Python is one of the most popular and versatile programming languages in the world. It can be used for web development, data analysis, machine learning, game development, and more. But how does Python work? How can you use it to create amazing applications? In this blog post, we will explore some of the basic concepts of Python, such as pips and modules, and how they can help you write better code.
Table of Contents
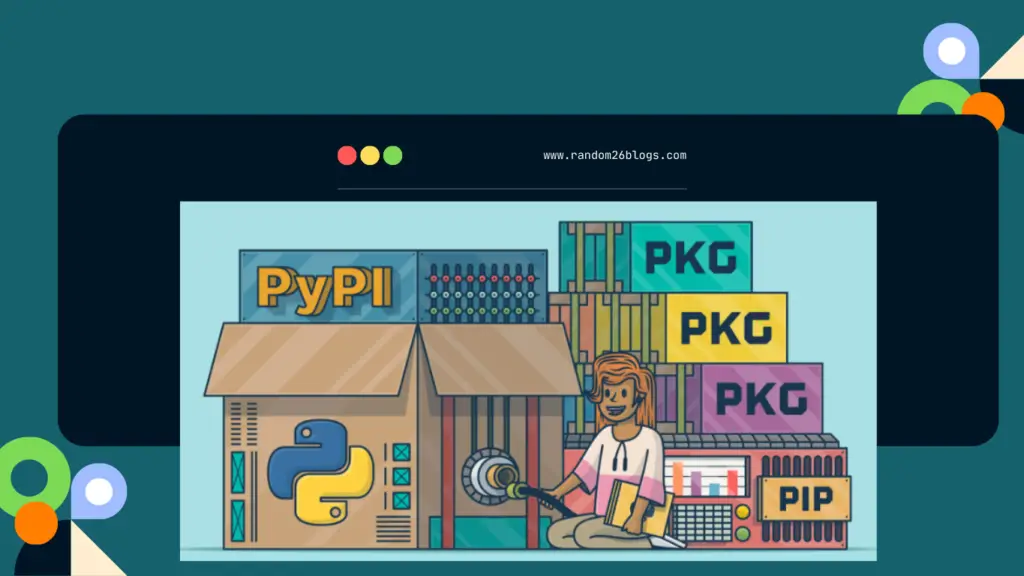
What is Python?
Python is an interpreted, high-level, general-purpose programming language. It was created by Guido van Rossum in 1991 and named after the comedy show Monty Python’s Flying Circus. Python has a simple and elegant syntax that makes it easy to read and write. It also supports multiple programming paradigms, such as object-oriented, procedural, functional, and imperative. Python has a large and comprehensive standard library that provides built-in modules for common tasks, such as file handling, networking, math, and more.
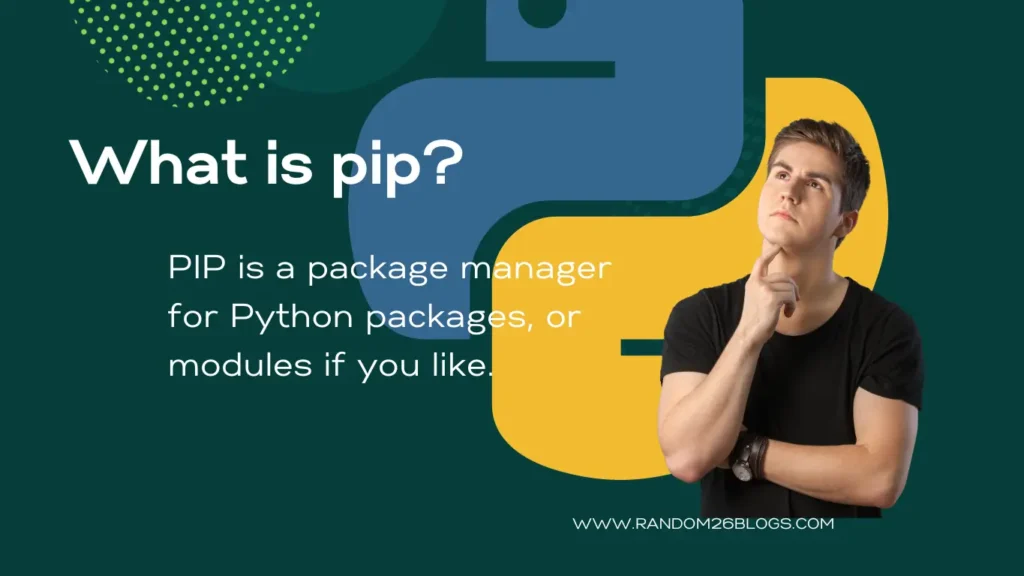
What are Pips?
Well, pips are also the name of a tool that helps you install and manage additional packages or libraries for Python. Pips stands for Pip Installs Packages or Pip Installs Python. Pips are not part of the standard library, but they are widely used by Python developers to extend the functionality of their programs.
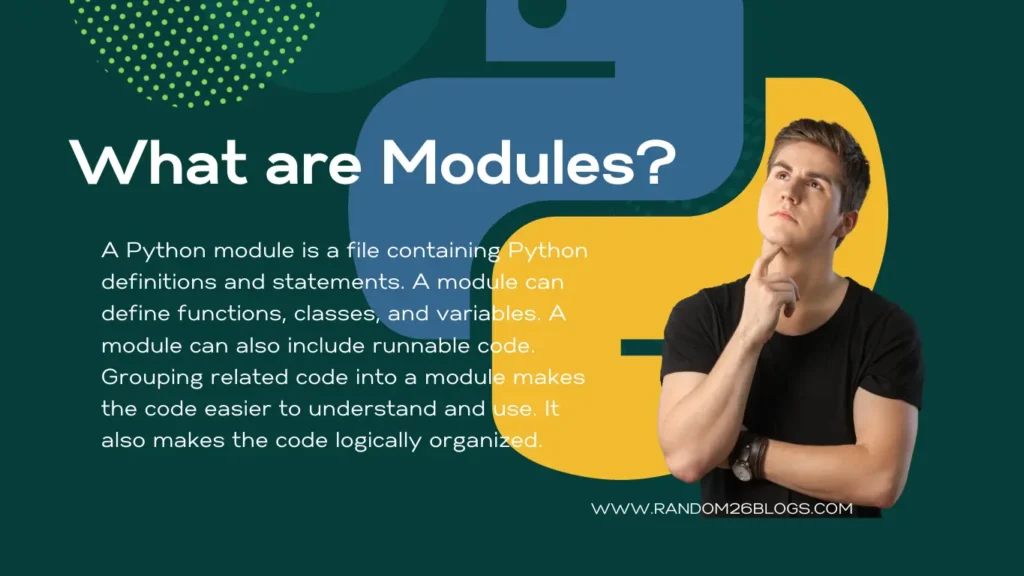
What are Modules?
Modules are files that contain Python code. They can define functions, classes, variables, and other objects that can be imported and used by other modules or scripts. For example, if you have a file called hello.py that contains the following code:
def say_hello(name):
print(f"Hello, {name}!")
You can import this module in another file or in the interactive interpreter and use the say_hello function:
import hello
hello.say_hello("Alice")
# Output: Hello, Alice!
Modules are useful because they allow you to organize your code into reusable and maintainable units. You can also use modules to avoid name conflicts between different variables or functions that have the same name.
How to Use Pips to Install Modules?
One of the advantages of using pips is that you can easily install modules from online repositories, such as PyPI (Python Package Index). PyPI is a website that hosts thousands of open-source projects for Python. You can find modules for almost any purpose, such as web development, data analysis, machine learning, game development, and more.
To use pips to install modules from PyPI, you need to have pips installed on your computer. You can check if you have pips by typing pip --version
in your terminal or command prompt. If you don’t have pips, you can follow the instructions on how to install it from [here].
Once you have pips installed, you can use the following command to install any module from PyPI:
pip install module_name
For example, if you want to install a module called requests that allows you to make HTTP requests in Python, you can type:
pip install requests
This will download and install the requests module and its dependencies on your computer. You can then import and use it in your Python code:
import requests
response = requests.get("https://www.bing.com")
print(response.status_code)
# Output: 200
How to Use Modules in Your Python Code?
Once you have installed a module using pips or any other method, you can use it in your Python code by importing it. There are different ways to import a module in Python:
- You can import the whole module by using
import module_name
. This will make all the objects defined in the module available under the module name. For example:
import math
print(math.pi)
# Output: 3.141592653589793
- You can import specific objects from a module by using
from module_name import object_name
. This will make only those objects available without the module name. For example:
from math import pi
print(pi)
# Output: 3.141592653589793
- You can import all objects from a module by using
from module_name import *
. This will make all the objects available without the module name. However, this is not recommended, as it can cause name conflicts and make your code less readable. For example:
from math import *
print(pi)
# Output: 3.141592653589793
- You can rename a module or an object by using
import module_name as new_name
orfrom module_name import object_name as new_name
. This can help you avoid name conflicts or make your code more concise. For example:
import math as m
print(m.pi)
# Output: 3.141592653589793
How to Create Your Own Modules?
You can also create your own modules in Python by writing your own code in a file with a .py extension. For example, if you have a file called my_module.py that contains the following code:
def add(a, b):
return a + b
def subtract(a, b):
return a - b
You can import and use this module in another file or in the interactive interpreter:
import my_module
print(my_module.add(2, 3))
# Output: 5
print(my_module.subtract(5, 2))
# Output: 3
You can also use the if __name__ == "__main__"
statement to execute some code only when the module is run as a script, and not when it is imported by another module. For example, if you modify the my_module.py file as follows:
def add(a, b):
return a + b
def subtract(a, b):
return a - b
if __name__ == "__main__":
print("This is my module")
print(add(2, 3))
print(subtract(5, 2))
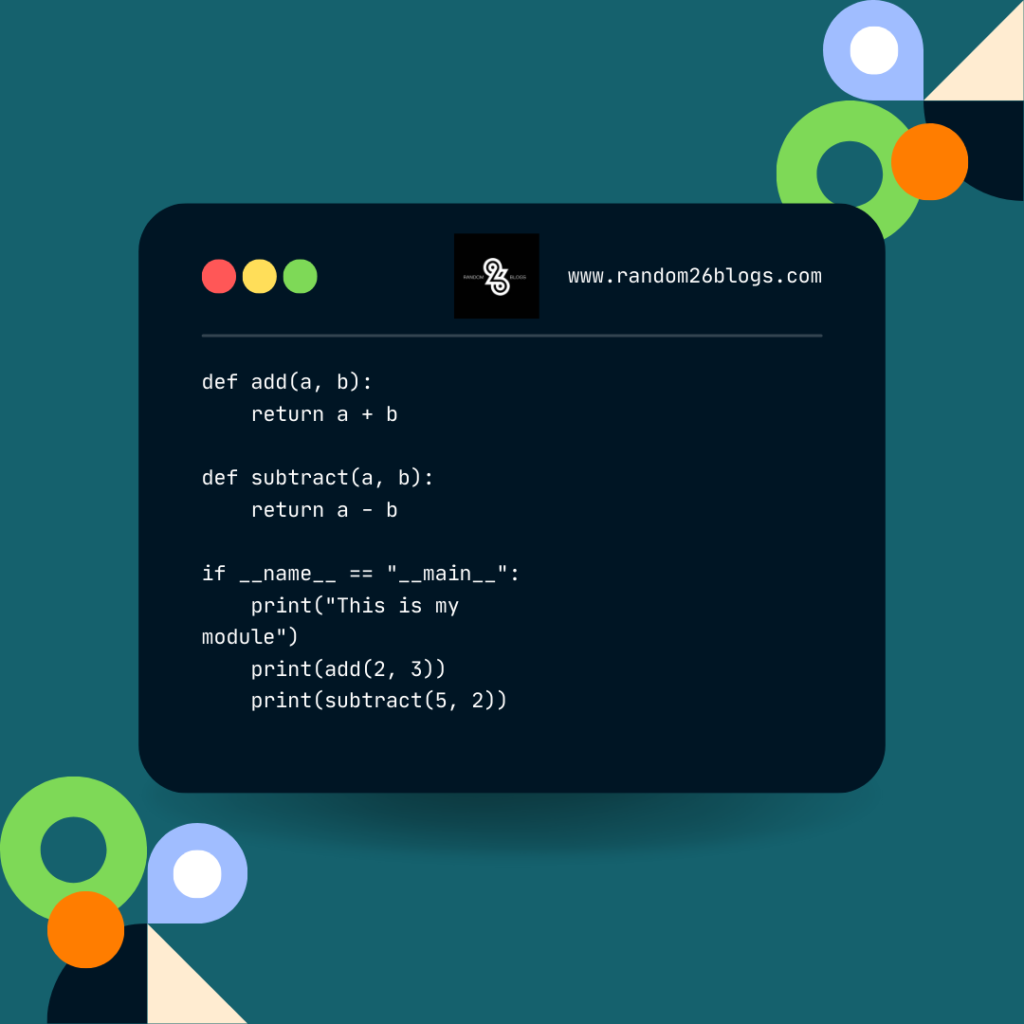
Then, if you run this file as a script, you will see the following output:
python my_module.py
# Output:
This is my module
5
3
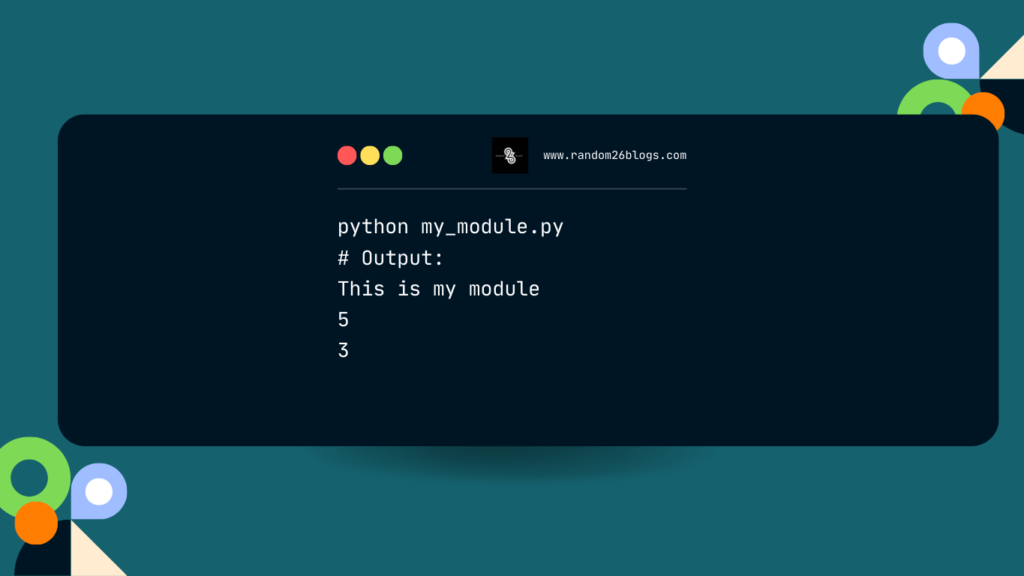
But if you import this module in another file or in the interactive interpreter, you will not see the output:
import my_module
# No output
Key point
In this blog post, we have learned some of the basic concepts of Python, such as pips and modules, and how they can help you write better code. We have seen how to use pips to install modules from online repositories, such as PyPI, and how to import and use modules in our Python code. We have also seen how to create our own modules and execute some code only when the module is run as a script.
We hope that this blog post has been helpful and informative for you. If you have any questions or feedback, please feel free to leave a comment below. Happy coding!
First of all I would like to say great blog! I had a quick question which I’d like to ask if you do not mind. I was interested to know how you center yourself and clear your thoughts before writing. I’ve had a difficult time clearing my thoughts in getting my ideas out there. I do enjoy writing however it just seems like the first 10 to 15 minutes are wasted just trying to figure out how to begin. Any suggestions or tips? Many thanks!
