Table of Contents
JavaScript arrays are versatile structures that allow you to store and manipulate collections of data. This cheat sheet provides a detailed guide to array properties, methods, and examples for each to help you get started or refresh your knowledge.
Array Properties
constructor
- Returns the constructor function for an array.
- Example 1:
let arr = [1, 2, 3];
console.log(arr.constructor); // Output: function Array() { [native code] }
- Example 2
let str = "Hello";
console.log(str.constructor); // Output: function String() { [native code] }
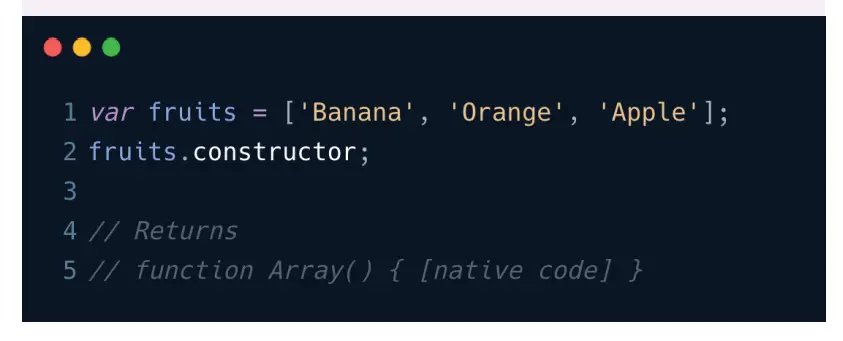
length
- Sets or returns the number of elements in an array.
- Example 1:
let arr = [1, 2, 3, 4];
console.log(arr.length); // Output: 4
- Example 2:
arr.length = 2;
console.log(arr); // Output: [1, 2]
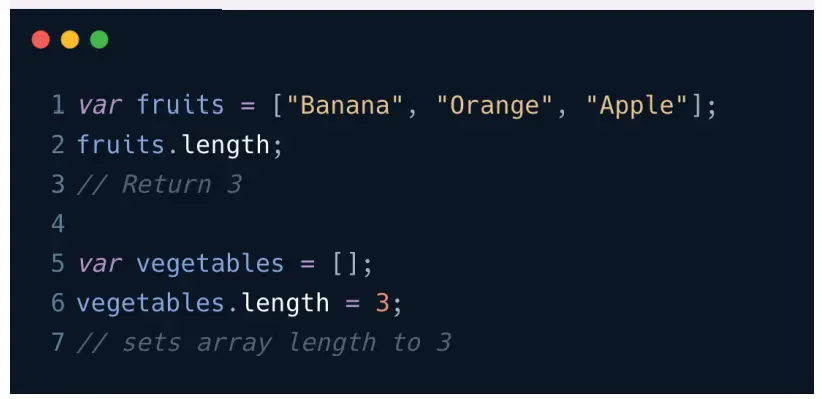
prototype
- Allows you to add new properties and methods to arrays.
- Example 1:
Array.prototype.sum = function() {
return this.reduce((acc, val) => acc + val, 0);
};
console.log([1, 2, 3].sum()); // Output: 6
- Example 2
Array.prototype.last = function() {
return this[this.length - 1];
};
console.log([1, 2, 3].last()); // Output: 3
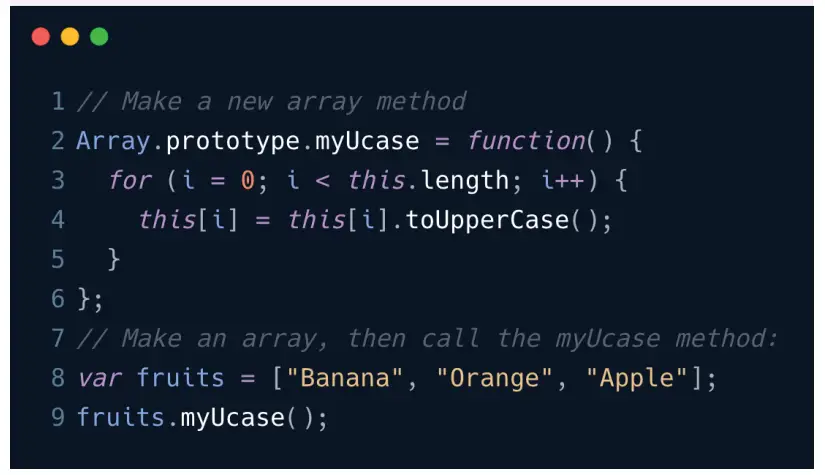
Array Methods
concat()
- Joins two or more arrays and returns a new array.
