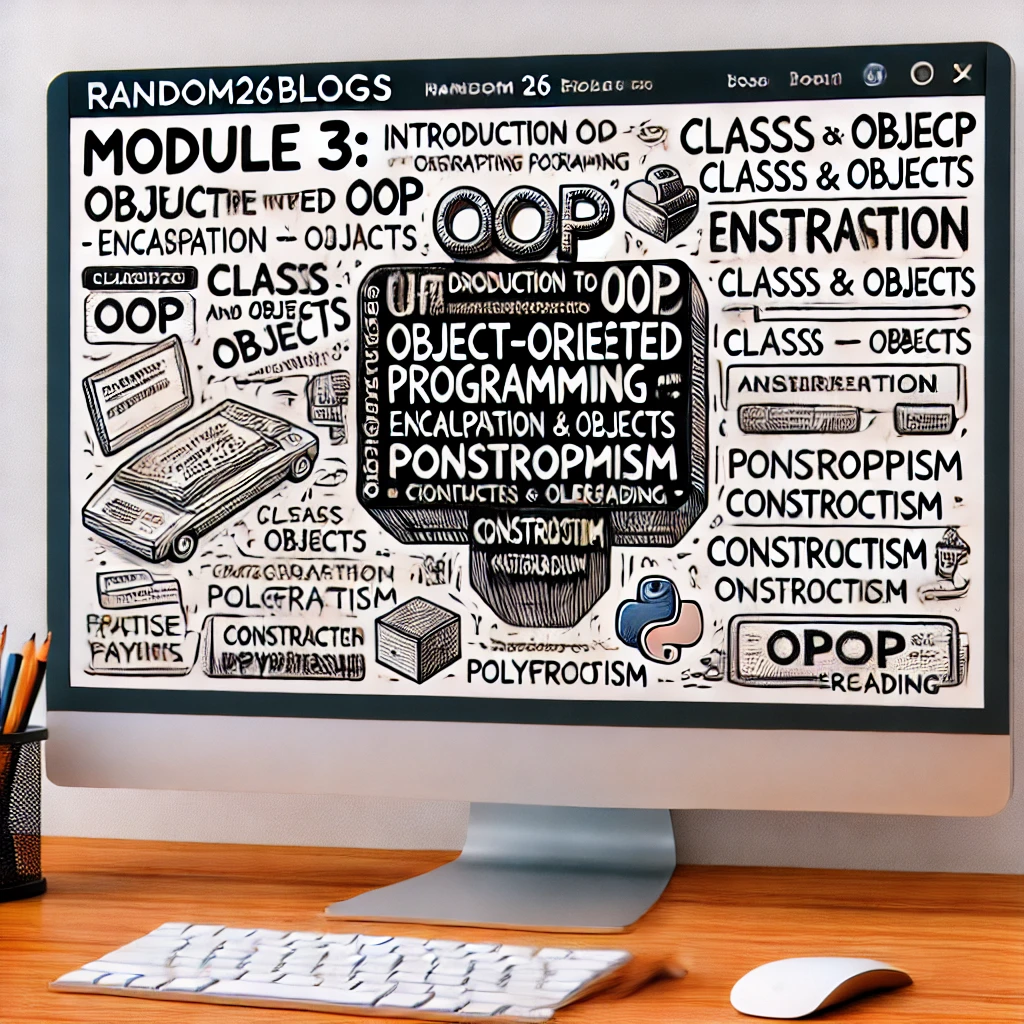
Introduction to OOP
Java (OOP) Object-Oriented Programming is a programming paradigm centered around objects and data rather than logic and functions. It helps developers create scalable, reusable, and manageable code. OOP revolves around four core principles: Encapsulation, Abstraction, Inheritance, and Polymorphism.
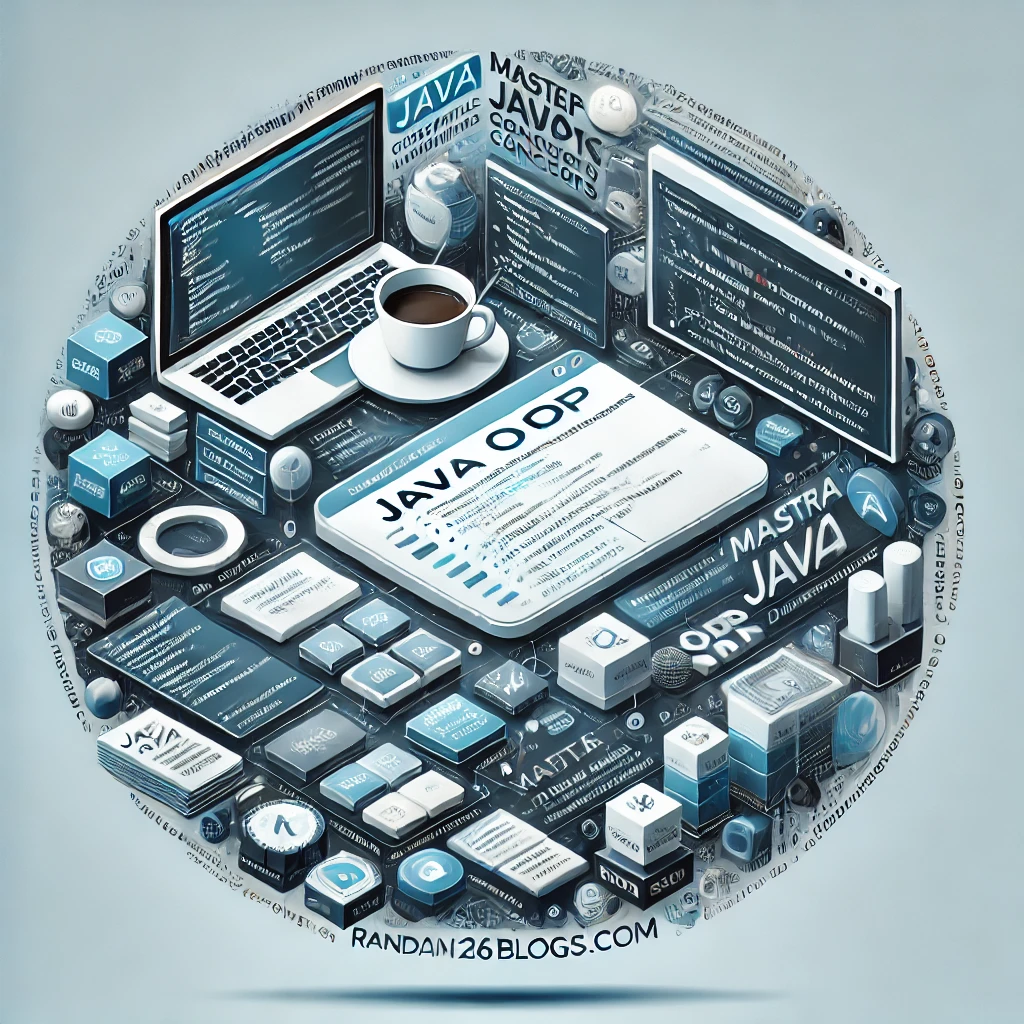
Table of Contents
Classes and Objects
Classes act as blueprints for objects. An object is an instance of a class containing both data (attributes) and methods (functions).