[url=https://avana.cfd/]buy dapoxetine cheap[/url]
[url=https://albuterol.cyou/]albuterol uk[/url]
[url=https://baclofen.cfd/]baclofen 10mg tablet[/url]
[url=http://propranolol.cyou/]propranolol order online[/url]
[url=https://synthroid.cyou/]synthroid 25 mg price[/url]
[url=https://finasteride.cyou/]propecia without prescription[/url]
[url=http://amoxicillin.cyou/]amoxicillin drug[/url]
[url=https://retina.cfd/]buy retin a over the counter[/url]
[url=https://albuterol.cfd/]ventolin 50 mg[/url]
[url=http://accutane.cyou/]can you buy accutane over the counter[/url]
[url=https://sildenafil.cyou/]female viagra south africa[/url]
[url=https://doxycycline.guru/]doxycycline hyclate capsules[/url]
[url=http://zithromax.cyou/]buy azithromycin online fast shipping[/url]
[url=http://prednisolone.directory/]can i buy prednisolone over the counter in uk[/url]
[url=https://levitra.cfd/]vardenafil price comparison[/url]
[url=http://tadalafil.cyou/]cialis price comparison[/url]
[url=https://clonidine.cyou/]clonidine buy uk[/url]
[url=https://amoxicillin.boutique/]amoxicillin 325[/url]
[url=http://xenical.cfd/]orlistat online pharmacy[/url]
[url=https://azithromycin.digital/]buy azithromycin usa[/url]
[url=http://fluoxetine.cyou/]fluoxetine 60 mg brand name[/url]
[url=http://furosemide.cyou/]furosemide canada[/url]
[url=https://lisinopril.cfd/]lisinopril 80 mg daily[/url]
[url=http://clonidine.cfd/]clonidine 0.2 mg tablets[/url]
[url=https://trazodone.best/]trazadone[/url]
[url=https://vardenafil.cfd/]buy levitra australia[/url]
[url=http://propecia.cyou/]cheap propecia[/url]
[url=https://albuterol.cfd/]ventolin usa over the counter[/url]
[url=http://clonidine.cyou/]buy clonidine australia[/url]
[url=https://wellbutrin.cfd/]wellbutrin brand cost[/url]
[url=https://robaxin.cyou/]robaxin v[/url]
[url=https://tadalafil.cyou/]can i order cialis online[/url]
[url=https://suhagra.cyou/]suhagra 100 mg[/url]
[url=http://accutane.cyou/]accutane online[/url]
[url=http://augmentin.cfd/]augmentin 875 mg 125 mg tablet[/url]
[url=https://diflucan.cyou/]diflucan 150 price[/url]
[url=https://finasteride.best/]buy generic propecia online canada[/url]
[url=https://retina.directory/]tretinoin 20 mg[/url]
[url=http://ivermectin.guru/]ivermectin cream uk[/url]
[url=https://propecia.cyou/]buy propecia australia[/url]
[url=http://lyrica.cfd/]buy lyrica from canada[/url]
[url=https://albuterol.guru/]ventolin proventil[/url]
[url=http://paxil.cfd/]paroxetine 12.5 price[/url]
[url=http://doxycycline.cfd/]doxycycline 40 mg cost[/url]
[url=https://augmentin.cfd/]amoxil 500mg capsules[/url]
[url=https://azithromycin.digital/]zithromax online pharmacy[/url]
[url=https://doxycycline.directory/]doxycycline order online canada[/url]
[url=http://trazodone.cfd/]trazodone online no prescription[/url]
[url=http://sildenafil.cfd/]buy viagra online in south africa[/url]
[url=http://finasteride.cyou/]propecia online pharmacy usa[/url]
[url=http://augmentin.guru/]augmentin 625[/url]
[url=http://sildenafil.cyou/]cheap canadian viagra online[/url]
[url=https://sildenafil.cyou/]viagra price india[/url]
[url=https://wellbutrin.cfd/]wellbutrin generic price[/url]
[url=https://lisinopril.cfd/]lisinopril 2mg tablet[/url]
[url=http://lexapro.cfd/]lexapro cheap price[/url]
tsmavic.wordpress.com – приворот женщины по фото
отворот сына от невестки, приворот в домашних условиях кто делал отзывы
http://www.magecam.ru – бесплатный приворот мужчины
[url=https://bactrim.cyou/]can you buy bactrim over the counter[/url]
[url=https://hydroxychloroquine.guru/]plaquenil medication[/url]
Hello everybody!
Have you ever heard of X-GPT Writer: a unique keyword content generator based on the ChatGPT neural network?
I also haven’t, until I was advised to automate routine tasks with this software, I want to say one thing! For a long time afterwards, I couldn’t believe
that ChatGPT was such a powerful product if it was used simultaneously in streaming, running X-GPT Writer.
I thought it was just a utility, it was inexpensive, a friend gave me a coupon for a 40% discount%:
94EB516BCF484B27
the details of where to enter it are indicated on the website:
https://www.xtranslator.ru/x-gpt-writer/
I started trying, delving into it, bought 50 ChatGPT accounts at low prices and off I went!
Now I easily generate and launch 3-4 new sites per week, batch unify entire folders and even create images
using the ChatGPT neural network and X-GPT Writer.
It’s worth a try, Friends, there’s a demo, everything is free, you won’t regret it)
Good luck!
ChatGPT и X-GPTWriter для уникальных текстов
ChatGPT как инструмент для генерации контента
Где найти промо коды на X-GPTWriter
Создание контента с помощью X-GPTWriter
Синонимизация текста с X-GPTWriter и ChatGPT
Автоматизированное создание текстов с помощью ChatGPT
X-GPTWriter: мощный инструмент для контент-креации
Создание оригинальных текстов с помощью синонимайзера на базе ChatGPT
автоматическое создание текстов через ChatGPT
Создание уникальных статей с ChatGPT
[url=https://lisinopril.cfd/]cost for 20 mg lisinopril[/url]
[url=http://baclofen.cfd/]baclofen[/url]
[url=https://lisinopril.cfd/]lisinopril tabs[/url]
[url=https://levitra.cfd/]buy levitra generic online[/url]
[url=https://vermox.cyou/]vermox canada otc[/url]
ритуал черного приворота – Обратится к магу – dzen.ru/id/653538d7d6100f7a6fee8469
приворот черная магия на вещи
[url=https://neurontin.cfd/]neurontin 400 mg capsules[/url]
[url=https://lyrica.cfd/]lyrica 125 mg[/url]
Hello
I want to tell you about my experience, how games have turned into real income and help me live!
One day a friend told me about a rather simple but exciting game, thanks to which, after training on a demo account, you can put funds for real bets.
At first I was skeptical about this, but now I have a different opinion!
Lucky jet 1Win – A simple and entertaining game, the guy with the satchel must fly as high as possible and achieve his goals, and these are your bets.
It’s corny, but after a detailed study, I started to win a little and found my stable style of play, the official website has both statistical calculations and classic strategies and hacks!
By the way, anyone who played the legendary Aviator will be delighted with Lucky jet 1Win,there is still the possibility of increased profits!
All information about the gameLucky jet,on the official multilingual website:
English – https://lucky-jetcash.com/en/
Spanish – https://lucky-jetcash.com/es/
Azerbaijanian – https://lucky-jetcash.com/az/
Dutch – https://lucky-jetcash.com/de/
French – https://lucky-jetcash.com/fr/
Portuguese – https://lucky-jetcash.com/pt/
Turkish – https://lucky-jetcash.com/tr/
Kazakh – https://lucky-jetcash.com/kk/
Rus – https://lucky-jetcash.com/
I wish you positivity and winnings in the coming 2024!
See you in the communityLucky jet 1Win!
lucky jet sinais
1win lucky jet
lucky jet crash game
lucky jet reviews
[url=https://lucky-jetcash.com]lucky jet statistic[/url]
[url=https://lucky-jetcash.com]lucky jet official site[/url]
[url=https://lucky-jetcash.com]lucky jet crash game[/url]
[url=https://lucky-jetcash.com]lucky jet signals[/url]
[url=http://hydroxychloroquine.guru/]plaquenil 300 mg[/url]
[url=https://wellbutrin.cfd/]zyban canada[/url]
Hi everyone
I want to tell you about my experience, how games have turned into real income and help me live!
One day a friend told me about a rather simple but exciting game, thanks to which, after training on a demo account, you can put funds for real bets.
At first I was skeptical about this, but now I have a different opinion!
Lucky jet 1Win – A simple and entertaining game, the guy with the satchel must fly as high as possible and achieve his goals, and these are your bets.
It’s corny, but after a detailed study, I started to win a little and found my stable style of play, the official website has both statistical calculations and classic strategies and hacks!
By the way, who played the legendary Aviator will be delighted with Lucky jet 1Win,there is still the possibility of increased profits!
All information about the gameLucky jet,on the official multilingual website:
English – https://lucky-jetcash.com/en/
Spanish – https://lucky-jetcash.com/es/
Azerbaijanian – https://lucky-jetcash.com/az/
Dutch – https://lucky-jetcash.com/de/
French – https://lucky-jetcash.com/fr/
Portuguese – https://lucky-jetcash.com/pt/
Turkish – https://lucky-jetcash.com/tr/
Kazakh – https://lucky-jetcash.com/kk/
Rus – https://lucky-jetcash.com/
I wish you positivity and winnings in the coming 2024!
See you in the communityLucky jet 1Win!
1 win lucky jet
lucky jet 1win
lucky jet играть
lucky jet signals
[url=https://lucky-jetcash.com]lucky jet offers[/url]
[url=https://lucky-jetcash.com]lucky jet reviews[/url]
[url=https://lucky-jetcash.com]lucky jet 1 win[/url]
[url=https://lucky-jetcash.com]lucky jet hack[/url]
[url=http://neurontin.cfd/]gabapentin online uk[/url]
[url=https://albuterol.cyou/]albuterol generic otc[/url]
[url=http://budesonide.cyou/]budesonide 64 mcg[/url]
[url=https://robaxin.cyou/]robaxin 750 cost[/url]
[url=http://onlinepharmacy.cyou/]overseas pharmacy no prescription[/url]
Hello everybody!
How do you feel about computer graphics and design?
Once I started looking at the works of leading designers and it became my hobby, computer graphics allows you to do
beautiful works, design solutions and advertising creatives.
By the way, I noticed that it broadens my horizons, and brings peace to my life!
I recommend profiles of these masters:
https://dprofile.ru/sh_alsina/collection/3728/aidentika
https://dprofile.ru/case/35919/function%20()%20%20%5Bnative%20code%5D%20
https://dprofile.ru/shev/story/3
https://dprofile.ru/case/49060/function%20()%20%20%5Bnative%20code%5D%20
https://dprofile.ru/lunarnia/story/3
https://dprofile.ru/sasheeme/cv
https://dprofile.ru/cases/search?City=1&hasAchievement=all&sort=date&city=984
https://dprofile.ru/yous/collection/4672/3d
https://dprofile.ru/case/33326/function%20()%20%20%5Bnative%20code%5D%20
https://dprofile.ru/anya_dobrota/collection/4443/followers
https://dprofile.ru/users/search?type=personal&sort=followers&filter=3&Filter=107
https://dprofile.ru/users/search?type=personal&sort=followers&Filter=7&city=831
https://dprofile.ru/vetcogray/cv
https://dprofile.ru/case/37490/function%20()%20%20%5Bnative%20code%5D%20
https://dprofile.ru/m00nshadow88/cv
https://dprofile.ru/kurikota/story/3
https://dprofile.ru/case/6094/function%20()%20%20%5Bnative%20code%5D%20
https://dprofile.ru/vostro/cv
https://dprofile.ru/krisplazun/cv
https://dprofile.ru/vicious/story/3
Good luck!
[url=http://vardenafil.directory/]price of levitra 10 mg[/url]
[url=http://bactrim.cyou/]bactrim pill[/url]
[url=http://onlinepharmacy.cyou/]canada pharmacy world[/url]
[url=https://prozac.cfd/]best price for generic prozac[/url]
[url=http://paxil.cfd/]buy paroxetine[/url]
[url=http://fluconazole.cyou/]diflucan usa[/url]
[url=http://fluoxetine.cyou/]fluoxetine 20 mg australia[/url]
[url=http://sildenafil.cfd/]prices for viagra prescription[/url]
[url=https://allopurinol.cfd/]allopurinol 100[/url]
Hello everybody!
How do you feel about computer graphics and design?
Once I started looking at the works of leading designers and it became my hobby, computer graphics allows you to do
beautiful works, design solutions and advertising creatives.
By the way, I noticed that it broadens my horizons, and brings peace to my life!
I recommend profiles of these masters:
https://dprofile.ru/sofya0829/info
https://dprofile.ru/kiselyuxa/info
https://dprofile.ru/n.kungurova.1995/following
https://dprofile.ru/mariinasolopova
https://dprofile.ru/dariamadai
https://dprofile.ru/petrovalz/story/2
https://dprofile.ru/egorov.d.nikita/story/2
https://dprofile.ru/anastasiaden/info
https://dprofile.ru/regina91/story/2
https://dprofile.ru/marta/followers
https://dprofile.ru/dinapetova/following
https://dprofile.ru/cldlne/info
https://dprofile.ru/anastasiya_derevnina/story/2
https://dprofile.ru/maksim_888/followers
https://dprofile.ru/iammkhv/followers
https://dprofile.ru/carrot_witch
https://dprofile.ru/ivmwtd/info
https://dprofile.ru/marinaus/followers
https://dprofile.ru/nastyasue/story/2
https://dprofile.ru/rusotoon/info
Good luck!
Забота о резиденции – это забота о спокойствии. Изоляция наружных поверхностей – это не только модный облик, но и обеспечение сохранения тепла в вашем уютном уголке. Наша бригада, коллектив экспертов, предлагаем вам сделать ваш дом в прекрасное место для жизни.
Наши работы – это не просто утепление, это искусство с каждым слоем. Мы предпочитаем гармонии между внешним видом и практической ценностью, чтобы ваш дом преобразился не только теплым и уютным, но и роскошным.
И самое важное – разумная цена! Мы уверены, что высококачественные услуги не должны быть дорогим удовольствием. [url=https://ppu-prof.ru/]Утепление дома снаружи стоимость[/url] начинается всего от 1250 рублей за квадратный метр.
Использование современных технологий и высококачественных материалов позволяют нам создавать утепление, которое долговечно и надежно. Забудьте о холодных стенах и дополнительных расходах на отопление – наше утепление станет вашим надежным экраном от холода.
Подробнее на [url=https://ppu-prof.ru/]https://www.ppu-prof.ru[/url]
Не откладывайте на потом заботу о удовольствии в вашем доме. Обращайтесь к квалифицированным специалистам, и ваше жилье станет настоящим художественным творением, которое принесет вам тепло и удовлетворение. Вместе мы создадим дом, в котором вам будет по-настоящему удобно!
[url=http://prednisolone.directory/]prednisolone 5mg price uk[/url]
->>>>>>>>>>>>>как приворожить парня чтоб любил<<<<<<<<<-
______________чтобы любимый позвонил заговор _____________
снять наговор самостоятельно с мужчины
как убрать приворот с мужчины, а также:
->>>>>>заклинания любви на девушку
->>>>>>как снять с себя любовный приворот
->>>>>>приворот на пороге читать
->>>>>>заговор на любимую
еврейский приворот
🚀 Wow, this blog is like a cosmic journey launching into the universe of endless possibilities! 💫 The mind-blowing content here is a captivating for the imagination, sparking awe at every turn. 🌟 Whether it’s lifestyle, this blog is a goldmine of exciting insights! #AdventureAwaits 🚀 into this exciting adventure of knowledge and let your mind roam! 🚀 Don’t just explore, savor the excitement! #BeyondTheOrdinary Your mind will be grateful for this exciting journey through the dimensions of endless wonder! 🌍
[url=http://albuterol.cyou/]cheap allbuterol[/url]
[url=https://fluconazole.cyou/]where can i buy diflucan online[/url]
[url=https://neurontin.cyou/]neurontin 900[/url]
[url=https://gabapentin.cfd/]neurontin 500 mg[/url]
[url=https://zoloft.cfd/]generic zoloft 40 mg[/url]
[url=http://doxycycline.cyou/]how to get doxycycline prescription[/url]
[url=https://ivermectin.guru/]stromectol tab[/url]
[url=https://augmentin.best/]cost of augmentin tablets[/url]
Привет всем!
Новинки интим-товаров: обзоры и отзывы!
Хотите узнать о последних тенденциях в мире интим-товаров? https://satisfucktor.ru предлагает вам самые свежие обзоры и
рейтинги секс-игрушек, эротического белья, лубрикантов и многого другого. Наши эксперты тщательно изучают каждый товар,
чтобы помочь вам сделать осознанный выбор. Подробные обзоры и сравнительные характеристики помогут вам найти идеальный
продукт для ваших интимных удовольствий. Посетите Satisfucktor.ru и окунитесь в мир страсти и удовольствия!
