка от клещей цена[/url]
Безопасность и гарантия результата:
При проведении уничтожения тараканов специалисты обеспечивают полную безопасность для здоровья жильцов и домашних животных. Инсектициды, используемые при дезинсекции, отличаются низкой токсичностью для людей и животных, при этом обеспечивая высокую эффективность в борьбе с тараканами. Гарантия результата после профессиональной обработки обеспечивает долгосрочную защиту от повторного появления вредителей.
Удачи!
обработка участка от комаров и клещей
избавиться от клопов в москве
уничтожение тараканов в москве
служба по выведению тараканов
уничтожение клопов с гарантией
[url=http://ozempictabs.com/]rybelsus 3mg[/url]
[url=https://wegovy.trade/]buy rybelsus from canada[/url]
[url=https://rybelsussemaglutide.online/]rybelsus generic cost[/url]
[url=http://rybelsus.monster/]rybelsus 7 mg tablet[/url]
[url=https://rybelsus.download/]rybelsus canada[/url]
[url=https://ozempic.company/]buy rybelsus[/url]
Доброго!
Меня зовут Вика)
Учусь в универе на 4 курсе, время дипломных подготовок, курсовых и рефератов, но Я девушка и у меня другие интересы….
Пришло время расплаты, и меня чуть не отчислили, хорошо подруга сказала что оказывается можно купить диплом или курсовую, конечно Вы не получите твердую 5, но 4 или 3 точно, пройдете дальше по учебе что и необходимо)
Вот сайт компании, дипломы на заказ для любых вузов под ключ: http://dodip.ru/
[url=http://dodip.ru/]купить диплом в гатчине[/url]
[url=http://dodip.ru/]купить диплом[/url]
[url=http://dodip.ru/]купить аттестат школы[/url]
Кстати у конкурентов сразу просили предоплату, каике-то там условия это пугает, тут ничего такого нет, все после результата, спасибо им большое!
Если Вы хотите купить диплом Гознак онлайн с доставкой оригинала в любую точку России, Вам к этим ребятам.
Удачи и хороших оценок!
купить диплом в подольске
купить диплом в серове
купить диплом колледжа
купить диплом в невинномысске
где купить диплом
купить диплом в нефтекамске
купить диплом в крыму
купить диплом в керчи
Здравствуйте!
Меня зовут Вика)
Учусь в универе на 4 курсе, время дипломных подготовок, курсовых и рефератов, но Я девушка и у меня другие интересы….
Пришло время расплаты, и меня чуть не отчислили, хорошо подруга сказала что оказывается можно купить диплом или курсовую, конечно Вы не получите твердую 5, но 4 или 3 точно, пройдете дальше по учебе что и необходимо)
Вот сайт компании, дипломы на заказ для любых вузов под ключ: http://dodip.ru/
[url=http://dodip.ru/]купить диплом в новоуральске[/url]
[url=http://dodip.ru/]купить диплом охранника[/url]
[url=http://dodip.ru/]купить диплом матроса[/url]
Кстати у конкурентов сразу просили предоплату, каике-то там условия это пугает, тут ничего такого нет, все после результата, спасибо им большое!
Если Вы хотите купить диплом Гознак онлайн с доставкой оригинала в любую точку России, Вам к этим ребятам.
Удачи и хороших оценок!
купить диплом в пскове
купить диплом физика
купить диплом в кирове
купить диплом в петропавловске-камчатском
купить диплом в ишиме
купить диплом сантехника
купить диплом техника
купить диплом в челябинске
[url=http://semaglutiderybelsus.online/]where to buy semaglutide[/url]
[url=http://semaglutidetabs.online/]semaglutide medication[/url]
Привет всем!
Были ли у вас случаи, когда вам приходилось писать дипломную работу в сжатые сроки? Это действительно трудно и ответственно, но важно не сдаваться и продолжать работать над учебными процессами, чем я и занимаюсь.
Для тех, кто умеет грамотно пользоваться интернетом и находить нужную информацию, это действительно оказывается полезным. Это помогает в процессе согласования и написания дипломной работы, и нет необходимости тратить время на посещение библиотек или встречи с дипломным руководителем. Если вам нужны хорошие источники для подготовки дипломных и курсовых работ, я могу поделиться полезными ссылками.
http://vuzdiploma.ru/
Желаю всем отличных оценок!
купить диплом сантехника
купить диплом образцы
купить диплом мастера маникюра и педикюра
купить диплом цена
купить диплом в кирове
купить диплом в санкт-петербурге
купить диплом в череповце
купить бланк диплома
купить диплом массажиста
купить аттестат за 9 классов
Здравствуйте!