- Example 1
let arr1 = [1, 2];
let arr2 = [3, 4];
console.log(arr1.concat(arr2)); // Output: [1, 2, 3, 4]
- Example 2:
let arr3 = ['a', 'b'];
let arr4 = ['c', 'd'];
console.log(arr3.concat(arr4, [5, 6])); // Output: ['a', 'b', 'c', 'd', 5, 6]
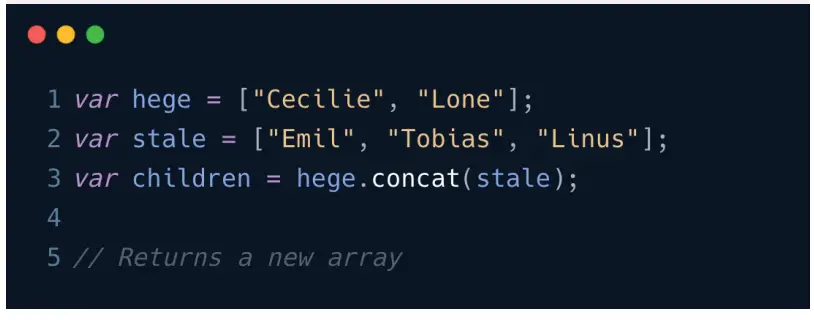
copyWithin()
- Copies array elements to another position in the array.
- Example 1
let arr = [1, 2, 3, 4, 5];
console.log(arr.copyWithin(0, 3)); // Output: [4, 5, 3, 4, 5]
- Example 2
let arr = ['a', 'b', 'c', 'd'];
console.log(arr.copyWithin(1, 2)); // Output: ['a', 'c', 'd', 'd']
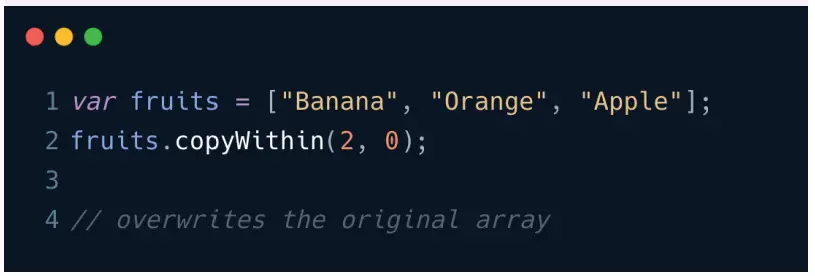
entries()
- Returns an Array Iterator object with key/value pairs.
- Example 1
let arr = ['a', 'b', 'c'];
for (let [index, value] of arr.entries()) {
console.log(index, value);
}
// Output:
// 0 'a'
// 1 'b'
// 2 'c'
- Example 2
let iterator = arr.entries();
console.log(iterator.next().value); // Output: [0, 'a']
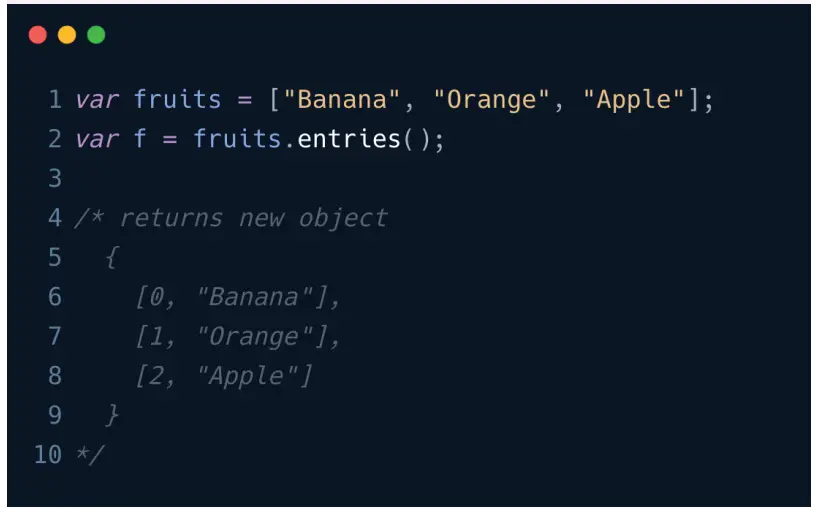
every()
- Tests whether all elements in an array pass a test.