[url=https://satisfucktor.ru/obzory/vibr/obzor-pokupatelya-na-vibropulyu-perezaryazhaemuyu-glitz-royal-gems-roy004-shots-media-osnovnye-harakteristiki-instrukcziya-i-uhod/]Обзор покупателя на Надувной вибратор Inflatable G-wand – основные характеристики, инструкция и уход[/url]
[url=https://satisfucktor.ru/obzory/obzor-telesnogo-falloimitatora-mr-strong-19-sm-osobennosti-ispolzovaniya-instruktsiya-preimushestva-i-nedostatki/]Потребительский обзор – “Приятные мелочи Презервативы. Классические презервативы Contex Classic – 3 шт.” – инструкция, преимущества и недостатки – полный разбор от покупателя[/url]
[url=https://satisfucktor.ru/obzory/vibr/obzor-pokupatelya-na-vibrator-realistik-iz-kiberkozhi-s-moshonkoj-%e2%84%9659-osnovnye-harakteristiki-instrukcziya-i-uhod-za-vibrator-realistik-iz-kiberkozhi-s-moshonkoj-%e2%84%9659/]Обзор покупателя на Вибратор-реалистик из киберкожи с мошонкой №59. Основные характеристики, инструкция и уход за Вибратор-реалистик из киберкожи с мошонкой №59[/url]
[url=https://satisfucktor.ru/obzory/bdsm/obzor-na-stek-krasnyj-kozhanyj-instrukcziya-osobennosti-ispolzovaniya-dlya-bdsm-igr-sovety-po-ismolzovani-stek-krasnyj-kozhanyj-dlya-czenitelej-bdsm/]Обзор на Стек с хлопушкой петлей Sitabella – инструкция, особенности использования для БДСМ игр, советы по использованию. Стек с хлопушкой петлей Sitabella для ценителей BDSM[/url]
[url=https://satisfucktor.ru/obzory/bdsm/naruchniki-maya-instrukcziya-osobennosti-ispolzovaniya-sovety-dlya-bdsm-igrnikov/]Обзор на Наручники однослойные кожа Pecado BDSM – инструкция, особенности использования для БДСМ игр, советы по использованию. Наручники однослойные кожа Pecado BDSM для ценителей BDSM[/url]
[url=https://satisfucktor.ru/obzory/vibr/obzor-pokupatelya-na-klassicheskij-silikonovyj-vibrator-4sexdream-osnovnye-harakteristiki-instrukcziya-i-uhod/]Мастурбатор-вагина в колбе для мужчин – обзоры, отзывы и особенности использования реалистичного вибромастурбатора[/url]
[url=https://satisfucktor.ru/masturbator/masturbator-vagina-diana-dlya-muzhchin-realistichnoe-ustrojstvo-dlya-udovletvoreniya-proverennye-obzory-i-otzyvy/]Ролевые костюмы для секса – Мужской костюм Официанта La Blinque. Обзор ролевого белья из магазина для взрослых[/url]
*****
https://satisfucktor.ru/obzory/bdsm/obzor-na-krasnyj-oshejnik-s-kolechkom-instrukcziya-osobennosti-ispolzovaniya-dlya-bdsm-igr-sovety-po-ispolzovaniyu-krasnyj-oshejnik-s-kolechkom-dlya-czenitelej-bdsm/
https://satisfucktor.ru/obzory/lubrikanty/obzor-na-shipuchuyu-sol-dlya-vann-saharnyj-arbuz-candy-bath-bar-laboratoriya-katrin-volshebstvo-dlya-relaksaczii-i-uhoda/
https://satisfucktor.ru/obzory/vibr/obzor-pokupatelya-na-abbott-vibronasadka-na-2-palcza-s-rebristoj-poverhnostyu-baile-pretty-love-osnovnye-harakteristiki-instrukcziya-i-uhod/
https://satisfucktor.ru/obzory/obzor-i-instrukcziya-po-ispolzovaniyu-steklyannyh-i-metallicheskih-analnyh-igrushek-bolshoj-stalnoj-plag-s-chernym-kristallom-black-rave-95-sm-preimushhestva-i-nedostatki/
https://satisfucktor.ru/obzory/lubrikanty/chestnyj-obzor-na-analnuyu-probku-colorful-joy-medium-s-fioletovym-kristallom-instrukcziya-ispolzovaniya-i-sovety-po-uhodu/
https://satisfucktor.ru/obzory/strapon/preimushhestva-i-nedostatki-realistichnoj-nasadki-7-realistic-perfect-erect-cock-185-sm-osobennosti-ispolzovaniya-i-kratkaya-instrukcziya/
https://satisfucktor.ru/obzory/lubrikanty/chestnyj-obzor-na-nabor-analnyh-probok-silicone-curve-anal-kit-kak-ispolzovat-instrukcziya-i-sovety-po-uhodu/
Удачи!
[url=http://retina.directory/]retin a cheapest price[/url]
[url=https://robaxin.cyou/]robaxin online canada[/url]
[url=https://bactrim.cyou/]bactrim 800 160 mg tablet[/url]
[url=https://vardenafil.cyou/]buying levitra[/url]
[url=https://semaglutidewegovy.online/]rybelsus for sale[/url]
Hi everyone
One day my friend showed me an interesting and colorful game, just like from My childhood, fruit combinations, very fascinating and most importantly develops logic and luck, it does not oblige to anything and helps to brighten up the time!
Once you understand how the internal mechanism of the slot works, it’s time to answer the question: “how to win?” Since each round of the game is completely dependent on the random number generator and is unpredictable, it is important to understand which strategy can be most effective and start testing it, preferably in demo mode, so as not to risk real funds. Despite the fact that the mathematical expectation remains negative in general, the chances of winning still exist for each player.
If you want to find out how to win in this wonderful slot, then one of the real solutions may be to set the bet until your balance doubles. It is important not to continue playing if you are unlucky and the money starts to run out.
The best site for playing sweet bonanza – https://sweet-bonanza-game.ru !
In the game, it is important to aim for 4 or more lollipop symbols, as this activates free spins, sweet bombs and multiplayer!
Good luck friends!
[url=https://sweet-bonanza-game.ru]sweet bonanza great.com[/url]
[url=https://sweet-bonanza-game.ru]max win sweet bonanza[/url]
[url=https://sweet-bonanza-game.ru]free spins sweet bonanza[/url]
[url=https://sweet-bonanza-game.ru]sweet bonanxa[/url]
[url=https://sweet-bonanza-game.ru]1xbet sweet bonanza[/url]
casino-bonanza-online
sweet bonanza рубли
sweet bonzana online
sweet bonanza slots
situs sweet bonanza candyland
sweet bonanza casino
play slot bonanza online
[url=https://rybelsus.best/]buy semaglutide online canada[/url]
[url=http://ozempic.quest/]buy ozempic online from india[/url]
[url=https://semaglutide.quest/]wegovy medication[/url]
[url=https://semaglutidewegovy.shop/]rybelsus diabetes medication[/url]
[url=http://semaglutidewegovy.online/]rybelsus tab 7mg[/url]
Hello
One day my friend showed me an interesting and colorful game, just like from My childhood, fruit combinations, very fascinating and most importantly develops logic and luck, it does not oblige to anything and helps brighten up the time!
Once you understand how the internal mechanism of the slot works, it’s time to answer the question: “how to win?” Since each round of the game is completely dependent on the random number generator and is unpredictable, it is important to understand which strategy can be most effective and start testing it, preferably in demo mode, so as not to risk real funds. Despite the fact that the mathematical expectation remains negative in general, the chances of winning still exist for each player.
If you want to find out how to win in this wonderful slot, then one of the real solutions may be to set the bet until your balance doubles. It is important not to continue playing if you are unlucky and the money starts to run out.
The best site for playing sweet bonanza – https://sweet-bonanza-game.ru !
In the game, it is important to aim for 4 or more lollipop symbols, as this activates free spins, sweet bombs and multiplayer!
Good luck friends!
[url=https://sweet-bonanza-game.ru]how to play sweet bonanza in us[/url]
[url=https://sweet-bonanza-game.ru]sweet bonanza real money australia[/url]
[url=https://sweet-bonanza-game.ru]sweetbonanta demo[/url]
[url=https://sweet-bonanza-game.ru]slot sweet bonanza[/url]
[url=https://sweet-bonanza-game.ru]бонанза демо версия[/url]
sweet bonanza slot free play
free spins sweet bonanza
slots sweet bonanza
swetbonanza
play sweet bonanza demo
sweet bonanza app
bonanza slot oyna
Привет всем!
Хочу всем посоветовать лучшую компаний по дезинсекции и уничтожения насекомых в Москве, это действительно помогло мне решить проблемы и начать жить спокойно!
Уничтожение тараканов: эффективные методы, безопасность и профессиональные услуги
Тараканы в доме могут вызывать не только физическое недомогание, но и создавать проблемы с гигиеной и комфортом. Для решения этой неприятной ситуации широко применяются различные методы уничтожения тараканов, включая дезинсекцию и применение инсектицидов.
Профессиональная дезинсекция:
Один из самых эффективных способов избавления от тараканов — профессиональная дезинсекция. Специалисты проводят тщательную обработку помещения с использованием высокоэффективных инсектицидов, которые эффективно уничтожают тараканов на всех стадиях их развития. Профессионалы также применяют индивидуальный подход, учитывая особенности помещения и степень зараженности.
Вот сайт профессионалов дезинсекции: https://dezinfekciya-mcd.ru
[url=https://dezinfekciya-mcd.ru/tarakan/]уничтожение тараканов в магазине[/url]
[url=https://dezinfekciya-mcd.ru/unichtozhenie/plesen/]уничтожение грибка в квартире[/url]
[url=https://dezinfekciya-mcd.ru/unichtozhenie/muravi/]уничтожение муравьев[/url]
Безопасность и гарантия результата:
При проведении уничтожения тараканов специалисты обеспечивают полную безопасность для здоровья жильцов и домашних животных. Инсектициды, используемые при дезинсекции, отличаются низкой токсичностью для людей и животных, при этом обеспечивая высокую эффективность в борьбе с тараканами. Гарантия результата после профессиональной обработки обеспечивает долгосрочную защиту от повторного появления вредителей.
Удачи!
услуги дезинсекции
уничтожение мышей в москве
поморить тараканов
служба травления тараканов
дезинсекция в москве
[url=https://ozempic.company/]ozempic tab 7mg[/url]
[url=http://semaglutide.download/]buy semaglutide[/url]
[url=http://rybelsus.cyou/]semaglutide online uk[/url]
[url=http://rybelsus.best/]buy ozempic online no script[/url]
[url=https://semaglutideozempic.online/]brand semaglutide[/url]
[url=https://semaglutiderybelsus.shop/]buy ozempic online no script needed[/url]
Привет всем!
Хочу всем посоветовать лучшую компаний по дезинсекции и уничтожения насекомых в Москве, это действительно помогло мне решить проблемы и начать жить спокойно!
Уничтожение тараканов: эффективные методы, безопасность и профессиональные услуги
Тараканы в доме могут вызывать не только физическое недомогание, но и создавать проблемы с гигиеной и комфортом. Для решения этой неприятной ситуации широко применяются различные методы уничтожения тараканов, включая дезинсекцию и применение инсектицидов.
Профессиональная дезинсекция:
Один из самых эффективных способов избавления от тараканов — профессиональная дезинсекция. Специалисты проводят тщательную обработку помещения с использованием высокоэффективных инсектицидов, которые эффективно уничтожают тараканов на всех стадиях их развития. Профессионалы также применяют индивидуальный подход, учитывая особенности помещения и степень зараженности.
Вот сайт профессионалов дезинсекции: https://dezinfekciya-mcd.ru
[url=https://dezinfekciya-mcd.ru/unichtozhenie/klopov/]избавиться от клопов в москве[/url]
[url=https://dezinfekciya-mcd.ru/unichtozhenie/plesen/]уничтожение плесени[/url]
[url=https://dezinfekciya-mcd.ru/unichtozhenie/klesch/]обработка участка от клещей цена[/url]
Безопасность и гарантия результата:
При проведении уничтожения тараканов специалисты обеспечивают полную безопасность для здоровья жильцов и домашних животных. Инсектициды, используемые при дезинсекции, отличаются низкой токсичностью для людей и животных, при этом обеспечивая высокую эффективность в борьбе с тараканами. Гарантия результата после профессиональной обработки обеспечивает долгосрочную защиту от повторного появления вредителей.
Удачи!
обработка участка от комаров и клещей
избавиться от клопов в москве
уничтожение тараканов в москве
служба по выведению тараканов
уничтожение клопов с гарантией
[url=http://ozempictabs.com/]rybelsus 3mg[/url]
[url=https://wegovy.trade/]buy rybelsus from canada[/url]
[url=https://rybelsussemaglutide.online/]rybelsus generic cost[/url]
[url=http://rybelsus.monster/]rybelsus 7 mg tablet[/url]
[url=https://rybelsus.download/]rybelsus canada[/url]
[url=https://ozempic.company/]buy rybelsus[/url]
Доброго!
Меня зовут Вика)
Учусь в универе на 4 курсе, время дипломных подготовок, курсовых и рефератов, но Я девушка и у меня другие интересы….
Пришло время расплаты, и меня чуть не отчислили, хорошо подруга сказала что оказывается можно купить диплом или курсовую, конечно Вы не получите твердую 5, но 4 или 3 точно, пройдете дальше по учебе что и необходимо)
Вот сайт компании, дипломы на заказ для любых вузов под ключ: http://dodip.ru/
[url=http://dodip.ru/]купить диплом в гатчине[/url]
[url=http://dodip.ru/]купить диплом[/url]
[url=http://dodip.ru/]купить аттестат школы[/url]
Кстати у конкурентов сразу просили предоплату, каике-то там условия это пугает, тут ничего такого нет, все после результата, спасибо им большое!
Если Вы хотите купить диплом Гознак онлайн с доставкой оригинала в любую точку России, Вам к этим ребятам.
Удачи и хороших оценок!
купить диплом в подольске
купить диплом в серове
купить диплом колледжа
купить диплом в невинномысске
где купить диплом
купить диплом в нефтекамске
купить диплом в крыму
купить диплом в керчи
Здравствуйте!
Меня зовут Вика)
Учусь в универе на 4 курсе, время дипломных подготовок, курсовых и рефератов, но Я девушка и у меня другие интересы….
Пришло время расплаты, и меня чуть не отчислили, хорошо подруга сказала что оказывается можно купить диплом или курсовую, конечно Вы не получите твердую 5, но 4 или 3 точно, пройдете дальше по учебе что и необходимо)
Вот сайт компании, дипломы на заказ для любых вузов под ключ: http://dodip.ru/
[url=http://dodip.ru/]купить диплом в новоуральске[/url]
[url=http://dodip.ru/]купить диплом охранника[/url]
[url=http://dodip.ru/]купить диплом матроса[/url]
Кстати у конкурентов сразу просили предоплату, каике-то там условия это пугает, тут ничего такого нет, все после результата, спасибо им большое!
Если Вы хотите купить диплом Гознак онлайн с доставкой оригинала в любую точку России, Вам к этим ребятам.
Удачи и хороших оценок!
купить диплом в пскове
купить диплом физика
купить диплом в кирове
купить диплом в петропавловске-камчатском
купить диплом в ишиме
купить диплом сантехника
купить диплом техника
купить диплом в челябинске
[url=http://semaglutiderybelsus.online/]where to buy semaglutide[/url]
[url=http://semaglutidetabs.online/]semaglutide medication[/url]
Привет всем!
Были ли у вас случаи, когда вам приходилось писать дипломную работу в сжатые сроки? Это действительно трудно и ответственно, но важно не сдаваться и продолжать работать над учебными процессами, чем я и занимаюсь.
Для тех, кто умеет грамотно пользоваться интернетом и находить нужную информацию, это действительно оказывается полезным. Это помогает в процессе согласования и написания дипломной работы, и нет необходимости тратить время на посещение библиотек или встречи с дипломным руководителем. Если вам нужны хорошие источники для подготовки дипломных и курсовых работ, я могу поделиться полезными ссылками.
http://vuzdiploma.ru/
Желаю всем отличных оценок!
купить диплом сантехника
купить диплом образцы
купить диплом мастера маникюра и педикюра
купить диплом цена
купить диплом в кирове
купить диплом в санкт-петербурге
купить диплом в череповце
купить бланк диплома
купить диплом массажиста
купить аттестат за 9 классов
Здравствуйте!
Диплом – это кошмар. Срыв сроков, здоровье пошло на убыль, но интернет помогает найти методы ускорения работы. Не сдаюсь.
http://www.52ru.ru/showthread.php?p=37402#post37402
http://best-broker.name/forum/34——4–mt5/29748—.html#29748
Желаю всем отличных оценок!
куплю диплом
купить диплом в калуге
купить диплом оценщика
купить диплом в воронеже
купить диплом в тамбове
купить свидетельство о рождении ссср
купить диплом с реестром
купить диплом в пятигорске
куплю диплом о высшем образовании
купить диплом продавца
[url=http://ozempic.us.org/]rybelsus semaglutide[/url]
🚀 Wow, blog ini seperti petualangan fantastis melayang ke galaksi dari kegembiraan! 💫 Konten yang menarik di sini adalah perjalanan rollercoaster yang mendebarkan bagi imajinasi, memicu kagum setiap saat. 🎢 Baik itu teknologi, blog ini adalah harta karun wawasan yang mendebarkan! #TerpukauPikiran Berangkat ke dalam petualangan mendebarkan ini dari imajinasi dan biarkan imajinasi Anda terbang! 🌈 Jangan hanya mengeksplorasi, alami kegembiraan ini! #MelampauiBiasa Pikiran Anda akan bersyukur untuk perjalanan mendebarkan ini melalui alam keajaiban yang menakjubkan! 🌍
Hello everyone!
I recommend visiting these resources, there is a lot of useful structured information, for any questions and just to take a break from all the work:
http://molbiol.ru/forums/index.php?showtopic=1061138
https://forum.bocu.ro/viewtopic.php?p=80599#80599
Good luck! If you know similar ones, be sure to let us know)
Hello everyone!
I suggest visiting these resources for a wealth of valuable, well-organized information, whether you have questions or simply need a break from your workload:
https://batcheres.bcbloggers.com/24548450/discover-the-uniqueness-of-stellar-spins-casino
https://nowewyrazy.uw.edu.pl/profil/pokiesnet07
Good luck! If you know similar ones, be sure to let us know)
Hello everyone!
I recommend visiting these resources, there is a lot of useful structured information, for any questions and just to take a break from all the work:
http://bereg.webtalk.ru/viewtopic.php?id=10178#p30372
https://directory.thenorthernecho.co.uk/company/59b7a71abda1a7464ea84fb77114bb76
Good luck! If you know similar ones, be sure to let us know)
Hello everyone!