Диплом – это кошмар. Срыв сроков, здоровье пошло на убыль, но интернет помогает найти методы ускорения работы. Не сдаюсь.
http://www.52ru.ru/showthread.php?p=37402#post37402
http://best-broker.name/forum/34——4–mt5/29748—.html#29748
Желаю всем отличных оценок!
куплю диплом
купить диплом в калуге
купить диплом оценщика
купить диплом в воронеже
купить диплом в тамбове
купить свидетельство о рождении ссср
купить диплом с реестром
купить диплом в пятигорске
куплю диплом о высшем образовании
купить диплом продавца
[url=http://ozempic.us.org/]rybelsus semaglutide[/url]
🚀 Wow, blog ini seperti petualangan fantastis melayang ke galaksi dari kegembiraan! 💫 Konten yang menarik di sini adalah perjalanan rollercoaster yang mendebarkan bagi imajinasi, memicu kagum setiap saat. 🎢 Baik itu teknologi, blog ini adalah harta karun wawasan yang mendebarkan! #TerpukauPikiran Berangkat ke dalam petualangan mendebarkan ini dari imajinasi dan biarkan imajinasi Anda terbang! 🌈 Jangan hanya mengeksplorasi, alami kegembiraan ini! #MelampauiBiasa Pikiran Anda akan bersyukur untuk perjalanan mendebarkan ini melalui alam keajaiban yang menakjubkan! 🌍
Hello everyone!
I recommend visiting these resources, there is a lot of useful structured information, for any questions and just to take a break from all the work:
http://molbiol.ru/forums/index.php?showtopic=1061138
https://forum.bocu.ro/viewtopic.php?p=80599#80599
Good luck! If you know similar ones, be sure to let us know)
Hello everyone!
I suggest visiting these resources for a wealth of valuable, well-organized information, whether you have questions or simply need a break from your workload:
https://batcheres.bcbloggers.com/24548450/discover-the-uniqueness-of-stellar-spins-casino
https://nowewyrazy.uw.edu.pl/profil/pokiesnet07
Good luck! If you know similar ones, be sure to let us know)
Hello everyone!
I recommend visiting these resources, there is a lot of useful structured information, for any questions and just to take a break from all the work:
http://bereg.webtalk.ru/viewtopic.php?id=10178#p30372
https://directory.thenorthernecho.co.uk/company/59b7a71abda1a7464ea84fb77114bb76
Good luck! If you know similar ones, be sure to let us know)
Hello everyone!
I recommend visiting these resources, there is a lot of useful structured information, for any questions and just to take a break from all the work:
http://www.dalby.com.au/new-south-wales/bateau-bay/arts-entertainment-and-leisure/national-casino
http://www.artisteer.com/Default.aspx?post_id=267508&p=forum_post&forum_id=9
Good luck! If you know similar ones, be sure to let us know)
Hello everyone!
I suggest checking out these resources, where you’ll find plenty of helpful, well-organized information, whether you have questions or just need a break from your work:
https://hypothes.is/users/reelsroyal
https://www.pampling.com/comunidad/blog-de-usuarios/151120-rozrywki
Good luck! If you know similar ones, be sure to let us know)
Hello everybody!
Have you ever heard of X-GPT Writer: a unique keyword content generator based on the ChatGPT neural network?
I also haven’t, until I was advised to automate routine tasks with this software, I want to say one thing! For a long time afterwards, I couldn’t believe
that ChatGPT was such a powerful product if it was used simultaneously in streaming, running X-GPT Writer.
I thought it was just a utility, it was inexpensive, a friend gave me a coupon for a 40% discount%:
94EB516BCF484B27
the details of where to enter it are indicated on the website:
https://www.xtranslator.ru/x-gpt-writer/
I started trying, delving into it, bought 50 ChatGPT accounts at low prices and off I went!
Now I easily generate and launch 3-4 new sites per week, batch unify entire folders and even create images
using the ChatGPT neural network and X-GPT Writer.
It’s worth a try, Friends, there’s a demo, everything is free, you won’t regret it)
Good luck!
ChatGPT и X-GPTWriter в качестве инструментов синонимизации
X-GPTWriter: купоны и акции на скидки
X-GPTWriter купить со скидкой
Генератор синонимов с ChatGPT
ChatGPT как инструмент для генерации контента
программа создания контента через ChatGPT
Где найти промо коды на X-GPTWriter
Как сделать текст более уникальным с ChatGPT
X-GPTWriter: мощный инструмент для контент-креации
ChatGPT и X-GPTWriter: новая эра контент-маркетинга
Здравствуйте!
Были ли у вас случаи, когда вам приходилось писать дипломную работу в сжатые сроки? Это действительно трудно и ответственно, но важно не сдаваться и продолжать работать над учебными процессами, чем я и занимаюсь.