- Example 1
let arr = [1, 2, 3, 4];
console.log(arr.every(val => val < 5)); // Output: true
- Example 2
let arr = [1, 2, 3, 4];
console.log(arr.every(val => val % 2 === 0)); // Output: false
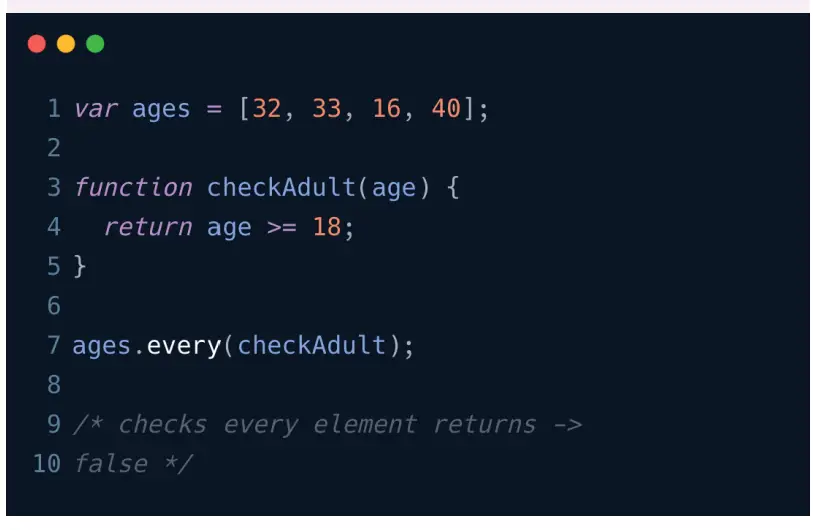
fill()
- Fills elements in an array with a static value.
- Example 1
let arr = [1, 2, 3, 4];
console.log(arr.fill(0, 2, 4)); // Output: [1, 2, 0, 0]
- Example 2:
let arr = Array(4);
console.log(arr.fill('a')); // Output: ['a', 'a', 'a', 'a']
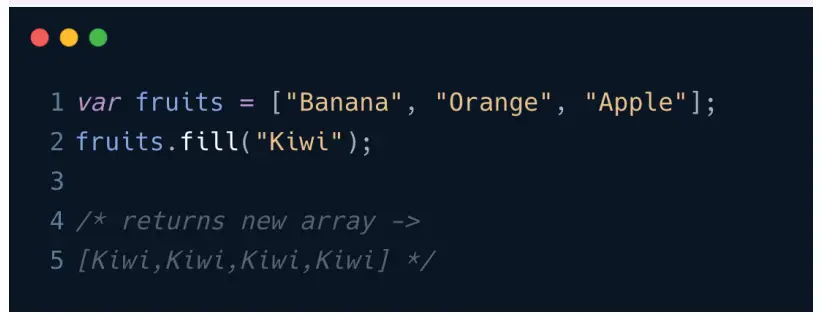
filter()
- Creates an array filled with elements that pass a test.