I recommend visiting these resources, there is a lot of useful structured information, for any questions and just to take a break from all the work:
http://www.dalby.com.au/new-south-wales/bateau-bay/arts-entertainment-and-leisure/national-casino
http://www.artisteer.com/Default.aspx?post_id=267508&p=forum_post&forum_id=9
Good luck! If you know similar ones, be sure to let us know)
Hello everyone!
I suggest checking out these resources, where you’ll find plenty of helpful, well-organized information, whether you have questions or just need a break from your work:
https://hypothes.is/users/reelsroyal
https://www.pampling.com/comunidad/blog-de-usuarios/151120-rozrywki
Good luck! If you know similar ones, be sure to let us know)
Hello everybody!
Have you ever heard of X-GPT Writer: a unique keyword content generator based on the ChatGPT neural network?
I also haven’t, until I was advised to automate routine tasks with this software, I want to say one thing! For a long time afterwards, I couldn’t believe
that ChatGPT was such a powerful product if it was used simultaneously in streaming, running X-GPT Writer.
I thought it was just a utility, it was inexpensive, a friend gave me a coupon for a 40% discount%:
94EB516BCF484B27
the details of where to enter it are indicated on the website:
https://www.xtranslator.ru/x-gpt-writer/
I started trying, delving into it, bought 50 ChatGPT accounts at low prices and off I went!
Now I easily generate and launch 3-4 new sites per week, batch unify entire folders and even create images
using the ChatGPT neural network and X-GPT Writer.
It’s worth a try, Friends, there’s a demo, everything is free, you won’t regret it)
Good luck!
ChatGPT и X-GPTWriter в качестве инструментов синонимизации
X-GPTWriter: купоны и акции на скидки
X-GPTWriter купить со скидкой
Генератор синонимов с ChatGPT
ChatGPT как инструмент для генерации контента
программа создания контента через ChatGPT
Где найти промо коды на X-GPTWriter
Как сделать текст более уникальным с ChatGPT
X-GPTWriter: мощный инструмент для контент-креации
ChatGPT и X-GPTWriter: новая эра контент-маркетинга
Здравствуйте!
Были ли у вас случаи, когда вам приходилось писать дипломную работу в сжатые сроки? Это действительно трудно и ответственно, но важно не сдаваться и продолжать работать над учебными процессами, чем я и занимаюсь.
Для тех, кто умеет грамотно пользоваться интернетом и находить нужную информацию, это действительно оказывается полезным. Это помогает в процессе согласования и написания дипломной работы, и нет необходимости тратить время на посещение библиотек или встречи с дипломным руководителем. Если вам нужны хорошие источники для подготовки дипломных и курсовых работ, я могу поделиться полезными ссылками.
http://vuzdiploma.ru/
Желаю всем отличных оценок!
купить диплом в новом уренгое
купить диплом в новороссийске
купить диплом в белогорске
купить диплом монтажника
купить диплом в ялте
купить диплом в петрозаводске
купить диплом в челябинске
купить диплом в иваново
купить диплом в северске
купить диплом эколога
Привет всем!
Я начал делать сетку сайтов, все было не в поиске, но прогон Bullet помог
У нас была жесткая тематика, но наш сайт пошел выше после работы парней с Bullet
https://www.google.com/search?q=%D0%BF%D1%80%D0%BE%D0%B3%D0%BE%D0%BD+%D0%B4%D0%BE%D1%80%D0%BE%D0%B2+bullet+kwork&sca_esv=46579d9512b829aa&ei=_hHSZaflIZKBxc8Psd2i6AY&udm=&ved=0ahUKEwin3MnYirWEAxWSQPEDHbGuCG0Q4dUDCBA&uact=5&oq=%D0%BF%D1%80%D0%BE%D0%B3%D0%BE%D0%BD+%D0%B4%D0%BE%D1%80%D0%BE%D0%B2+bullet+kwork&gs_lp=Egxnd3Mtd2l6LXNlcnAiJNC_0YDQvtCz0L7QvSDQtNC-0YDQvtCyIGJ1bGxldCBrd29yazIJECEYChigARgqSKYVUJ4EWO8ScAN4AJABAZgBrQKgAccNqgEFMi02LjG4AQPIAQD4AQHCAgcQIRgKGKABiAYB&sclient=gws-wiz-serp
https://www.google.com/search?q=%D0%BF%D1%80%D0%BE%D0%B3%D0%BE%D0%BD+%D1%81%D0%B0%D0%B9%D1%82%D0%B0+bullet&sca_esv=46579d9512b829aa&ei=PAjSZb-wLdSP1fIPxeKh6AM&udm=&ved=0ahUKEwi_vsOxgbWEAxXUR1UIHUVxCD0Q4dUDCBA&uact=5&oq=%D0%BF%D1%80%D0%BE%D0%B3%D0%BE%D0%BD+%D1%81%D0%B0%D0%B9%D1%82%D0%B0+bullet&gs_lp=Egxnd3Mtd2l6LXNlcnAiHtC_0YDQvtCz0L7QvSDRgdCw0LnRgtCwIGJ1bGxldEj_GlC0BljhGHAGeAGQAQKYAYYCoAG9EaoBBTAuMi44uAEDyAEA-AEBwgIKEAAYRxjWBBiwA8ICCBAhGKABGMMEwgIMECEYChigARjDBBgqwgIKECEYChigARjDBIgGAZAGCA&sclient=gws-wiz-serp
Удачи!
Привет всем!
Мой сайт стоял на месте, но данный продукт смог его раскачать
Я начал делать сетку сайтов, все было не в поиске, но прогон Bullet помог
https://www.google.com/search?q=%D0%BF%D1%80%D0%BE%D0%B4%D0%B2%D0%B8%D0%B6%D0%B5%D0%BD%D0%B8%D0%B5+%D1%81%D0%B0%D0%B9%D1%82%D0%B0+%D0%B2+google+%D1%82%D0%B0%D1%80%D0%B8%D1%84+bullet+kwork&sca_esv=46579d9512b829aa&ei=LxzSZZCSGrygwPAP8fGNmAQ&udm=&ved=0ahUKEwjQ5cq0lLWEAxU8EBAIHfF4A0MQ4dUDCBA&uact=5&oq=%D0%BF%D1%80%D0%BE%D0%B4%D0%B2%D0%B8%D0%B6%D0%B5%D0%BD%D0%B8%D0%B5+%D1%81%D0%B0%D0%B9%D1%82%D0%B0+%D0%B2+google+%D1%82%D0%B0%D1%80%D0%B8%D1%84+bullet+kwork&gs_lp=Egxnd3Mtd2l6LXNlcnAiQ9C_0YDQvtC00LLQuNC20LXQvdC40LUg0YHQsNC50YLQsCDQsiBnb29nbGUg0YLQsNGA0LjRhCBidWxsZXQga3dvcmsyCBAhGKABGMMESPVMUOYcWJ5FcAZ4AZABA5gB8AGgAY8UqgEGMC4xMC40uAEDyAEA-AEBwgIKEAAYRxjWBBiwA8ICCBAAGIkFGKIEwgIMECEYChigARjDBBgqiAYBkAYI&sclient=gws-wiz-serp
https://www.google.com/search?q=%D0%BF%D1%80%D0%BE%D0%B3%D0%BE%D0%BD+%D0%B4%D0%BE%D1%80%D0%BE%D0%B2+bullet+kwork&sca_esv=46579d9512b829aa&ei=_hHSZaflIZKBxc8Psd2i6AY&udm=&ved=0ahUKEwin3MnYirWEAxWSQPEDHbGuCG0Q4dUDCBA&uact=5&oq=%D0%BF%D1%80%D0%BE%D0%B3%D0%BE%D0%BD+%D0%B4%D0%BE%D1%80%D0%BE%D0%B2+bullet+kwork&gs_lp=Egxnd3Mtd2l6LXNlcnAiJNC_0YDQvtCz0L7QvSDQtNC-0YDQvtCyIGJ1bGxldCBrd29yazIJECEYChigARgqSKYVUJ4EWO8ScAN4AJABAZgBrQKgAccNqgEFMi02LjG4AQPIAQD4AQHCAgcQIRgKGKABiAYB&sclient=gws-wiz-serp
Удачи!
[url=https://semaglutide.download/]rybelsus canada pharmacy prices[/url]
nice content!nice history!! boba 😀
wow, amazing
nice content!nice history!! boba 😀
wow, amazing
nice content!nice history!! boba 😀
nice content!nice history!! boba 😀
nice content!nice history!! boba 😀
nice content!nice history!! boba 😀
nice content!nice history!! boba 😀
Super, fantastic
Great job
Excellent effort
Impressive, fantastic
Marvelous, impressive
wow, amazing
nice content!nice history!! boba 😀
Thank you, I’ve recently been looking for information about this topic for ages and yours is the greatest I have discovered till now.
However, what about the bottom line? Are
you sure concerning the supply?
wow, amazing
nice content!nice history!! boba 😀
nice content!nice history!! boba 😀
wow, amazing
Excellent write-up
wow, amazing
nice content!nice history!! boba 😀
wow, amazing
nice content!nice history!! boba 😀
[url=https://prednisoneo.com/]prednisone buy cheap[/url]
[url=https://happyfamilymedicalstore.online/]escrow pharmacy canada[/url]
[url=http://oazithromycin.online/]where can i get zithromax[/url]
phising
Уютный уголок для семьи
6. Стильный дом из бруса 9х12: современный дизайн
дома из бруса 9х12 [url=https://domizbrusa9x12spb.ru/]https://domizbrusa9x12spb.ru/[/url] .
I strongly recommend to avoid this site. My personal experience with it was only frustration as well as suspicion of deceptive behavior. Proceed with extreme caution, or alternatively, look for a more reputable site to meet your needs.
I strongly recommend to avoid this site. My personal experience with it was purely frustration as well as doubts about fraudulent activities. Be extremely cautious, or alternatively, look for an honest site to meet your needs.
I urge you steer clear of this site. The experience I had with it has been only disappointment and suspicion of scamming practices. Be extremely cautious, or alternatively, look for a trustworthy platform to fulfill your requirements.
I urge you stay away from this platform. The experience I had with it was nothing but frustration along with concerns regarding deceptive behavior. Proceed with extreme caution, or better yet, look for an honest site for your needs.
I strongly recommend stay away from this platform. The experience I had with it has been nothing but frustration along with doubts about deceptive behavior. Exercise extreme caution, or alternatively, find a trustworthy service for your needs.
[url=http://happyfamilystorerx.online/]best european online pharmacy[/url]
[url=https://happyfamilymedicalstore.online/]legal online pharmacies in the us[/url]
[url=https://ezithromycin.online/]zithromax 500mg[/url]
[url=https://oprednisone.online/]prednisone over the counter[/url]
[url=https://oprednisone.online/]prednisone 10mg buy online[/url]
[url=http://azithromycinps.online/]azithromycin 500mg buy[/url]
[url=https://valtrexid.com/]generic for valtrex buy without a prescription[/url]
[url=https://azithromycinps.online/]azithromycin cost india[/url]
[url=http://valtrexmedication.com/]generic valtrex 1000mg for sale[/url]
[url=http://oazithromycin.com/]azithromycin 600 mg tablet[/url]
[url=https://predniso.online/]200 mg prednisone[/url]
[url=http://azithromycinmds.com/]azithromycin 500 mg tablet price in india[/url]
[url=http://metformin.store/]buy metformin online singapore[/url]
[url=https://prednisonekx.online/]buy prednisone online canada without prescription[/url]
[url=http://valtrexarb.online/]valtrex medicine price[/url]
[url=http://metforminn.com/]glucophage online prescription[/url]
[url=http://prednisoneo.com/]prednisone 60 mg daily[/url]
[url=https://prednisoneo.online/]prednisone 20 mg generic[/url]
[url=http://happyfamilymedicalstore.online/]online pharmacy delivery usa[/url]
Renew your home’s appearance, comfort with window replacements.
[url=http://azithromycinps.online/]how much is azithromycin in mexico[/url]
[url=http://happyfamilystorerx.online/]pharmacy online 365 discount code[/url]
[url=https://oprednisone.com/]generic prednisone over the counter[/url]
[url=https://happyfamilymedicalstore.online/]what’s the best online pharmacy[/url]
[url=http://happyfamilymedicalstore.online/]foreign pharmacy no prescription[/url]
[url=http://happyfamilymedicalstore.online/]best rx pharmacy online[/url]
[url=https://happyfamilymedicalstore.online/]cheap canadian pharmacy[/url]
[url=http://valtrexmedication.com/]where to buy valtrex online[/url]
[url=http://prednisonecsr.com/]prednisone 5443[/url]
[url=https://valtrexmedication.online/]where can i get valtrex over the counter[/url]
[url=http://happyfamilymedicalstore.online/]top 10 pharmacy websites[/url]
[url=http://valtrexarb.online/]valtrex usa[/url]
Бро, когда ты на мото, девчонки просто сходят с ума! Я решил проверить эту теорию и зарегистрировался на сайте знакомств. И оказалось, что это правда – все красотки тут как на подбор, и все хотят с тобой покататься!
У меня есть мотоцикл, который просто сводит с ума. И когда я загружаю фото на нем на сайт знакомств, девчонки просто в очередь становятся! Все они с отличной фигурой, красивыми лицами и жаждой приключений.
Наши встречи – это настоящий адреналин! Мы катались по ночным улицам Москвы, ощущая ветер в волосах и адреналин в крови. И каждая из них была как новый поворот на моем мотоцикле – острая, захватывающая и незабываемая.
Флирт на сайтах знакомств – это не только способ познакомиться с классными девчонками, но и возможность окунуться в мир адреналина и приключений. Так что, парни, не теряйте времени, идите и покоряйте сердца этих красоток на ваших мото!
Твоя вторая половинка может ждать именно тебя на этих сайтах!
https://tuvape.es/minimalist-japanese-inspired-furniture-2/#comment-156386
https://www.quu.at/about/#comment-45500
https://whimwham.pk/product/pencil-sketch/#comment-2921
https://hainews.id/antisipasi-bahaya-listrik-pln-lahat-gelar-forum-keselamatan-ketenagalistrikan-bersama-stakeholder/#comment-15312
https://yugunga-nya.org.au/annual-report-2022-23-yugunga-nya-peoples-trust/#comment-695
http://www.reclick.lv/index.php/2020/06/18/hello-world/?unapproved=319690&moderation-hash=5d801bc02aae27e67d439157b1057995#comment-319690
https://torrezinfor.tech/couchbase-tiene-como-objetivo-aumentar-la-productividad-de-la-base-de-datos-de-los-desarrolladores-con-la-herramienta-capella-iq-ai/#comment-1483
https://www.arkade-games.com/how-to-be-in-the-flow-and-create-something-beautiful/#comment-34968
https://360ef.pl/seven-weeks-working-pro-bono-with-a-national-charity/#comment-34003
https://www.regenbogenwiese.net/thread/10815-afina-3/?postID=36205#post36205
[url=https://metforminbi.online/]cost for metformin[/url]
[url=https://happyfamilymedicalstore.online/]top 10 online pharmacy in india[/url]
[url=http://happyfamilystorerx.online/]reputable indian online pharmacy[/url]
[url=http://azithromycinmds.com/]how to buy azithromycin online usa[/url]
[url=https://valtrexid.com/]valtrex capsules[/url]
[url=http://metformindi.online/]metformin online usa no prescription[/url]
[url=http://prednisoneo.com/]prices for 5mg prednisone[/url]
[url=http://azithromycinps.online/]buy azithromycin nz[/url]
[url=https://oazithromycin.online/]azithromycin 500g[/url]
[url=https://predniso.online/]over the counter prednisone cream[/url]
[url=http://oprednisone.com/]prednisone 60 mg daily[/url]
[url=http://oazithromycin.com/]azithromycin 250 mg tabs[/url]
[url=https://metforminbi.online/]best generic metformin[/url]
[url=https://prednisonekx.online/]prednisone price south africa[/url]
[url=http://azithromycinhq.com/]zithromax 500mg cost[/url]
[url=https://ezithromycin.online/]azithromycin 500 mg mexico[/url]
[url=https://ametformin.com/]glucophage 250 mg[/url]
Заказать купленный диплом, онлайн.
Легальный способ купить диплом, без заморочек.
Купить диплом с гарантией качества, советы от профессионалов.
Почему выгодно купить диплом, все секреты.
Как купить диплом безопасно, без риска.
Лучшие предложения по покупке диплома, важные моменты.
Как купить диплом быстро, спешите.
Скрытая покупка дипломов, важная информация.
Лучшие дипломы для покупки, подробности на сайте.
Как купить диплом онлайн, подробности у нас.
Официальные документы для покупки, срочные варианты.
Легальная покупка дипломов, без рисков.
Официальные дипломы для покупки, гарантированный результат.
Купить диплом просто, подробности на сайте.
Как купить дипломы онлайн, безопасность на первом месте.
Почему стоит купить диплом здесь и сейчас, подробности на сайте.
Заказать диплом онлайн без проблем, подробности здесь.
Официальный документ об образовании, безопасность на первом месте.
купить диплом [url=7arusak-diploms.com]7arusak-diploms.com[/url] .