Example:
public class R26BCar {
String model;
int year;
void displayInfo() {
System.out.println("Model: " + model);
System.out.println("Year: " + year);
}
public static void main(String[] args) {
R26BCar car1 = new R26BCar();
car1.model = "Toyota";
car1.year = 2021;
car1.displayInfo();
}
}
Output: Model: Toyota
Year: 2021
Encapsulation
The meaning of Encapsulation, is to make sure that “sensitive” data is hidden from users. To achieve this, you must:
- declare class variables/attributes as
private
- provide public get and set methods to access and update the value of a
private
variable
Example:
public class R26BStudent {
private String name;
public void setName(String newName) {
name = newName;
}
public String getName() {
return name;
}
public static void main(String[] args) {
R26BStudent student = new R26BStudent();
student.setName("John");
System.out.println("Student Name: " + student.getName());
}
}
Output:
Student Name: John
Abstraction
Data abstraction is the process of hiding certain details and showing only essential information to the user.
Abstraction can be achieved with either abstract classes or interfaces (which you will learn more about in the next chapter).
The abstract
keyword is a non-access modifier, used for classes and methods:
- Abstract class: is a restricted class that cannot be used to create objects (to access it, it must be inherited from another class).
- Abstract method: can only be used in an abstract class, and it does not have a body. The body is provided by the subclass (inherited from).
Example:
abstract class R26BAnimal {
abstract void makeSound();
}
class R26BDog extends R26BAnimal {
void makeSound() {
System.out.println("Woof Woof!");
}
}
public class TestAbstraction {
public static void main(String[] args) {
R26BAnimal dog = new R26BDog();
dog.makeSound();
}
}
Output: Woof Woof!
Inheritance
In Java, it is possible to inherit attributes and methods from one class to another. We group the “inheritance concept” into two categories:
- subclass (child) – the class that inherits from another class
- superclass (parent) – the class being inherited from
To inherit from a class, use the extends
keyword.
Example:
class R26BVehicle {
void start() {
System.out.println("Vehicle is starting...");
}
}
class R26BCar extends R26BVehicle {
void honk() {
System.out.println("Car is honking: Beep Beep!");
}
}
public class TestInheritance {
public static void main(String[] args) {
R26BCar car = new R26BCar();
car.start();
car.honk();
}
}
Output: Vehicle is starting…
Car is honking: Beep Beep!
Polymorphism
Polymorphism allows methods to perform differently based on the context or object type.
Example:
class R26BCalculator {
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
}
public class TestPolymorphism {
public static void main(String[] args) {
R26BCalculator calc = new R26BCalculator();
System.out.println("Sum: " + calc.add(5, 3));
System.out.println("Sum: " + calc.add(5.5, 3.5));
}
}
Output: Sum: 8
Sum: 9.0
Constructors and Overloading
A constructor initializes objects, while overloading allows multiple methods with the same name but different parameters.
Example:
public class R26BBook {
String title;
R26BBook(String bookTitle) {
title = bookTitle;
}
void displayTitle() {
System.out.println("Book Title: " + title);
}
public static void main(String[] args) {
R26BBook book = new R26BBook("Java OOP Guide");
book.displayTitle();
}
}
Output: Book Title: Java OOP Guide
Funny and Challenging Practice Questions
- Inheritance Madness:
- Create a class
R26BPet
. Create subclassesR26BDog
andR26BCat
. Add aspeak()
method to each class with different sounds. Instantiate them and call their methods.
- Create a class
- Polymorphism Puzzle:
- Write a program where
R26BChef
has acook()
method. Overload the method to prepare different dishes when called with various arguments.
- Write a program where
- Encapsulation Enigma:
- Create a class
R26BWallet
. Ensure the balance is private and can only be accessed and modified using proper methods. Try updating the balance incorrectly and handle errors gracefully.
- Create a class
- Constructor Chaos:
- Design a
R26BGameCharacter
class where the constructor takes the name and initial score. Allow overloaded constructors with different initializations.
- Design a
Conclusion
Java OOP is a powerful approach to building modular, reusable, and maintainable code. Mastering concepts like classes, objects, encapsulation, abstraction, inheritance, and polymorphism is essential for every Java developer.
Start practicing now by solving the challenging R26B practice problems above and level up your Java skills!