- Example 1
let arr = [1, 2, 3, 4];
console.log(arr.filter(val => val > 2)); // Output: [3, 4]
- Example 2
let arr = ['apple', 'banana', 'cherry'];
console.log(arr.filter(val => val.includes('a'))); // Output: ['apple', 'banana']
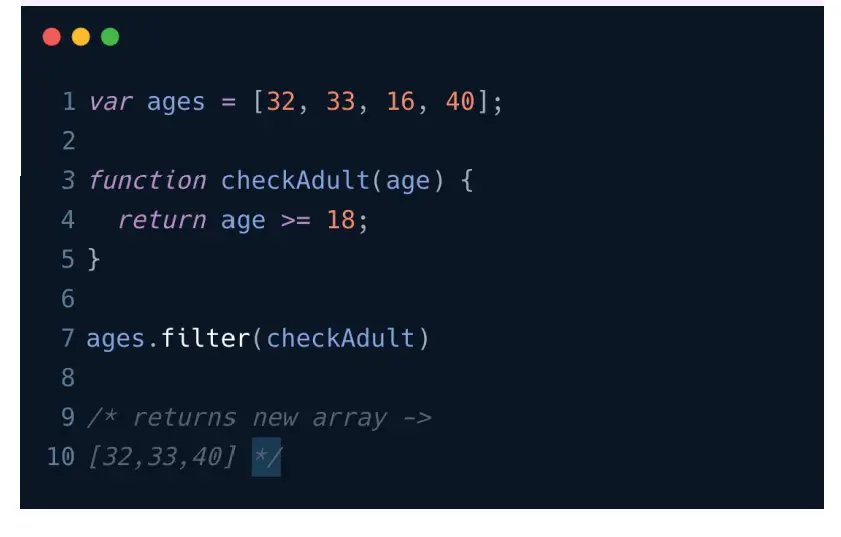
findIndex()
- Returns the index of the first element in an array that passes a test.
- Example 1
let arr = [1, 2, 3, 4];
console.log(arr.findIndex(val => val > 2)); // Output: 2
- Example 2
let arr = ['cat', 'dog', 'fish'];
console.log(arr.findIndex(val => val.length > 3)); // Output: 2
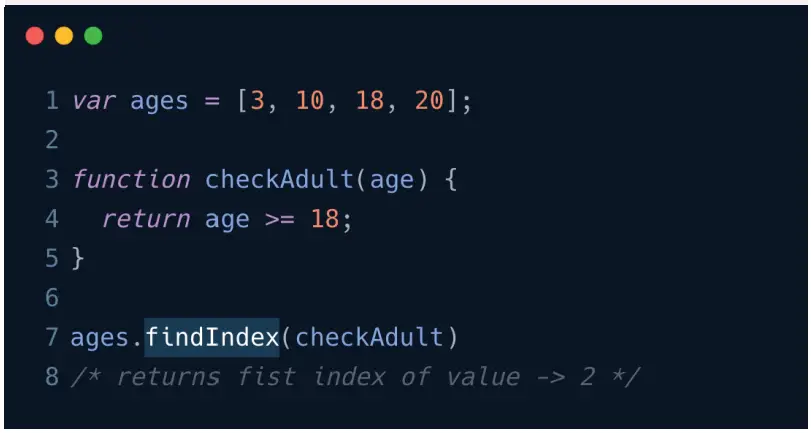
forEach()
- Calls a function once for each element in an array, in order.
- Example 1:
let arr = [1, 2, 3];
arr.forEach((value, index) => {
console.log(Index: ${index}, Value: ${value});
});
// Output:
// Index: 0, Value: 1
// Index: 1, Value: 2
// Index: 2, Value: 3
- Example 2
let fruits = ['apple', 'banana', 'cherry'];
fruits.forEach(fruit => console.log(fruit));
// Output: apple, banana, cherry
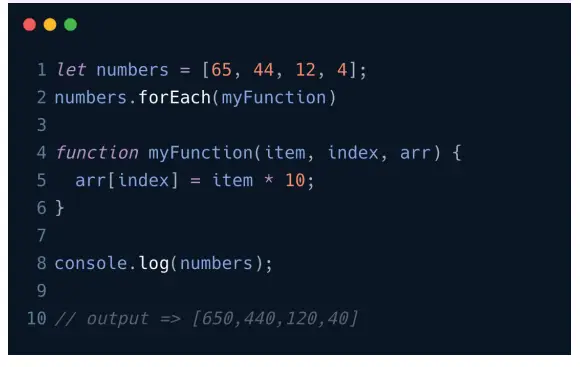
includes()
- Determines whether an array contains a specified element.