[url=http://diflucand.online/]diflucan 200 mg over the counter[/url]
[url=http://baclofem.com/]baclofen generic lioresal[/url]
[url=https://accutanemix.online/]accutane 10mg[/url]
[url=http://mcadvair.online/]how much is advair cost[/url]
XEvil 5.0 automatically solve most kind of captchas,
Including such type of captchas: ReCaptcha-2, ReCaptcha-3, Hotmail, Google captcha, SolveMedia, BitcoinFaucet, Steam, Amazon, Twitter, Microsoft, Twitch, Outlook, +12k
+ hCaptcha, ArkoseLabs FunCaptcha, ReCaptcha Enterprize supported in new XEvil 6.0!
1.) Fast, easy, precisionly
XEvil is the fastest captcha killer in the world. Its has no solving limits, no threads number limits
you can solve even 1.000.000.000 captchas per day and it will cost 0 (ZERO) USD! Just buy license for 59 USD and all!
2.) Several APIs support
XEvil supports more than 6 different, worldwide known API: 2captcha.com, anti-captcha (antigate), rucaptcha.com, death-by-captcha, etc.
just send your captcha via HTTP request, as you can send into any of that service – and XEvil will solve your captcha!
So, XEvil is compatible with hundreds of applications for SEO/SMM/password recovery/parsing/posting/clicking/cryptocurrency/etc.
3.) Useful support and manuals
After purchase, you got access to a private tech.support forum, Wiki, Skype/Telegram online support
Developers will train XEvil to your type of captcha for FREE and very fast – just send them examples
4.) How to get free trial use of XEvil full version?
– Try to search in Google “Home of XEvil”
– you will find IPs with opened port 80 of XEvil users (click on any IP to ensure)
– try to send your captcha via 2captcha API ino one of that IPs
– if you got BAD KEY error, just tru another IP
– enjoy! 🙂
– (its not work for hCaptcha!)
WARNING: Free XEvil DEMO does NOT support ReCaptcha, hCaptcha and most other types of captcha!
http://XEvil.Net/
Hello i am kavin, its my first occasion to commenting anyplace, when i read this article i thought i could also make comment due to this
good article.
Do you mind if I quote a few of your articles as long
as I provide credit and sources back to your website?
My blog is in the very same niche as yours and my visitors would definitely benefit
from a lot of the information you provide
here. Please let me know if this ok with you.
Thanks!
At MCI Clinic, we specialize in cataract treatment with extensive experience, state-of-the-art equipment, and affordable prices. Our skilled professionals perform advanced procedures such as phacoemulsification, ensuring precise removal of the cloudy lens and its replacement with a high-quality artificial one. The surgery is quick, safe, and minimally invasive, providing excellent outcomes for our patients.
Choosing MCI means benefiting from top-tier medical care without the high cost. We are committed to restoring your clear vision and improving your quality of life through effective, cost-efficient cataract treatments.
MCI Clinic – [url=https://mci.md/]operatia cataracta[/url]
[url=http://accutanemix.online/]pharmacy canadian accutane[/url]
[url=http://azithromycinmds.online/]azithromycin india[/url]
[url=http://flomaxms.online/]flomax 0.4 mg daily[/url]
[url=https://enolvadex.online/]where to get nolvadex[/url]
Its like you learn my thoughts! You seem to grasp so much approximately this, like
you wrote the e-book in it or something. I think that you could do with some %
to pressure the message home a little bit, however
other than that, this is wonderful blog. An excellent read.
I will certainly be back.
[url=http://asynthroid.online/]where can you get synthroid[/url]
[url=https://accutaneiso.com/]buy accutane online nz[/url]
[url=http://baclofenx.com/]baclofen price 10mg in usa[/url]
[url=https://sildenafilps.online/]where to buy sildenafil online[/url]
[url=http://advaird.com/]advair diskus 250 50 mcg[/url]
[url=http://acutanep.online/]how do i get accutane[/url]
[url=http://hackedcard.cvv2cvc.net]http://hackedcard.cvv2cvc.net[/url]
Item 1 Card Total Balance: $3 100 – Price $ 110.00
Item 3 Cards Total Balance $9 600 – Price $ 180.00
Item PayPal Transfers $500 – Price $ 49.00
Item PayPal Transfers $2 000 – Price $ 149.00
Item Western Union Transfers $ 1 000 – Price $ 99.00
Item Western Union Transfers $ 300 – Price $ 249.00
*Prices on the website may vary slightly
[url=http://hacked-cards.cvv2cvc.net]Hacked paypal acc Cloned cards[/url] Hacked Credit cards
[url=http://hackedcards.cvv2cvc.net]Buy Cloned Cards Shop Cloned cards[/url]
Item 1 Card Total Balance: $3 100 – Price $ 110.00
Item 3 Cards Total Balance $9 600 – Price $ 180.00
Item PayPal Transfers $500 – Price $ 49.00
Item PayPal Transfers $2 000 – Price $ 149.00
Item Western Union Transfers $ 1 000 – Price $ 99.00
Item Western Union Transfers $ 300 – Price $ 249.00
*Prices on the website may vary slightly
[url=http://hackedcard.cvv2cvc.net]http://hackedcard.cvv2cvc.net[/url] Dumps Paypal buy
Рекомендации по безопасной покупке диплома о высшем образовании
Полезные советы по покупке диплома о высшем образовании без риска
Вопросы и ответы: можно ли быстро купить диплом старого образца?
Полезные советы по безопасной покупке диплома о высшем образовании
Как получить диплом техникума официально и без лишних проблем
Советы по созданию квадратных номеров на заказ, Изготовление квадратных номеров как акцент в интерьере, Как подобрать стиль и цвет квадратного номера для вашего дома, Мастер-класс по ручной работе с квадратными номерами, Какой материал лучше всего подходит для квадратных номеров в доме, Оригинальные идеи использования квадратных номеров в дизайне, Советы по выбору и заказу квадратных номеров для двери в интернет-магазине, Почему квадратные номера стали трендом в дизайне дома, Как сделать интерьер вашего дома современным с помощью квадратных номеров, Как использовать квадратные номера для создания уютного и стильного интерьера, Идеи для оформления дверей с помощью квадратных номеров, Какие функции могут выполнять квадратные номера в доме, Какие материалы лучше всего подходят для квадратных номеров, Сравнение цен на изготовление квадратных номеров в различных городах, Как выбрать правильный цвет квадратного номера для дома, Идеи использования квадратных номеров в разных стилях интерьера, Квадратные номера с подсветкой: как сделать дом еще стильнее, Как выбрать квадратные номера для большой семьи.
Быстрая схема покупки диплома старого образца: что важно знать?
odincovoschool16.flybb.ru/viewtopic.php?f=2&t=924
Микрозайм без отказов и проверок с выдачей на карту. [url=https://t.me/s/mfo_2024_online]МФО 2024[/url] года.
KRAKEN – ссылка, зеркало, сайт, кракен зеркало
Где и как купить диплом о высшем образовании без лишних рисков
Как получить диплом стоматолога быстро и официально
Всё, что нужно знать о покупке аттестата о среднем образовании без рисков
kapitoshka-nk.ru/dwqa-questions/dwqa-ask-question/#
Полезные советы по безопасной покупке диплома о высшем образовании
guyspages.com/read-blog/2137
Как приобрести диплом техникума с минимальными рисками
Как купить аттестат 11 класса с официальным упрощенным обучением в Москве
Пошаговая инструкция по официальной покупке диплома о высшем образовании
gosprav.ru/gosudarstvo/3/index
На [url=https://mirtinvest.ru/]mirtinvest.ru[/url] представлена подборка из более 40 лицензированных микрофинансовых организаций, предлагающих микрокредиты на карту онлайн без отказов. Все компании соответствуют законодательным требованиям, а максимальная процентная ставка не превышает 0,8% в день. Минимальные требования к заемщикам и доступность для лиц от 18 лет делают этот сервис оптимальным решением для быстрого получения финансовой поддержки. Выберите подходящее предложение и получите деньги на карту в кратчайшие сроки.
Диплом техникума купить официально с упрощенным обучением в Москве
Как официально купить аттестат 11 класса с упрощенным обучением в Москве
Быстрая схема покупки диплома старого образца: что важно знать?
reddevils.cn/video-52?page=86&per-page=8
Процесс получения диплома стоматолога: реально ли это сделать быстро?
Приобретение диплома ПТУ с сокращенной программой обучения в Москве
romb4x4.ru/forum/threads/kuplju-diplomy-v-ufe-v880a.12629
Как официально купить аттестат 11 класса с упрощенным обучением в Москве
is 80 mg of prednisone a high dose order prednisone online cheap can you break prednisone in half
side effects of prednisone with alcohol https://cialisbag.com/ – prednisone over the counter
Как оказалось, купить диплом кандидата наук не так уж и сложно
Реально ли приобрести диплом стоматолога? Основные шаги
campusvirtualcoopecaja.com/web/blog/index.php?entryid=8
Можно ли быстро купить диплом старого образца и в чем подвох?
Официальная покупка диплома вуза с упрощенной программой обучения
newru.getbb.ru/viewtopic.php?f=29&t=1386
Рекомендации по безопасной покупке диплома о высшем образовании
bisound.com/forum/showthread.php?p=978814#post978814
Полезные советы по покупке диплома о высшем образовании без риска
ekocom.ru/forum/?PAGE_NAME=profile_view&UID=13757
Как приобрести аттестат о среднем образовании в Москве и других городах
Приобретение диплома ПТУ с сокращенной программой обучения в Москве
Как оказалось, купить диплом кандидата наук не так уж и сложно
garsonvape.ru/245-antenny-orenburg
Как официально приобрести аттестат 11 класса с минимальными затратами времени
bookmarksparkle.com/story17136231/где-купить-диплом
buy clomid us buy cheap clomid pills where to order azithromycin [url=https://zithromaxshopx247.top/]azithromycin 2024[/url] can i order azithromycin discount programs for cymbalta [url=https://cymbaltashopx247.top/]cymbalta for cheap[/url] can i buy cymbalta online desyrel 50 mg tablets desyrel 50 mg tablets desyrel buy cymbalta tablet size [url=https://cymbaltashopx247.top/]cymbalta online order[/url] cymbalta buy online order clomid online [url=https://clomidshopx247.top/]buy clomid uk[/url] cost clomid pills crestor buy uk how to buy cymbalta coumadin tablets contraindications buy lopressor online
[u][b] Здравствуйте![/b][/u]
Мы предлагаем дипломы.
[url=http://seriallove.bbok.ru/viewtopic.php?id=7752#p123841/]seriallove.bbok.ru/viewtopic.php?id=7752#p123841[/url]
Можно ли купить аттестат о среднем образовании? Основные рекомендации
Как быстро получить диплом магистра? Легальные способы
Узнайте стоимость диплома высшего и среднего образования и процесс получения
allods.my.games/forum/index.php?page=User&userID=135513
Приобретение диплома ПТУ с сокращенной программой обучения в Москве
Возможно ли купить диплом стоматолога, и как это происходит
derivsocial.org/read-blog/3061
dp.getbb.ru/viewtopic.php?f=3&t=1027
Всё, что нужно знать о покупке аттестата о среднем образовании без рисков
Идеи для изготовления креативных квадратных номеров, Оригинальные способы создания номеров в форме квадрата
Микрозаймы — это быстрый способ решить финансовые проблемы, но если подходить к этому необдуманно, можно легко попасть в долговую яму. Зачем ставить под угрозу свое финансовое будущее? На нашем [url=https://all-credit.ru/zaym-1000-rubley/ ]сайте[/url] вы найдете советы экспертов, которые помогут грамотно выбрать условия займа и избежать опасных ловушек. Основная ошибка заемщиков — это игнорирование деталей договора и выбор суммы, которую сложно вернуть. Почему важно оценить свои возможности до оформления? Как избежать высоких процентов и скрытых платежей? Ответы на эти вопросы собраны на [url=https://all-credit.ru/mfo-odobrenya-vsem-online/ ]странице[/url], где наши специалисты делятся рекомендациями для безопасного займа.
Полезные советы по безопасной покупке диплома о высшем образовании
Больше никаких отказов! [url=https://mirtinvest.ru/]mirtinvest.ru[/url] предлагает вам подборку проверенных МФО, выдающих займы онлайн без звонков и лишних проверок. Основные требования — это наличие паспорта и именной банковской карты. Мы гарантируем одобрение для 90% заявок на суммы до 30 000 рублей. Убедитесь, что получить займ может быть легко и быстро. Наш портал — это ваш надёжный путь к срочной финансовой помощи.
Узнайте стоимость диплома высшего и среднего образования и процесс получения
Привет, друзья!
Мы готовы предложить документы ВУЗов
[url=http://hebergementweb.org/threads/diplom-za-neskolko-dnej-legko-i-bystro.1814550/]hebergementweb.org/threads/diplom-za-neskolko-dnej-legko-i-bystro.1814550[/url]
Yes, you can play online roulette for real money. There are many online casinos that offer real money games – and the best ones are listed in our article. Yes, most online casinos are accessible via mobile while many offer real money roulette apps for an optimum experience. Whether you have an iPhone, Android or a tablet, you will be able to enjoy a classic game of roulette on the go. There is also the possibility to play online roulette for free with no download, depending on your casino and game of choice. You can email the site owner to let them know you were blocked. Please include what you were doing when this page came up and the Cloudflare Ray ID found at the bottom of this page. Go for the double dozen. Perhaps the best way to play roulette is to bet on two 12s at the same time. Doing this allows you to cover 24 numbers with just two bets. What’s more, when you wager equal amounts, you can still make a profit if the right number comes in. In other words, if you bet £5 and £5, the potential return on either is £5. Therefore, you’ll make a £5 profit and have almost 66% of the roulette table covered.
https://trentonmmnm318632.alltdesign.com/1-deposit-casino-bonus-canadian-dollars-49859318
Unlock the best free no deposit casino bonus promos in September 2024! Free no depositoffers are a great way to try out casino games without spending any money. We’ll guide you to the best offers for September, so you can explore new casinos and games risk-free. One of the best casinos out there, when you deposit they usually give u 1 week of bonuses of 50 free spins, and the withdrawals are really fast, the only “bad” thing can be the game providers because they only have rtg, and the best thing about this casino is that beside the other rtg casinos, this one and others of same… Note that you need to redeem bonus codes to benefit from the welcome bonuses for new players or get other rewards at Casino Brango. The Brango Casino free chip code is “THEKINGS,” while the 200% boost without rules comes with the code “LIMITLESS CODE.”
Приобретение диплома ПТУ с сокращенной программой обучения в Москве
Как приобрести диплом о среднем образовании в Москве и других городах
polskiefajerwerki.net/users/view/17869
Легальная покупка диплома о среднем образовании в Москве и регионах
matchfishing.ru/forumtest/1/member.php?u=23110
Сколько стоит получить диплом высшего и среднего образования легально?
Как правильно приобрести диплом колледжа или ПТУ в России, важные моменты
ukrdiplom.com/stoimost-attestata-2024
Если вы хотите подчеркнуть стиль своему автомобилю и создать неповторимый образ, то на сайте baikalwheels.ru у вас есть возможность [url=https://baikalwheels.ru/catalog?type=litye_diski]литые диски в белгороде купить[/url] популярных мировых брендов. В каталоге представлены такие производители, как Replika, Nitro, Legeartis и K&K, которые известны надежностью. Размеры варьируются от 16 до 22 дюймов, что позволяет подобрать идеальные диски для любых автомобилей — от седанов до внедорожников.
Сколько стоит получить диплом высшего и среднего образования легально?
mediamemorial.ru/club/user/69594/forum/message/4259/13741
Стоимость дипломов высшего и среднего образования и как избежать подделок
Стоимость дипломов высшего и среднего образования и как избежать подделок
KRAKEN – ссылка, зеркало, сайт, кракен официальные ссылки
Официальная покупка школьного аттестата с упрощенным обучением в Москве
Стоимость дипломов высшего и среднего образования и как избежать подделок
polosedan-club.com/search/search
Приобретение диплома ПТУ с сокращенной программой обучения в Москве
Полезные советы по покупке диплома о высшем образовании без риска
Как получить диплом техникума официально и без лишних проблем
Купить диплом магистра оказалось возможно, быстрое обучение и диплом на руки
онлайн казино с депозитом
игровые автоматы играть бесплатно и без регистрации
Всё, что нужно знать о покупке аттестата о среднем образовании
Как не попасть впросак при покупке диплома колледжа или ПТУ в России
vip.rolevaya.info/viewtopic.php?id=5566#p9619
Парадокс, но купить диплом кандидата наук оказалось не так и сложно
behealthy.maxbb.ru/posting.php?mode=post&f=8
Как официально купить диплом вуза с упрощенным обучением в Москве
Привет!
Мы готовы предложить документы ВУЗов
[url=http://veneraroleplay.listbb.ru/viewtopic.php?f=4&t=463/]veneraroleplay.listbb.ru/viewtopic.php?f=4&t=463[/url]
Полезная информация как официально купить диплом о высшем образовании
dimsan.blogspot.com/2009/06/user-profile-hive-cleanup-service?m=1
http://cupe.pro/bitrix/redirect.php?goto=https://t.me/s/bonus_za_registratsiyu_bez_depa
Купить диплом о среднем полном образовании, в чем подвох и как избежать обмана?