Для тех, кто умеет грамотно пользоваться интернетом и находить нужную информацию, это действительно оказывается полезным. Это помогает в процессе согласования и написания дипломной работы, и нет необходимости тратить время на посещение библиотек или встречи с дипломным руководителем. Если вам нужны хорошие источники для подготовки дипломных и курсовых работ, я могу поделиться полезными ссылками.
http://vuzdiploma.ru/
Желаю всем отличных оценок!
купить диплом в новом уренгое
купить диплом в новороссийске
купить диплом в белогорске
купить диплом монтажника
купить диплом в ялте
купить диплом в петрозаводске
купить диплом в челябинске
купить диплом в иваново
купить диплом в северске
купить диплом эколога
Привет всем!
Я начал делать сетку сайтов, все было не в поиске, но прогон Bullet помог
У нас была жесткая тематика, но наш сайт пошел выше после работы парней с Bullet
https://www.google.com/search?q=%D0%BF%D1%80%D0%BE%D0%B3%D0%BE%D0%BD+%D0%B4%D0%BE%D1%80%D0%BE%D0%B2+bullet+kwork&sca_esv=46579d9512b829aa&ei=_hHSZaflIZKBxc8Psd2i6AY&udm=&ved=0ahUKEwin3MnYirWEAxWSQPEDHbGuCG0Q4dUDCBA&uact=5&oq=%D0%BF%D1%80%D0%BE%D0%B3%D0%BE%D0%BD+%D0%B4%D0%BE%D1%80%D0%BE%D0%B2+bullet+kwork&gs_lp=Egxnd3Mtd2l6LXNlcnAiJNC_0YDQvtCz0L7QvSDQtNC-0YDQvtCyIGJ1bGxldCBrd29yazIJECEYChigARgqSKYVUJ4EWO8ScAN4AJABAZgBrQKgAccNqgEFMi02LjG4AQPIAQD4AQHCAgcQIRgKGKABiAYB&sclient=gws-wiz-serp
https://www.google.com/search?q=%D0%BF%D1%80%D0%BE%D0%B3%D0%BE%D0%BD+%D1%81%D0%B0%D0%B9%D1%82%D0%B0+bullet&sca_esv=46579d9512b829aa&ei=PAjSZb-wLdSP1fIPxeKh6AM&udm=&ved=0ahUKEwi_vsOxgbWEAxXUR1UIHUVxCD0Q4dUDCBA&uact=5&oq=%D0%BF%D1%80%D0%BE%D0%B3%D0%BE%D0%BD+%D1%81%D0%B0%D0%B9%D1%82%D0%B0+bullet&gs_lp=Egxnd3Mtd2l6LXNlcnAiHtC_0YDQvtCz0L7QvSDRgdCw0LnRgtCwIGJ1bGxldEj_GlC0BljhGHAGeAGQAQKYAYYCoAG9EaoBBTAuMi44uAEDyAEA-AEBwgIKEAAYRxjWBBiwA8ICCBAhGKABGMMEwgIMECEYChigARjDBBgqwgIKECEYChigARjDBIgGAZAGCA&sclient=gws-wiz-serp
Удачи!
Привет всем!
Мой сайт стоял на месте, но данный продукт смог его раскачать
Я начал делать сетку сайтов, все было не в поиске, но прогон Bullet помог
https://www.google.com/search?q=%D0%BF%D1%80%D0%BE%D0%B4%D0%B2%D0%B8%D0%B6%D0%B5%D0%BD%D0%B8%D0%B5+%D1%81%D0%B0%D0%B9%D1%82%D0%B0+%D0%B2+google+%D1%82%D0%B0%D1%80%D0%B8%D1%84+bullet+kwork&sca_esv=46579d9512b829aa&ei=LxzSZZCSGrygwPAP8fGNmAQ&udm=&ved=0ahUKEwjQ5cq0lLWEAxU8EBAIHfF4A0MQ4dUDCBA&uact=5&oq=%D0%BF%D1%80%D0%BE%D0%B4%D0%B2%D0%B8%D0%B6%D0%B5%D0%BD%D0%B8%D0%B5+%D1%81%D0%B0%D0%B9%D1%82%D0%B0+%D0%B2+google+%D1%82%D0%B0%D1%80%D0%B8%D1%84+bullet+kwork&gs_lp=Egxnd3Mtd2l6LXNlcnAiQ9C_0YDQvtC00LLQuNC20LXQvdC40LUg0YHQsNC50YLQsCDQsiBnb29nbGUg0YLQsNGA0LjRhCBidWxsZXQga3dvcmsyCBAhGKABGMMESPVMUOYcWJ5FcAZ4AZABA5gB8AGgAY8UqgEGMC4xMC40uAEDyAEA-AEBwgIKEAAYRxjWBBiwA8ICCBAAGIkFGKIEwgIMECEYChigARjDBBgqiAYBkAYI&sclient=gws-wiz-serp
https://www.google.com/search?q=%D0%BF%D1%80%D0%BE%D0%B3%D0%BE%D0%BD+%D0%B4%D0%BE%D1%80%D0%BE%D0%B2+bullet+kwork&sca_esv=46579d9512b829aa&ei=_hHSZaflIZKBxc8Psd2i6AY&udm=&ved=0ahUKEwin3MnYirWEAxWSQPEDHbGuCG0Q4dUDCBA&uact=5&oq=%D0%BF%D1%80%D0%BE%D0%B3%D0%BE%D0%BD+%D0%B4%D0%BE%D1%80%D0%BE%D0%B2+bullet+kwork&gs_lp=Egxnd3Mtd2l6LXNlcnAiJNC_0YDQvtCz0L7QvSDQtNC-0YDQvtCyIGJ1bGxldCBrd29yazIJECEYChigARgqSKYVUJ4EWO8ScAN4AJABAZgBrQKgAccNqgEFMi02LjG4AQPIAQD4AQHCAgcQIRgKGKABiAYB&sclient=gws-wiz-serp
Удачи!