- Example 1:
let arr = [1, 2, 3];
console.log(arr.includes(2)); // Output: true
- Example 2
let names = ['John', 'Jane', 'Joe'];
console.log(names.includes('Jack')); // Output: false
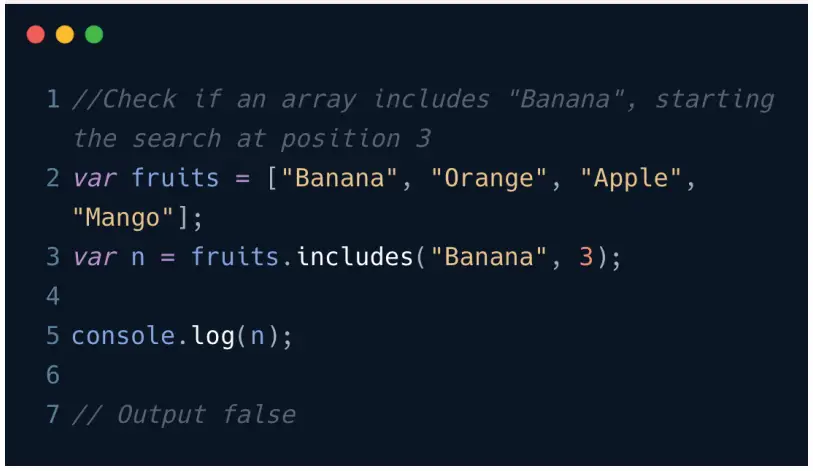
join()
- Converts all the elements of an array into a string.
- Example 1:
let arr = ['a', 'b', 'c'];
console.log(arr.join('-')); // Output: "a-b-c"
- Example 2
let numbers = [1, 2, 3];
console.log(numbers.join(', ')); // Output: "1, 2, 3"
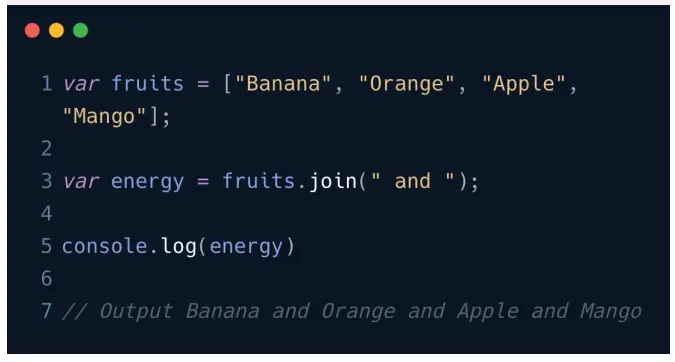
lastIndexOf()
- Searches the array for the specified item and returns its position (last occurrence).
- Example 1
let arr = [1, 2, 3, 2, 1];
console.log(arr.lastIndexOf(2)); // Output: 3
- Example 2
let chars = ['a', 'b', 'c', 'b', 'a'];
console.log(chars.lastIndexOf('b')); // Output: 3
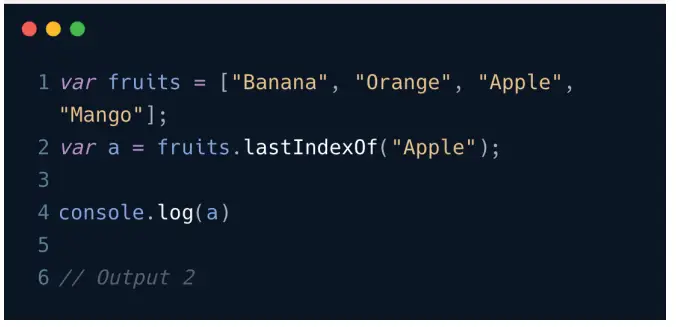
map()
- Creates a new array by calling a function on every element in the array.
- Example 1
let arr = [1, 2, 3];
let doubled = arr.map(x => x * 2);
console.log(doubled); // Output: [2, 4, 6]
- Example 2
let names = ['John', 'Jane'];
let greetings = names.map(name => Hello, ${name}!);
console.log(greetings); // Output: ["Hello, John!", "Hello, Jane!"]
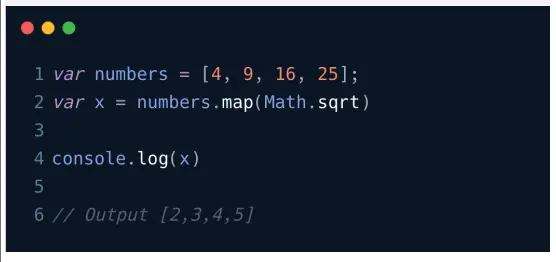
pop()
- Removes the last element of an array and returns that element.