Приобретение диплома ПТУ с сокращенной программой обучения в Москве
На сегодняшний день для развития бизнеса в интернете недостаточно просто создать сайт. Важно, чтобы он был в топе в поисковых системах. Digital агентство ПАРОВОЗ уже более десятилетия профессионально занимается [url=https://seo18.su/]сайты с продвижением товаров и услуг[/url] гарантируя компаниям постоянный поток клиентов. Мы предлагаем тестовый период, в рамках которого вы сможете оценить качество наших услуг и ощутить результаты на практике.
Секрет нашего успеха — это внедрение передовых технологий и высокий профессионализм. Мы не просто увеличиваем посещаемость, а разрабатываем [url=https://seo18.su/]что входит в seo оптимизацию[/url] которое включает в себя наращивание ссылочной массы, накрутку поведенческих факторов и подъем позиций в топе Гугл и Яндексе. Если вы хотите, чтобы ваш сайт приносил прибыль, начинайте действовать — с каждым днем рынок становится плотнее.
Официальная покупка диплома вуза с сокращенной программой в Москве
+ for the post
_________________
[URL=https://rucasino.domainrenewal.site]slot casino бездепозитный бонус[/URL]
онлайн казино с депозитом
демо казино слоты
Можно ли купить аттестат о среднем образовании? Основные рекомендации
deti-journal.ru/kupit-diplomi-o-visshem-obrazovanii-bistro-i-nadezhno
Купить диплом старого образца, можно ли это сделать по быстрой схеме?
[url=https://finance-global.org/menedzhment/vnedrenie-izmenenij-v-kompanii-po-modeli-kottera/]
The One Stock Everyone Should Be Watching Right Now!
[/url]
anatoliyrud.ekafe.ru/viewtopic.php?f=29&t=3176
Идеи для изготовления креативных квадратных номеров, Топовые способы сделать номер квадратной формы
Процесс получения диплома стоматолога: реально ли это сделать быстро?
[url=https://mikro-zaim-online.ru/]лучшие микрозаймы онлайн на карту без отказа[/url]
Официальная покупка диплома вуза с сокращенной программой в Москве
bookmarkbirth.com/story17114600/получу-диплом-песня
Что делать, чтобы не попасть в беду с одинаковыми номерами авто, советы для автолюбителей.
Почему дубликаты номеров автомобилей – это проблема, информируем целиком и деталями.
Какие штрафы ждут владельцев авто с дубликатными номерами, подробный анализ в одном материале.
Как защитить свое авто от ситуации с одинаковыми госномерами, практические советы для всех автовладельцев.
Что делать, если вы столкнулись с дубликатами госномеров, подробности от экспертов.
Рекомендации по защите своего автомобиля от ситуации с дубликатами, тонкости, о которых нужно знать каждому автовладельцу.
Как правильно реагировать на наличие дубликатов номеров на вашем транспортном средстве, полезная информация для всех владельцев машин.
изготовление гос номеров [url=https://www.dublikatznakov77.ru/]https://www.dublikatznakov77.ru/[/url] .
Купить диплом о среднем образовании в Москве и любом другом городе
Вопросы и ответы: можно ли быстро купить диплом старого образца?
moneywang.com/4306/comment-page-30#comment-2495443
[url=https://bankovskaya-garantiya-24.ru/]https://bankovskaya-garantiya-24.ru/[/url] – как банковская гарантия поможет сохранить ликвидность вашего бизнеса и обеспечить исполнение финансовых обязательств перед партнерами и клиентами.
Как приобрести диплом техникума с минимальными рисками
gamesmaker.ru/forum/common/offtopics/#replyform
Актуальное зеркало казино [url=https://vavadafhl.xyz/]вавада на сегодняшний день[/url]: как найти и использовать дублирующий сайт для доступа к игровым слотам и бонусам.
Yo, what’s good, my guy? I’m so pumped we got to chill, dawg.
It seems that this could significantly enhance your project Ferrous material disposal regulations
Goodbye for now, and may serenity guide your steps
Полезные советы по безопасной покупке диплома о высшем образовании
При приближении к мегакурорту который стоит 5 млдр. $, строющегося недалеко от побережья Дубая, по истине восторгает его масштабность.
Создававшийся уже два десятилетия, “Сердце Европы” представляет собой лишь одну из частей света – огромной коллекции искусственных островов в форме атласа, но после завершения проекта он будет его роскошным центральным компонентом.
Во время эпидемии он может оказаться великолепным творением в своем стремлении воссоздать европейский континент для избранных туристов, которые не хотят путишествовать.
На фоне всемирного стихания туризма, в связи с Ковидом, он также являет большой рывок веры в долгосрочную перспективу страны.
После поездки длиной в 4 км на по воде от материка, триста созданных руками человека островов мира поднимаются из Персидского залива, как перевернутые площадки для гольфа.
Большая их часть стоят безлюдными с начала старта этого мероприятия с 2003-го года, а последующие мировые финансовые скачки препятствовали прогрессу строительства.
И вот перед нами предстает “HoE”.
Больше пятнадцати роскошных гостиниц, домов отдыха и особняков олигархов уже готовы или строятся на этих водных участках. Источник новостей [url=https://ligalitolko.site/catalogue/view/99.html]ligalitolko.site[/url]
stromectol pret [url=https://essaydw.com/]stromectol canadian pharmacy[/url] buy stromectol for humans in tijuana
stromectol bulgaria https://essaydw.com/ – stromectol tablets 3mg
[url=https://vavadafim.com/]vavada casino store[/url] – все о доступных играх, бонусах и акциях в казино Вавада, а также советы от опытных игроков и экспертов.
Диплом техникума купить официально с упрощенным обучением в Москве
Как не стать жертвой мошенников при покупке диплома о среднем полном образовании
forumkk.listbb.ru/viewtopic.php?f=12&t=611
Сколько стоит диплом высшего и среднего образования и как его получить?
Вопросы и ответы: можно ли быстро купить диплом старого образца?
Новая статья раскрывает особенности игры [url=https://vavadatok.com/]вавада[/url] на деньги и дает полезные советы для успешной игры и выигрыша.
https://salon-elos.ru/igrovoy-avtomati-na-dengi/betunlim-casino-promokod.php Betunlim casino РїСЂРѕРјРѕРєРѕРґ
http://winone1.ru/fonbet-vhod-lichniy-kabinet/pin-ap-bukmekerskaya-skachat-prilozhenie.php
http://lipa-fv.ru/promokod-na-1xbet/kakie-est-bukmekerskie-kontori-onlayn.php
http://knigarulit.ru/igrat-besplatno-v-igrovie-avtomati/syka-casino-promokod.php Syka casino РїСЂРѕРјРѕРєРѕРґ
http://salon-elos.ru/mines-are/igrovie-avtomati-svini-igrat-besplatno.php
http://winone1.ru/fribet-bukmekerskaya-kontora/aviator-pin-up.php
http://bonus-vsem01.ru/vse-igrovie-avtomati-besplatnie/bonanza-lileynik-opisanie.php
http://lipa-fv.ru/igrat-besplatno-slot-avtomati/kak-potratit-bonusniy-schet-v-1xbet.php
https://salon-elos.ru/prilozhenie-skachat-fonbet-na/fonbet-bukmekerskaya-kontora-fribet-za-registratsiyu.php Фонбет букмекерская контора фрибет за регистрацию
http://salon-elos.ru/igrovie-avtomati-besplatnie-igri-bez-registratsii/stariy-slot.php
http://bonus-vsem01.ru/1xbet-zerkala-rabochie-na-segodnya/reklama-azino777-vitya-ak.php
http://winone1.ru/igrovie-avtomati-igrat-besplatno-5000/fonbet-fribet-2000r.php
http://lipa-fv.ru/igrat-avtomati-besplatno-slot/igrovie-avtomati-loto-ru.php Ргровые автоматы loto ru
http://bonus-vsem01.ru/vulkani-igrovie-avtomati-igrat-besplatno/1xbet-alternativniy-vhod-na-sayt.php
http://knigarulit.ru/1-win-sayt/telegramm-stavki-na-hokkey.php
http://salon-elos.ru/igrovoy-avtomat-besplatno-bez-registratsii-demo/pin-ap-zerkalo-rabochee-na-segodnya.php
http://bonus-vsem01.ru/bukmekerskaya-kontora-skachat-na-android/bukmekerskie-kontori-v-internete.php
http://salon-elos.ru/1win-zerkalo-segodnya/otzivi-o-fonbet.php
http://lipa-fv.ru/fribeti-bukmekerskie-kontori/igrovie-avtomati-reyting-po-otdache.php
http://bonus-vsem01.ru/mostbet-skachat/fonbet-vhod-polnaya-versiya.php
http://salon-elos.ru/telefon-1xbet/udalit-akkaunt-fonbet-navsegda-kak-s-telefona.php
http://winone1.ru/mobilniy-sayt-azino777/slot-avtomati-bez-registratsii-igrat-besplatno.php
http://salon-elos.ru/igrovie-avtomati-besplatnie-igri-bez-registratsii/stavki-na-sport-germaniya.php
http://salon-elos.ru/casino-x-sayt/populyarnie-igrovie-avtomati-na-dengi-onlayn.php
http://lipa-fv.ru/igrat-besplatno-slot-avtomati/polnaya-versiya-sayta-1-xbet.php
be8d8d3
http://avtofreon.ru/remont-jelektromufty/img_20200817_081141-1/?unapproved=147834&moderation-hash=92915ea34779fdcb76cc75b6d9abdf12#comment-147834
https://jibun-blog.com/2020/01/11/blog/?unapproved=58086&moderation-hash=0e7496bf02f4790e26e2616376f45cde#comment-58086
https://www.lydiabeckercoaching.de/2019/04/02/24-werde-deiner-macht-bewusst/75136
http://uenews.ru/exclusive/79002-sozdan-pervyy-v-mire-pk-razmerom-s-kompyuternuyu-mysh.html
http://lancier.ru/stroenie-glaz/kak-opredelit-tochno-svoj-cvet.html
http://ruseti.ru/seti/422.htm
http://steampunker.ru/blog/18233.html
http://toyfaq.ru/alltoy/consolstaff/consdrug/342-igra-toy-commander-dreamcast.html
https://www.geniustags.com/product/mango-womens-bag/?unapproved=448899&moderation-hash=e0e0fd4b04f07e5c23e28f998d2ddf12448899
https://www.paveltlapak.cz/cookies-2022/19806
https://pizda-rasskazy.site/otkrytie-magazina-4/30621
Всё о покупке аттестата о среднем образовании: полезные советы
Сколько стоит получить диплом высшего и среднего образования легально?
Диплом вуза купить официально с упрощенным обучением в Москве
Купить диплом магистра оказалось возможно, быстрое обучение и диплом на руки
topnewsgadget.ru/vash-diplom-nasha-zabota-o-vashem-budushhem
Полезные советы по безопасной покупке диплома о высшем образовании
funnywipes.maxbb.ru/viewtopic.php?f=3&t=829
Всё, что нужно знать о покупке аттестата о среднем образовании без рисков
Диплом техникума купить официально с упрощенным обучением в Москве
Привет, друзья!
Мы можем предложить документы техникумов
[url=http://productinn.mn.co/posts/67852089/]productinn.mn.co/posts/67852089[/url]
Круто, я искала этот давно
_________________
[url=https://slo.hotkazino.space]вакансии спб букмекер[/url]
Как правильно приобрести диплом колледжа или ПТУ в России, важные моменты
Как правильно купить диплом колледжа и пту в России, подводные камни
Купить диплом о среднем образовании в Москве и любом другом городе
terralogie.com/?p=6591&lang=en
Возможно ли купить диплом стоматолога, и как это происходит
Привет!
Мы можем предложить документы ВУЗов
[url=http://selkovo.rolka.me/viewtopic.php?id=4054#p11785/]selkovo.rolka.me/viewtopic.php?id=4054#p11785[/url]
Как правильно купить диплом колледжа и пту в России, подводные камни
Как официально приобрести аттестат 11 класса с минимальными затратами времени
Можно ли купить аттестат о среднем образовании? Основные рекомендации
infiniterealities.listbb.ru/viewtopic.php?f=7&t=462
Купить диплом старого образца, можно ли это сделать по быстрой схеме?
Пошаговая инструкция по безопасной покупке диплома о высшем образовании
nutriciolog.ru/poluchite-diplom-bystro-i-bez-lishnix-xlopot
Приобретение школьного аттестата с официальным упрощенным обучением в Москве
Купить диплом о среднем образовании в Москве и любом другом городе
Процесс получения диплома стоматолога: реально ли это сделать быстро?
budemir.ru/kupit-diplom-magistra-nedorogo-i-bez-riska
Как оказалось, купить диплом кандидата наук не так уж и сложно
Покупка диплома о среднем полном образовании: как избежать мошенничества?
tawasol1.mn.co/spaces/9349784/feed
Возможно ли купить диплом стоматолога, и как это происходит
Вопросы и ответы: можно ли быстро купить диплом старого образца?
Реально ли приобрести диплом стоматолога? Основные этапы
Парадокс, но купить диплом кандидата наук оказалось не так и сложно
prema-diploms.ru
Как приобрести аттестат о среднем образовании в Москве и других городах
Узнайте, как приобрести диплом о высшем образовании без рисков
Нужна отделка и покраска деревянного дома? Наша бригада из опытных строителей из Белоруссии готова воплотить ваши идеи в реальность! Современные технологии, индивидуальный подход, и качество – это наши гарантии. Посетите наш сайт [url=https://otdelka-fasada-i-pokraska-doma-pr1.ru]по отделке и покраске дома[/url] и начните строительство вашего уюта прямо сейчас! #БелорусскаяБригада #отделка дома #покраска дома
Как получить диплом стоматолога быстро и официально
Как приобрести диплом о среднем образовании в Москве и других городах
Процесс получения диплома стоматолога: реально ли это сделать быстро?
sustalks.com/blogs/8366/Ваш-диплом-без-лишних-ожиданий
Официальное получение диплома техникума с упрощенным обучением в Москве
Здравствуйте!
Вы слышали когда-нибудь о X GPT Writer, X Parser light или X Translator?
Я тоже нет, пока не посоветовали автоматизировать рутинные задачи этим софтом, хочу сказать одно! Я потом долго не мог поверить,
что можно так автоматизировать рутинные задачи и главное получить на выходе 100% свежий контент, что будут на ура индексировать
поисковые системы)
X-GPTWriter контент генератор
Не знаете, как создать уникальный контент? X-GPTWriter — это контент-генератор, который поможет вам с легкостью решить эту задачу. Просто введите тему, и X-GPTWriter сгенерирует текст, который будет актуален и интересен вашей аудитории. Используйте его для создания постов в социальных сетях, статей и описаний товаров. С X-GPTWriter контент больше не будет для вас проблемой!
X-GPTWriter генератор контента для видео
С X-GPTWriter вы сможете легко создавать сценарии и текст для ваших видео. Этот генератор контента для видео поможет вам разработать уникальные идеи и интересные сюжеты. Используйте X-GPTWriter для оптимизации своего процесса создания видео и повышения качества контента. Его простота и функциональность делают X-GPTWriter идеальным помощником для авторов. Начните использовать X-GPTWriter для видео уже сегодня и привлекайте больше зрителей!
Кстати, друг дал купон на скидку 40%: 94EB516BCF484B27
подробности где его вводить указаны на сайте: http://www.xtranslator.ru/x-gpt-writer
Стоит попробовать Друзья, там есть демо, все бесплатно, не пожалеете)
X-Translator контент идея генератор
генератор контента на русском языке
X-Parser Light парсер контента интернет магазина
генератор контента онлайн
X-GPTWriter человеки генератор контента
скачать уникализатор текстов X-Translator
уникализатор текста лучший X-GPTWriter
лучший генератор контента
генератор уникального контента
Уникальный контент с синонимизатором на базе ChatGPT
[url=https://www.xtranslator.ru/x-parser-light/]бесплатные парсеры контента[/url]
Удачи!
Привет всем!
Вы слышали когда-нибудь о X GPT Writer, X Parser light или X Translator?
Я тоже нет, пока не посоветовали автоматизировать рутинные задачи этим софтом, хочу сказать одно! Я потом долго не мог поверить,
что можно так автоматизировать рутинные задачи и главное получить на выходе 100% свежий контент, что будут на ура индексировать
поисковые системы)
X-GPTWriter генератор контент плана онлайн бесплатно
Создавайте контент-планы легко и бесплатно с X-GPTWriter! Этот генератор контент-плана поможет вам организовать ваши идеи и следить за публикациями. Вы сможете легко планировать контент на месяцы вперед, что существенно повысит вашу продуктивность. С X-GPTWriter вы всегда будете в курсе, какой контент вам нужно подготовить. Начните с X-GPTWriter и сделайте ваш контент более структурированным!
X-GPTWriter бесплатный генератор контента
Ищете бесплатный генератор контента? X-GPTWriter предлагает вам уникальный инструмент для создания текстов без каких-либо затрат. Вы можете генерировать статьи, посты и описания на любые темы быстро и легко. С X-GPTWriter ваш контент будет качественным и интересным для аудитории. Попробуйте бесплатный генератор контента с X-GPTWriter уже сегодня!