[url=https://semaglutide.download/]rybelsus canada pharmacy prices[/url]
nice content!nice history!! boba 😀
wow, amazing
nice content!nice history!! boba 😀
wow, amazing
nice content!nice history!! boba 😀
nice content!nice history!! boba 😀
nice content!nice history!! boba 😀
nice content!nice history!! boba 😀
nice content!nice history!! boba 😀
Super, fantastic
Great job
Excellent effort
Impressive, fantastic
Marvelous, impressive
wow, amazing
nice content!nice history!! boba 😀
Thank you, I’ve recently been looking for information about this topic for ages and yours is the greatest I have discovered till now.
However, what about the bottom line? Are
you sure concerning the supply?
wow, amazing
nice content!nice history!! boba 😀
nice content!nice history!! boba 😀
wow, amazing
Excellent write-up
wow, amazing
nice content!nice history!! boba 😀
wow, amazing
nice content!nice history!! boba 😀
[url=https://prednisoneo.com/]prednisone buy cheap[/url]
[url=https://happyfamilymedicalstore.online/]escrow pharmacy canada[/url]
[url=http://oazithromycin.online/]where can i get zithromax[/url]
phising
Уютный уголок для семьи
6. Стильный дом из бруса 9х12: современный дизайн
дома из бруса 9х12 [url=https://domizbrusa9x12spb.ru/]https://domizbrusa9x12spb.ru/[/url] .
I strongly recommend to avoid this site. My personal experience with it was only frustration as well as suspicion of deceptive behavior. Proceed with extreme caution, or alternatively, look for a more reputable site to meet your needs.
I strongly recommend to avoid this site. My personal experience with it was purely frustration as well as doubts about fraudulent activities. Be extremely cautious, or alternatively, look for an honest site to meet your needs.
I urge you steer clear of this site. The experience I had with it has been only disappointment and suspicion of scamming practices. Be extremely cautious, or alternatively, look for a trustworthy platform to fulfill your requirements.
I urge you stay away from this platform. The experience I had with it was nothing but frustration along with concerns regarding deceptive behavior. Proceed with extreme caution, or better yet, look for an honest site for your needs.
I strongly recommend stay away from this platform. The experience I had with it has been nothing but frustration along with doubts about deceptive behavior. Exercise extreme caution, or alternatively, find a trustworthy service for your needs.
[url=http://happyfamilystorerx.online/]best european online pharmacy[/url]
[url=https://happyfamilymedicalstore.online/]legal online pharmacies in the us[/url]
[url=https://ezithromycin.online/]zithromax 500mg[/url]
[url=https://oprednisone.online/]prednisone over the counter[/url]
[url=https://oprednisone.online/]prednisone 10mg buy online[/url]
[url=http://azithromycinps.online/]azithromycin 500mg buy[/url]
[url=https://valtrexid.com/]generic for valtrex buy without a prescription[/url]
[url=https://azithromycinps.online/]azithromycin cost india[/url]
[url=http://valtrexmedication.com/]generic valtrex 1000mg for sale[/url]
[url=http://oazithromycin.com/]azithromycin 600 mg tablet[/url]
[url=https://predniso.online/]200 mg prednisone[/url]
[url=http://azithromycinmds.com/]azithromycin 500 mg tablet price in india[/url]
[url=http://metformin.store/]buy metformin online singapore[/url]
[url=https://prednisonekx.online/]buy prednisone online canada without prescription[/url]
[url=http://valtrexarb.online/]valtrex medicine price[/url]