- Example 1:
let arr = [1, 2, 3];
let last = arr.pop();
console.log(last); // Output: 3
console.log(arr); // Output: [1, 2]
- Example 2
let fruits = ['apple', 'banana', 'cherry'];
console.log(fruits.pop()); // Output: cherry
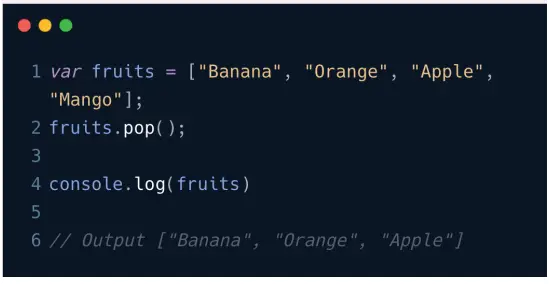
push()
- Adds new items to the end of an array and returns the new length.
- Example 1
let arr = [1, 2, 3];
console.log(arr.push(4)); // Output: 4
console.log(arr); // Output: [1, 2, 3, 4]
- Example 2
let fruits = ['apple'];
fruits.push('banana', 'cherry');
console.log(fruits); // Output: ['apple', 'banana', 'cherry']
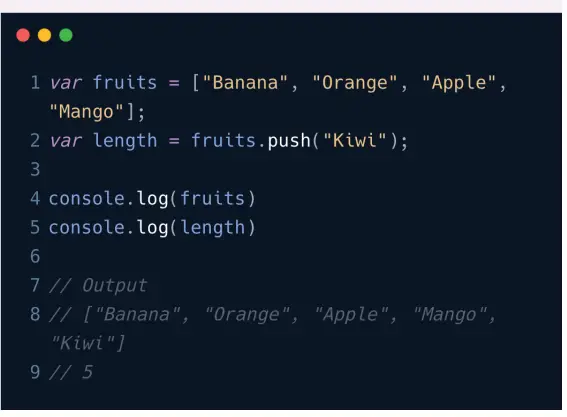
isArray()
- Determines whether an object is an array.
- Example 1
let arr = [1, 2, 3];
console.log(Array.isArray(arr)); // Output: true
- Example 2
let notArray = "hello";
console.log(Array.isArray(notArray)); // Output: false
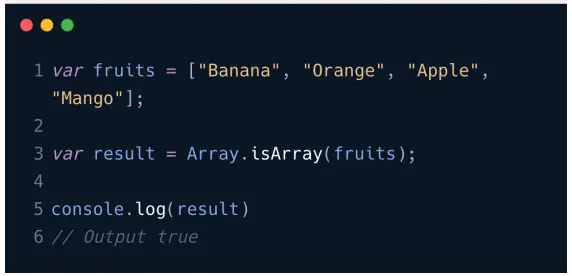
reduce()
- Reduces the array to a single value by executing a function for each value (from left to right).
- Example 1
let arr = [1, 2, 3];
let sum = arr.reduce((acc, val) => acc + val, 0);
console.log(sum); // Output: 6
- Example 2
let numbers = [5, 10, 15];
let product = numbers.reduce((acc, val) => acc * val, 1);
console.log(product); // Output: 750
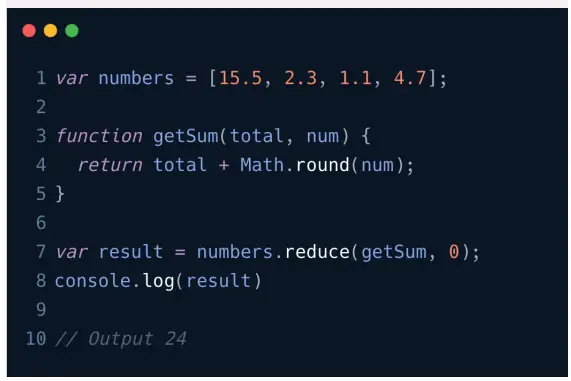
reduceRight()
- Reduces the array to a single value by executing a function for each value (from right to left).