Кстати, друг дал купон на скидку 40%: 94EB516BCF484B27
подробности где его вводить указаны на сайте: http://xtranslator.ru/
Стоит попробовать Друзья, там есть демо, все бесплатно, не пожалеете)
X-GPTWriter сайты генераторы контента
X-Translator бесплатный генератор контента
онлайн генератор контента
X-Parser Light парсер контента вконтакте
Легкий способ создавать контент с X-GPTWriter
X-GPTWriter генератор контента для видео
X-Translator генераторы контента для сайта
X-Translator генератор рич контента для озон
универсальные парсеры контента
уникализатор текста онлайн бесплатно X-Translator
[url=http://www.xtranslator.ru]X-Translator контент для сайта генератор[/url]
Удачи!
Реально ли приобрести диплом стоматолога? Основные этапы
mihail.ekafe.ru/viewforum.php?f=1
Вопросы и ответы: можно ли быстро купить диплом старого образца?
Процесс получения диплома стоматолога: реально ли это сделать быстро?
girlscools.ru/kupit-diplom-vash-start-dlya-karernogo-rosta
Узнайте стоимость диплома высшего и среднего образования и процесс получения
Как купить аттестат 11 класса с официальным упрощенным обучением в Москве
Диплом вуза купить официально с упрощенным обучением в Москве
socialbuzztoday.com/story2640813/получить-диплом-матроса
Как купить диплом о высшем образовании с минимальными рисками
8saksx-diploms.ru
[url=https=https://uggs-russia.com/]Купить UGG Australia оригинал в Москве[/url] можно у нас – официальный интернет-магазин.
Как оказалось, купить диплом кандидата наук не так уж и сложно
Сколько стоит диплом высшего и среднего образования и как его получить?
madesocials.com/story2427694/купить-диплом-Тюмень
Быстрое обучение и получение диплома магистра – возможно ли это?
opaseke.com/users/7219
Привет всем!
Вы слышали когда-нибудь о X GPT Writer, X Parser light или X Translator?
Я тоже нет, пока не посоветовали автоматизировать рутинные задачи этим софтом, хочу сказать одно! Я потом долго не мог поверить,
что можно так автоматизировать рутинные задачи и главное получить на выходе 100% свежий контент, что будут на ура индексировать
поисковые системы)
X-GPTWriter генератор контента на русском языке
X-GPTWriter — это мощный генератор контента на русском языке, который поможет вам создавать уникальные и интересные тексты. Этот инструмент идеально подходит для блогеров, маркетологов и предпринимателей, желающих улучшить качество своего контента. С X-GPTWriter вы сможете быстро генерировать статьи, посты и описания, которые будут захватывать внимание вашей аудитории. Используйте его для создания материалов, соответствующих вашим требованиям и ожиданиям. Начните создавать уникальный контент на русском с помощью X-GPTWriter уже сегодня!
X-GPTWriter генератор контента инстаграм
С X-GPTWriter создание контента для Instagram стало проще и увлекательнее! Этот генератор поможет вам быстро генерировать посты, которые будут привлекать внимание ваших подписчиков. Используйте X-GPTWriter для создания ярких и оригинальных описаний и хештегов. Благодаря этому инструменту ваш профиль будет всегда актуальным и интересным. Попробуйте X-GPTWriter для вашего Instagram уже сегодня!
Кстати, друг дал купон на скидку 40%: 94EB516BCF484B27
подробности где его вводить указаны на сайте: http://xtranslator.ru/x-parser-light/
Стоит попробовать Друзья, там есть демо, все бесплатно, не пожалеете)
рич контент генератор
X-Translator генератор контента онлайн
контент генераторы
X-Translator генератор контент плана онлайн бесплатно
X-Parser Light php парсер контента
X-GPTWriter генератор для уникального контента
генератор контента для социальных сетей
X-Parser Light парсер контента sjs
X-Translator уникальный контент генератор
контент генератор
[url=https://www.xtranslator.ru/x-parser-light/]X-Parser Light парсер контента вконтакте[/url]
Удачи!
Полезная информация как официально купить диплом о высшем образовании
saksx-diploms24.ru
Как получить диплом техникума с упрощенным обучением в Москве официально
Процесс получения диплома стоматолога: реально ли это сделать быстро?
fishing.ukrbb.net/posting.php?mode=post&f=21&sid=0dac82fd73d57e1319286cd0b3247972
Парадокс, но купить диплом кандидата наук оказалось не так и сложно
Приобретение диплома ПТУ с сокращенной программой обучения в Москве
Как приобрести аттестат о среднем образовании в Москве и других городах
deanonnic.listbb.ru/viewtopic.php?f=5&t=546
Диплом пту купить официально с упрощенным обучением в Москве
Парадокс, но купить диплом кандидата наук оказалось не так и сложно
Сколько стоит диплом высшего и среднего образования и как его получить?
igenplan.ru/forum/user/94899
Стоимость дипломов высшего и среднего образования и как избежать подделок
russa-diploms.ru
Приобретение школьного аттестата с официальным упрощенным обучением в Москве
paladiny.ru/forummess.dwar.php?TopicID=28121
Как приобрести диплом техникума с минимальными рисками
Как безопасно купить диплом колледжа или ПТУ в России, что важно знать
productinn.mn.co/posts/pogruzhenie-v-mir-igrovyh-avtomatov-gizbo-casino
Можно ли быстро купить диплом старого образца и в чем подвох?
telegra.ph/medosmotrdlyapostupleniyavvuz20200724
Покупка школьного аттестата с упрощенной программой: что важно знать
godoor.ru
Полезные советы по покупке диплома о высшем образовании без риска
Пошаговая инструкция по официальной покупке диплома о высшем образовании
kai87la.flybb.ru/viewtopic.php?f=5&t=651
Как получить диплом техникума с упрощенным обучением в Москве официально
+ for the post
_________________
[url=https://igra.best-casino-play.rent]слоты для ламп[/url]
Всё, что нужно знать о покупке аттестата о среднем образовании без рисков
Стоимость дипломов высшего и среднего образования и как избежать подделок
Узнайте, как безопасно купить диплом о высшем образовании
telegra.ph/dlyapostupleniyavvuzdokumenty0724
Пошаговая инструкция по официальной покупке диплома о высшем образовании
Как получить диплом техникума с упрощенным обучением в Москве официально
nimstradingltd.com/2024/08/14/kupit-diplom-903620mzok
[b]Celebrate the setting of phobia and festivity on the haunted night![/b]
And make certain not to miss the possibility to revamp your togs and house at a special offer,
as today we have promotional discounts on all commodities in honor of the holiday occasion.
Reward yourself to the adult version of [b]Halloween temptations[/b] with our cut prices.
.:re:. : https://gurudodesconto.info
[url=https://gurudodesconto.info]Coupons for Halloween[/url]
Сколько стоит диплом высшего и среднего образования и как это происходит?
enfogentraining.com/blog/index.php?entryid=95208
[url=] https://bento.me/zimorodok-79-seriya-onlajn-novaya-seriya-na-russkom-yazyke-18102024
https://bento.me/pryamoj-efir-zimorodok-79-seriya-s-russkoj-ozvuchkoj-premera-18102024
https://bento.me/tureckij-serial-zimorodok-79-seriya-smotret-onlajn-18-oktyabrya-2024-gjxmc
https://bento.me/poslednyaya-seriya-zimorodok-79-na-russkom-ot-18-oktyabrya-2024
https://bento.me/zimorodok-3-sezon-6-seriya-data-vyhoda-18-oktyabrya-2024
https://bento.me/smotrite-zimorodok-79-seriya-data-vyhoda-18102024-na-russkom
https://bento.me/tureckij-serial-zimorodok-pryamaya-translyaciya-79-serii-18-oktyabrya-2024
https://bento.me/zimorodok-79-seriya-18-oktyabrya-2024-goda-russkaya-versiya-onlajn
https://bento.me/zimorodok-79-seriya-novaya-seriya-18-oktyabrya-na-russkom
https://bento.me/onlajn-translyaciya-zimorodok-79-seriya-18102024-s-russkoj-ozvuchkoj
https://bento.me/russkaya-versiya-zimorodok-79-seriya-premera-18-oktyabrya-2024-goda
https://bento.me/novyj-epizod-zimorodok-79-seriya-russkaya-ozvuchka-ot-18-oktyabrya-2024
https://bento.me/tureckij-serial-zimorodok-79-seriya-smotret-onlajn-s-18102024
https://bento.me/zimorodok-79-seriya-russkaya-ozvuchka-18-oktyabrya-2024-onlajn%5B/url%5D
Как получить диплом стоматолога быстро и официально
prema365-diploms.ru
[url=https://ludobro.ru/reviews/booi/]скачать казино онлайн с выводом денег[/url]
https://ludobro.ru/reviews/leebet/
https://ludobro.ru/reviews/shampion-slots/
https://ludobro.ru/reviews/shampion-slots/
[url=https://ludobro.ru/reviews/riobet/]в какие автоматы реально выиграть деньги отзывы[/url]
https://ludobro.ru/reviews/casino-x/
https://ludobro.ru/reviews/vavada/
https://ludobro.ru/reviews/gold/
https://ludobro.ru/reviews/gama/
Официальная покупка школьного аттестата с упрощенным обучением в Москве
http://metalltag.ru/bitrix/redirect.php?goto=https://t.me/s/bonus_za_registratsiyu_bez_depa
interesting news
_________________
[URL=https://igra.top10casinossites.lat]онлайн казино игра[/URL]
[url=https://old-tverlib.ru/1win-igrovie-avtomati.html]1win игровые автоматы[/url]
О бонусах: “Щедрая бонусная система! Особенно порадовал приветственный бонус и акции для постоянных игроков. Часто провожу время в казино и на ставках.”
Как официально приобрести аттестат 11 класса с минимальными затратами времени
arusak-diploms.ru
Полезная информация как купить диплом о высшем образовании без рисков
Plan1&1
http://suncheon.info/bbs/board.php?bo_table=free&wr_id=31671
Быстрое обучение и получение диплома магистра – возможно ли это?
interesting news
_________________
[URL=https://igra.bestcasinossites.shop]отзывы об отеле amwaj oyoun resort casino[/URL]
Приобретение школьного аттестата с официальным упрощенным обучением в Москве
[url=https://bento.me/zimorodok-79-seriya-onlajn-novaya-seriya-na-russkom-yazyke-18102024]https://bento.me/pacanki-9-sezon-6-seriya-24102024-smotret-onlajn
https://bento.me/pacanki-9-sezon-6-seriya-24102024-smotret-onlajn-bhojze
https://bento.me/pacanki-6-vypusk-smotret-onlajn-v-hd-kachestve-24-oktyabrya-2024
https://bento.me/pyatnica-pacanki-ostrov-poslednij-vypusk-efir-ot-24-oktyabrya-2024
https://bento.me/pacanki-9-sezon-6-vypusk-or-novye-pacanki-or-2024-24102024
https://bento.me/kanal-pyatnica-stoit-li-smotret-onlajn-krajnij-6-vypusk-shou-pacanki-ot-24102024
https://bento.me/prmoj-efir-novye-pacanki-novaya-seriya-6-ot-24-10-2024
https://bento.me/primaya-translyaciya-shou-pacanok-ot-24102024-6-seriya
https://bento.me/kanal-pyatnica-zapis-novogo-vypuska-shou-pacanki-ostrov-6-seriya-ot-24-oktyabrya-2024-20-00-smotret-onlajn
https://bento.me/pacanki-6-seriya-24-oktyabrya
https://bento.me/pacanki-9-sezon-6-seriya-smotret-onlajn-novyj-vypusk-24102024
https://bento.me/pacanki-9-sezon-6-seriya-onlajn-translyaciya-i-zapis-efira-24-oktyabrya-2024
https://bento.me/novaya-seriya-pacanki-9-sezon-6-vypusk-pryamoj-efir-ot-24-oktyabrya-2024
https://bento.me/smotret-onlajn-pacanki-9-sezon-6-vypusk-efir-na-kanale-pyatnica-24102024
https://bento.me/pacanki-9-sezon-6-seriya-chto-proizoshlo-v-vypuske-ot-24-oktyabrya-2024
https://bento.me/novyj-epizod-shou-pacanki-9-sezon-6-seriya-v-horoshem-kachestve-24102024
https://bento.me/pacanki-9-sezon-6-seriya-smotret-onlajn-besplatno-na-pyatnice-24-oktyabrya-2024
https://bento.me/pacanki-9-sezon-6-seriya-efir-na-pyatnice-24102024-pryamaya-translyaciya
https://bento.me/pacanki-9-sezon-6-vypusk-segodnya-vecherom-smotret-besplatno-24-oktyabrya-2024
https://bento.me/pacanki-9-sezon-polnyj-6-vypusk-efir-24102024-onlajn-v-hd
https://wokwi.com/projects/412485508100938753
https://herbalmeds-forum.biolife.com.my/d/199167-httpswokwicomprojects412485508100938753
https://yellowpagesclassifiedads.com/post/njauso
https://bento.me/onlajn-translyaciya-zimorodok-79-seriya-18102024-s-russkoj-ozvuchkoj
https://bento.me/russkaya-versiya-zimorodok-79-seriya-premera-18-oktyabrya-2024-goda
https://bento.me/novyj-epizod-zimorodok-79-seriya-russkaya-ozvuchka-ot-18-oktyabrya-2024
https://bento.me/tureckij-serial-zimorodok-79-seriya-smotret-onlajn-s-18102024
https://bento.me/zimorodok-79-seriya-russkaya-ozvuchka-18-oktyabrya-2024-onlajn%5B/url%5D%5B/url%5D
Быстрая схема покупки диплома старого образца: что важно знать?
arusak-diploms.ru
[url=https://i.postimg.cc/YqQPnw4g/2.jpg][img]https://i.postimg.cc/9XnNbYc6/03.jpg
[/img][/url]
ЗАРАБАТЫВАЙТЕ HOT Wallet, ИГРАЯ В ИГРУ!
Хочешь крипту без вложений? Всё через Telegram!
Приглашай друзей и получай 20% в HOT от них и 5% от их друзей.
Присоединяйся к нашей Telegram-группе “Деревня” по ссылке: https://bit.ly/48lAs6P и получи +5% к монетам!
В группе полное описание — всё просто и понятно!
ПОЛНАЯ ИНСТРУКЦИЯ ПО РЕГИСТРАЦИИ И ЗАРАБОТКУ МОНЕТЫ HOT ОТ NEAR
Всё, что нужно знать о покупке аттестата о среднем образовании без рисков
играть в Sakura Fortune
игровой автомат Sakura Fortune
крейзи тайм
крейзи тайм статистика сегодня
крейзи тайм
крейзи тайм на деньги
[url=https=https://stmb-trucks.ru/]Спецтехника мировых брендов[/url], предлагаемая компанией ООО «СТМБ», — это оборудование, проверенное временем и многими клиентами по всему миру. Мы предлагаем технику ведущих производителей, которая гарантирует надёжную и бесперебойную работу в любых условиях. В сочетании с нашим сервисом и поддержкой, ваша спецтехника всегда будет готова к выполнению самых сложных задач.
Ciao ai romantici e agli amanti del casino!
Winnita si dimostrato un casino online di fiducia che mette al centro dell’attenzione il divertimento dei suoi giocatori. Con una vasta gamma di giochi di alta qualit, promozioni vantaggiose e un ambiente sicuro, facile capire perch sempre pi giocatori italiani scelgono Winnita. Sia che tu sia un principiante o un giocatore esperto, questa piattaforma ha tutto cio di cui hai bisogno per vivere un’esperienza di gioco entusiasmante e gratificante.
Winnita Casino Italia: An In-Depth Review
Winnita Casino Italia has quickly gained popularity among online gaming enthusiasts, offering a unique blend of excitement, security, and a vast selection of games. With its user-friendly interface and generous promotions, Winnita stands out in the competitive landscape of online casinos. This review will explore the key features, game offerings, bonuses, and overall experience of playing at Winnita Casino Italia.
Key Features of Winnita Casino
1. License and Security
Winnita Casino operates under a reputable gaming license, ensuring that it adheres to strict regulations and standards for fair play. The platform utilizes advanced encryption technology to protect players’ personal and financial information, giving players peace of mind while they enjoy their gaming experience.
2. Game Variety
One of the main attractions of Winnita Casino is its extensive game library. Players can choose from a wide range of options, including:
Slot Machines: From classic fruit slots to modern video slots, Winnita offers a diverse selection that caters to all preferences. Players can enjoy thrilling themes and engaging gameplay, with many games featuring exciting bonus rounds and jackpots.
Table Games: Fans of traditional casino games will find a solid variety at Winnita, including classics like blackjack, roulette, baccarat, and poker. Each game comes with different variations to suit various playing styles and strategies.
Live Casino: For those seeking an authentic casino experience, the live dealer section allows players to interact with professional croupiers in real-time. This feature adds an extra layer of excitement, as players can enjoy games like live blackjack, live roulette, and more from the comfort of their homes.
Bonuses and Promotions
Il link al Casino e qui – https://xinzhu.eu
winnita Official
crazy time uscite
giochi slot gratis da scaricare
fowl play gold 4 download gratis android
[url=https://xinzhu.eu/]tabella carte coin master[/url]
Buoni compensi!
свит бонанза демо
свит бонанза
Приобретение школьного аттестата с официальным упрощенным обучением в Москве
2orik-diploms.ru
Новые МФО предлагают выгодные условия: займ до 30 000 рублей под 0.8%, без лишних проверок и ожидания.
https://maps.google.com.eg/url?q=https://t.me/s/zaim_bez_otkazov_na_kartu
https://cse.google.mv/url?q=https://t.me/s/zaim_bez_otkazov_na_kartu
http://maps.google.at/url?q=https://t.me/s/zaim_bez_otkazov_na_kartu
https://cse.google.cf/url?sa=i&url=https://t.me/s/zaim_bez_otkazov_na_kartu
http://cse.google.co.jp/url?sa=i&url=https://t.me/s/zaim_bez_otkazov_na_kartu
https://clients1.google.dz/url?q=https://t.me/s/zaim_bez_otkazov_na_kartu
[url=https://888starz.bet/]child pussy[/url]
aviator играaviator на деньгиaviator играть на деньгислот aviator на деньгиaviator money game
aviator играaviator на деньгиaviator играть на деньгислот aviator на деньгиaviator money game
aviator играaviator на деньгиaviator играть на деньгислот aviator на деньгиaviator money game
aviator играaviator на деньгиaviator играть на деньгислот aviator на деньгиaviator money game
aviator играaviator на деньгиaviator играть на деньгислот aviator на деньгиaviator money game
aviator играaviator на деньгиaviator играть на деньгислот aviator на деньгиaviator money game
aviator играaviator на деньгиaviator играть на деньгислот aviator на деньгиaviator money game
aviator играaviator на деньгиaviator играть на деньгислот aviator на деньгиaviator money game
На сайте [url=https://mostbet-wn3.xyz/]мостбет casino[/url] вы найдете рабочее зеркало для доступа к играм и азартным развлечениям без блокировок и ограничений.
MEGA зеркало!!!
Подробнее https://teletype.in/@bitwa/s6yVw8PjoID
https://mg2at.hair
https://t.me/megaweb_ref
m3ga at, m3ga gl, m3ga fl, https m3ga at, m3ga ссылка, m3ga at зеркало, http m3ga gl, сайт m3ga, m3ga gl зеркало, m3ga hl, mega m3ga, m3ga sb, http m3ga at, m3ga com, официальный сайт m3ga, m3ga at не работает, m3ga kz, m3ga вход, m3ga ay, m3ga gl ссылка, m3ga hn, m3ga как зайти, m3ga зеркало сайт, m3ga gd, m3ga fo, m3ga gl сайт, m3ga at ссылка mega, mega m3ga gl, m3ga lg, https m3ga gl, m3ga at зеркало официальный, aurosonic mully shvman m3ga moving mountains, m3ga gl официальный, https m3ga at https m3ga at ссылка, m3ga gl com, megaweb4 at m3ga at mega gd, m3ga at зеркало официальный сайт, m3ga sb info, https m3ga at вход, m3ga gl не работает, m3ga ta, m3ga gl ссылка mega, m3ga g, m3ga gl mega555darknetsbfomarket com, m3ga gl вход, m3ga gl официальный сайт, http m3ga gl mega555darknetsbfomarket com, m3ga fn, https m3ga at ссылка mega, https m3ga com, m3ga 555, m3ga at1, m3ga jl, m3ga at megarcopen org, m3ga gll, m3ga go, m3ga ot, m3ga lv, m3ga mbot, m3ga onion, рабочие зеркала m3ga, m3ga gl megarcopen org, m3ga moriarty, m3ga web, m3ga hh, m3ga nt, m3ga ru, m3ga tg, m3ga hair, m3ga даркнет как зайти, m3ga мориарти, moving mountains feat m3ga, http m3ga at не работает, m3ga fо, m3ga официальные ссылки, m3ga рабочая ссылка, приложение m3ga, http m3ga gl ссылка, https m3ga to login index e, m3ga f, m3ga tor, m3ga актуальные ссылки, m3ga gi, m3ga gl грибы, m3ga gl зеркало megadarknet click, m3ga top com, megadarknet click зеркало m3ga, m3ga shop, www m3ga, http m3ga gl не работает, m3ga 4at, m3ga dl, m3ga fo megarcopen org, m3ga gk, m3ga gl m3ga at вход акт ссылка, m3ga t, m3ga ti, m3ga web4, mega http m3ga gl, https mega hn https m3ga gl, m3ga ap, m3ga atr, m3ga bl, m3ga da, m3ga nh, m3ga rt, m3ga sb зеркала, m3ga ссылка megarcopen org, почему не работает m3ga, приложение сайта m3ga, http m3ga athttp m3ga at, http m3ga gl megarcopen org, m3ga al, m3ga block, m3ga fb, m3ga fu, m3ga gl зеркало официальный сайт, m3ga ink com, m3ga rc, m3ga сайт мориарти, megaweb3 at m3ga at mega gd, сайт https m3ga at
aviator играaviator на деньгиaviator играть на деньгислот aviator на деньгиaviator money game
aviator играaviator на деньгиaviator играть на деньгислот aviator на деньгиaviator money game
aviator играaviator на деньгиaviator играть на деньгислот aviator на деньгиaviator money game
aviator играaviator на деньгиaviator играть на деньгислот aviator на деньгиaviator money game
Привет романтикам и любителям казино!
Воспользуйтесь всеми возможностями Daddy Casino и получите 50 фриспинов для старта. Официальный сайт Daddy Casino доступен через рабочее зеркало. Играть можно в любое время, заходя через дэдди казино зеркало. Новым игрокам доступны щедрые бонусы Daddy Casino. Используйте промокоды Daddy Casino для максимальной выгоды.
Ссылка на Казино – [url=https://daddy-casino-lucky.ru]daddy casino официальный сайт вход[/url]
daddy casino зеркало
дэдди казино
daddy казино официальный сайт
Хороших гонораров!
Your article helped me a lot, is there any more related content? Thanks!
Hey there, amigo!Your article helped me a lot, is there any more related content? Thanks!
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
спасибо, интересное чтение
_________________
[url=https://igra.9xbk1.site]williams букмекерская контора[/url]
Официальная покупка диплома вуза с упрощенной программой обучения
futurerp.5nx.ru/viewtopic.php?f=43&t=148
Маркетплейс «Кракен» (кракен маркет) – один из самых популярных ресурсов в даркнете для приобретения различных товаров и услуг. Он доступен через браузер Tor, что обеспечивает пользователям анонимность и безопасность. Чтобы попасть на сайт, можно использовать официальные ссылки на «кракен маркетплейс» через проверенные зеркала, такие как kra12.at и kra12.cc.
Зеркала «кракен маркет» ([URL=’https://http-kra12.ru’]кракен зеркало[/URL]) созданы для того, чтобы обходить блокировки и сохранять доступ к сайту в случае ограничения работы основного домена. С помощью таких зеркал пользователи могут без проблем попасть на кракен маркетплейс и продолжить безопасно совершать покупки в даркнете.
Спасибо, долго искал
_________________
[URL=https://igra.vilkastavka.site]играть в игровые автоматы онлайн на деньги с выводом средств карту[/URL]
онлайн казино списком
список казино
[url=https://t.me/s/marathonbet_skachat]марафонбет мобильная[/url] — потому что ставки с дивана удобнее! Забудьте про блокировки и ищите своё приложение на главном экране. Никаких оправданий — только вы и спорт!
Urgent TON Crypto Inside information!
The Open Network (TON) has experienced a remarkable surge in popularity throughout 2024, with the number of token holders skyrocketing by 2400% to over 90 million. This growth has been particularly pronounced since June, with trading volumes reaching a peak of $2.4 billion in September.
Currently priced at $5.28, Toncoin (TON) has a market capitalization of $13.40 billion.
Insider information about TON will priced more than $20 (up to 400%)
For more information please see: https://ke1taro.com/crypto/
Free limited access code here:
crypto2
crypto3
crypto3
crypto4
crypto5
crypto4
crypto2
crypto4
crypto5
crypto1
crypto3
crypto4
свежие бездепы казино за регистрацию
свежие бездепозитные бонусы казино
Где Купить Мефедрон? САЙТ – KOKAIN.VIP Сколько стоит Мефедрон? САЙТ – KOKAIN.VIP
КУПИТЬ НА САЙТЕ – https://kokain.vip/
ЗАКАЗАТЬ ЧЕРЕЗ ТЕЛЕГРАММ- https://kokain.vip/
ДОСТАВКА В РУКИ – https://kokain.vip/
ЗАКЛАДКА ОНЛАЙН – https://kokain.vip/
ССЫЛКА В ТЕЛЕГРАММ – https://kokain.vip/
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
Теги поисковых слов для запроса “Мефедрон в Москве”
Где Купить Мефедрон в Москве? Как Купить закладку Мефедрона в Москве? Цена на Мефедрон в Москве? Купить Мефедрон с Доставкой в руки в Москве?
Сколько Стоит Мефедрон в Москве? Почему так просто Купить Мефедрон закладкой в Москве? Гарантия на Мефедрон в Москве? Купить Мефедрон с Гарантией?
Круглосуточные магазины Мефедрона в Москве? Оптовые и Розничные продажи Мефедрона в Москве? Купить Мефедрон в Москве через Телеграмм? Лучший Мефедрон Купить?
Купить Мефедрон в Москве по скидке и хорошей цене? Купить Мефедрон в Москве через свой телефон или ноутбук можно легко? Сколько где Мефедрона стоит цена?
Как купить Мефедрон в Москве если нет очень много денег и нужно угостить девушку? С кем можно разделить грамм Мефедрон в Москве?
Не плохой или хороший Мефедрон можно Купить в Москве на своей улице закладкой? Девушка угостила меня Хорошим Мефедроном в Москве из магазина?
Мои друзья любят употреблять Мефедрон в Москве днем вечером и ночью и потом не спят целые сутки под Мефедрон в Москве? Мой любимый Мефедрон на планете купить?
Мефедрон в Москве можно заказать с доставкой в руки через хорошего курьера прямо в теллеграмм и его привезут в руки без палева в Москве?
Мой Мефедрон в Москве можно на выставке показывать потому что такой Мефедрон в Москве никто и никогда не виде подобного качества тут просто нет?
Как хороший Мефедрон в Москве качественный провозят через границу из других стран чтоб люди радовались качеству Мефедрон в Москве? Очень купить Мефедрон советовали!
Как ведут себя люди когда употребляют хороший качественный Мефедрон в Москве чтоб не спалиться в черных очках, которые цветом как Мефедрон в Москве?
Могут ли принять мусора за Мефедрон в Москве если узнают что ты берешь его анонимно на сайте с гарантией который много лет продает Мефедрон в Москве?
Все люди по разному реагируют и задают себе вопрос, на который уже есть давно ответ – Как же купить Мефедрон в Москве если хочеться качественного Мефедрона?
Дополнительные теги – Купить Шишки в Москве, Купить экстази в Москве , Купить гашиш в Москве, Купить мефедрон в Москве, Купить экстази в Москве, Купить МДМА в Москве,
Купить лсд в Москве, Купить Амфетамин в Москве, Купить скорость альфа ПВП в Москве, Купить гидропонику в Москве, Купить метамфетамин в Москве, Купить эйфоретики в Москве,
Купить закладки в Москве, Купить ШИШКИ закладкой в Москве , Купить Стимуляторы в Москве, Купить Галлюцыногены в Москве, Купить Наркотики закладкой в Москве. Тег окончен
I’m not sure why but this website is loading incredibly slow for me.
Is anyone else having this problem or is it a issue on my end?
I’ll check back later and see if the problem still exists.
Удивительно, но купить диплом кандидата наук оказалось не так сложно
trading-labor.de/Покупка_диплома_с_доставкой_по_России
Howdy! Do you know if they make any plugins to help with Search Engine Optimization? I’m trying to get my blog
to rank for some targeted keywords but I’m not seeing very good gains.
If you know of any please share. Cheers!
Профессиональная установка окон — важный этап, от которого зависит долговечность и функциональность конструкции. OknaAlum предоставляет услуги монтажа, гарантируя высокое качество работ и полную герметичность. На сайте [url=https://oknaalum.ru/]oknaalum.ru[/url] вы сможете ознакомиться с нашими услугами и узнать, как мы обеспечиваем точную установку, чтобы окна служили вам долгие годы без потери функциональности. Доверьтесь профессионалам!
bar real estate Montenegro realestate
Вы устали от нескончаемых поисков нужных вам товаров в Интернете? Откройте для себя наилучший вариант с ссылка на мега тор! Благодаря обширному ассортименту категорий и свыше миллиона товаров мега сайт даркнет ссылка гарантирует, что вы найдете то, что вам нужно именно сейчас. Зарегистрируйтесь без излишних формальностей, не вводя свой email, и ощутите всю удобность анонимного шопинга. Отправляйтесь в mega at сейчас же и откройте для себя мир безграничных возможностей.
ссылка на мегу через тор
mg2:https://xn--meg-sb-dua.com
Casino is not just a game of luck; it’s a thrilling challenge that can be mastered with the right strategy. Imagine the excitement of hitting 21 and beating the dealer with confidence. Whether you’re a novice or an experienced player, learning [url=https://casinorealmoneyuk.com/]no deposit bonus at online casinos[/url] can transform your gaming experience and lead to significant wins. Don’t miss out on the opportunity to improve your skills and enjoy the rewards. Start playing blackjack today and take the first step towards becoming a pro!
Вам надоело жертвовать конфиденциальностью ради удобства? Mega ценит вашу анонимность! С помощью похоронного кода и зеркала TOR мега даркнет маркет ссылка гарантирует, что ваш просмотр останется незаметным и безопасным. Наслаждайтесь удобством выбора города для получения товара и изучайте разнообразие категорий. Присоединяйтесь к megaweb4 прямо сейчас, чтобы сделать покупки конфиденциальными и безопасными.
мега дарк нет
mega ссылка:https://xn--meg-sb-dua.com
Casino is not just a game of luck; it’s a thrilling challenge that can be mastered with the right strategy. Imagine the excitement of hitting 21 and beating the dealer with confidence. Whether you’re a novice or an experienced player, learning [url=https://casinorealmoneyuk.com/]online casino developer[/url] can transform your gaming experience and lead to significant wins. Don’t miss out on the opportunity to improve your skills and enjoy the rewards. Start playing blackjack today and take the first step towards becoming a pro!
Привет всем!
Долго думал как поднять параметры Ahrefs, Domain Rank, сайт и свои проекты и узнал >>>
Программы для линкбилдинга, такие как Xrumer, помогают ускорить создание ссылок. Прогон ссылок Хрумером способствует росту DR и улучшению показателей Ahrefs. Массовые рассылки ссылок через форумы улучшают видимость сайта в поисковых системах. Увеличение авторитетности сайта с помощью Xrumer происходит быстро. Используйте Xrumer для улучшения результатов.
Нашел крутых ребят, разработали недорогой и главное продуктивный прогон Xrumer – https://yandex.com/search/?text=%D0%BF%D1%80%D0%BE%D0%B3%D0%BE%D0%BD+bullet&lr=10466
Форумный постинг для новичков
Повышение авторитетности сайта
Прогон ссылок для повышения авторитета
Форумные проги для SEO
https://santamail.site/
Удачи и роста в топах!
[url=https://888starz.bet/]купить бомбу[/url]
Casino is not just a game of luck; it’s a thrilling challenge that can be mastered with the right strategy. Imagine the excitement of hitting 21 and beating the dealer with confidence. Whether you’re a novice or an experienced player, learning [url=https://casinorealmoneydk.com/]online casino real money[/url] can transform your gaming experience and lead to significant wins. Don’t miss out on the opportunity to improve your skills and enjoy the rewards. Start playing blackjack today and take the first step towards becoming a pro!
Casino is not just a game of luck; it’s a thrilling challenge that can be mastered with the right strategy. Imagine the excitement of hitting 21 and beating the dealer with confidence. Whether you’re a novice or an experienced player, learning [url=https://casinorealmoneydk.com/]princess casino[/url] can transform your gaming experience and lead to significant wins. Don’t miss out on the opportunity to improve your skills and enjoy the rewards. Start playing blackjack today and take the first step towards becoming a pro!
Your creativity never ceases to amaze me. Keep these awesome ideas coming! https://anapa-vip-transport.ru/
Полезная информация как купить диплом о высшем образовании без рисков
Wow, I can’t wait to try this at home. It looks so fun and easy!
https://24ekb-domasnij-internet-3.ru
мегафон интернет екатеринбург
This is such an informative post—thank you for sharing these amazing tips
https://dosug.oopssamara.ru/
провайдер мегафон
https://24ekb-domasnij-internet-3.ru
мегафон тарифы екатеринбург
Casino is not just a game of luck; it’s a thrilling challenge that can be mastered with the right strategy. Imagine the excitement of hitting 21 and beating the dealer with confidence. Whether you’re a novice or an experienced player, learning [url=https://ca.getb8.us/]best casino online site[/url] can transform your gaming experience and lead to significant wins. Don’t miss out on the opportunity to improve your skills and enjoy the rewards. Start playing blackjack today and take the first step towards becoming a pro!