- Example 1
let arr = [1, 2, 3];
let sum = arr.reduceRight((acc, val) => acc + val, 0);
console.log(sum); // Output: 6
- Example 2
let words = ["right", "from", "reduce"];
let sentence = words.reduceRight((acc, word) => acc + " " + word);
console.log(sentence); // Output: "reduce from right"
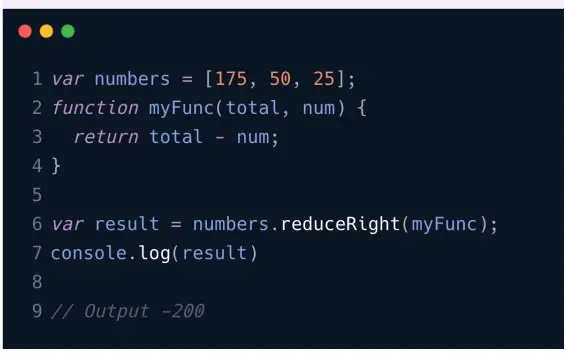
reverse()
- Reverses the order of elements in an array.
- Example 1
let arr = [1, 2, 3];
console.log(arr.reverse()); // Output: [3, 2, 1]
- Example 2
let chars = ['a', 'b', 'c'];
console.log(chars.reverse()); // Output: ['c', 'b', 'a']
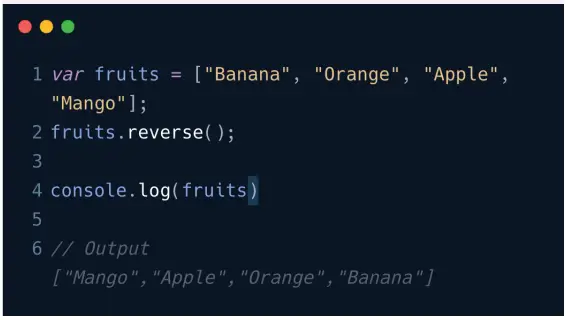
unshift()
The unshift() method adds new items to the beginning of
an array, and returns the new length.
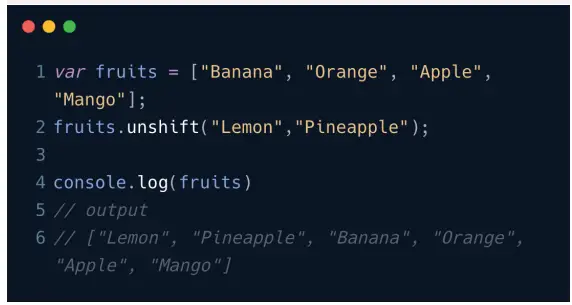
valueOf()
The valueOf() method returns the array. This method is the
default method of the array object. Array.valueOf() will
return the same as Array
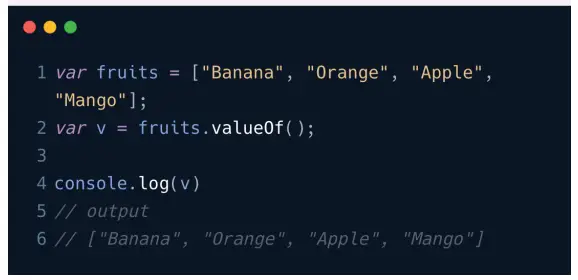
İçerik çiftleme SEO optimizasyonu, dijital pazarlama hedeflerimize ulaşmamıza yardımcı oldu. https://royalelektrik.com//esenyurt-elektrikci/
Üsküdar yer süzgeci tıkanıklığı Tıkanıklık sorunuyla karşılaştığımızda, bu firma ile çalışmak bize büyük bir rahatlama sağladı. Profesyonel ekip, sorunumuzu hızlıca çözdü ve gereksiz masraflardan kaçınmamıza yardımcı oldu.Kesinlikle tavsiye ederim! https://friend24.in/blogs/3182/%C3%9Csk%C3%BCdar-Kanal-T%C4%B1kan%C4%B1kl%C4%B1%C4%9F%C4%B1-A%C3%A7ma
Üsküdar acil tesisat hizmeti Su kaçağı konusunda gerçekten uzmanlar. Hizmetten çok memnun kaldım. https://onmybet.com/read-blog/25148
[…] Complete JavaScript Cheat Sheet: Arrays Post Views: 128 […]