Java is one of the most popular and versatile programming languages in the world. It is widely used for web development, enterprise applications, mobile apps, game development, and more. But how does Java work? How can you use it to create powerful applications? In this blog post, we will explore some of the basic concepts of Java, such as the JDK, libraries, and frameworks, and how they can help you write efficient code.
Table of Contents
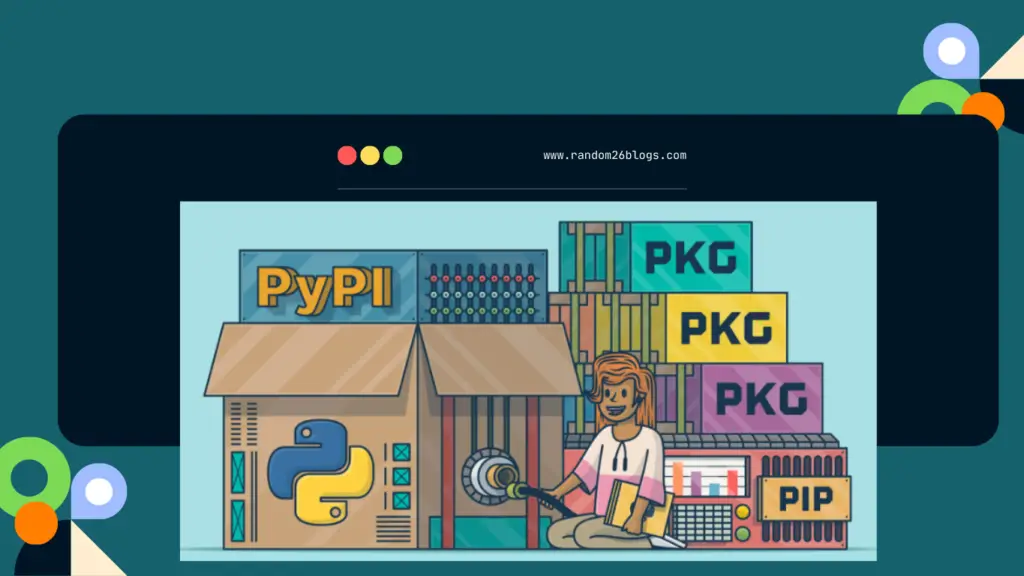
What is Java?
Java is a high-level, object-oriented, platform-independent programming language. It was created by James Gosling and released by Sun Microsystems in 1995. Known for its "Write Once, Run Anywhere" (WORA) philosophy, Java allows applications to run on any device with a Java Virtual Machine (JVM). Java has a simple yet robust syntax, making it easy to learn and use for developers of all levels. It is widely supported and features a comprehensive standard library, which includes classes and methods for tasks like file handling, networking, math, and more.
What is the JDK?
The Java Development Kit (JDK) is a software development environment used to create Java applications. It includes:
- Compiler (javac): Converts Java code into bytecode.
- Java Runtime Environment (JRE): Allows Java programs to run.
- Libraries: A collection of pre-written code (classes and methods) to simplify development.
- Utilities: Tools like
javadoc
,jar
, and more to assist in Java development.
Having the JDK installed is essential for developing Java applications. You can download it from the official Oracle website.
What are Libraries?
Libraries in Java are collections of pre-written classes and methods that help developers accomplish specific tasks without reinventing the wheel. For example:
- Apache Commons: For utilities like string manipulation and file handling.
- Google Guava: For collections, caching, and concurrency.
- Joda-Time: For date and time management (replaced by the modern Java
java.time
package).
Libraries allow developers to save time and focus on the core functionality of their applications.
What are Frameworks?
Frameworks are structures or templates that provide a foundation for building applications. They dictate the overall architecture and include pre-defined classes and methods to streamline development. Popular Java frameworks include:
- Spring: For enterprise applications and dependency injection.
- Hibernate: For database management and ORM (Object-Relational Mapping).
- JavaFX: For building rich user interfaces.
Using frameworks makes it easier to maintain code and implement complex functionalities with minimal effort.
How to Use Libraries in Java?
To use libraries in Java, you typically need to include their .jar
(Java ARchive) files in your project. Here's how:
- Add the Library: Download the
.jar
file and add it to your project's classpath. - Use the Classes: Import the necessary classes in your Java code. For example:
import org.apache.commons.lang3.StringUtils;
public class Main {
public static void main(String[] args) {
String reversed = StringUtils.reverse("Hello, World!");
System.out.println(reversed);
}
}
If you're using a build tool like Maven or Gradle, you can add dependencies directly to your pom.xml
or build.gradle
file.
How to Use Frameworks in Java?
Using frameworks involves configuring your project to follow the specific structure required by the framework. For instance, to use Spring:
- Add Dependencies: Use Maven or Gradle to add Spring libraries.
- Set Up Configuration: Use XML or annotations to configure beans and application properties.
- Write Code: Use Spring's pre-defined classes and annotations to build your application.
Example with Spring Boot:
javaCopy code
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
How to Create Your Own Library?
You can create your own library in Java by writing reusable classes and packaging them into a .jar
file. Here’s an example:
- Write your code:
- Compile the code:
- Create the
.jar
file: - Use the
.jar
file in your projects by adding it to the classpath.
public class MathUtils {
public static int add(int a, int b) {
return a + b;
}
}
jar cvf MathUtils.jar MathUtils.class
javac MathUtils.java
jar cvf MathUtils.jar MathUtils.class
Then, if you run this file as a script, you will see the following output:
python my_module.py
# Output:
This is my module
5
3
But if you import this module in another file or in the interactive interpreter, you will not see the output:
import my_module
# No output
ALL IN ALL
SO, WHAT WE HAVE explored the basics of Java, including the JDK, libraries, and frameworks. We’ve seen how to install and use libraries in Java projects, the importance of frameworks for efficient application development, and how to create your own libraries.
Java’s extensive ecosystem empowers developers to build versatile applications, whether for the web, mobile, or enterprise solutions. By mastering these concepts, you can harness the full potential of Java to create powerful and scalable applications.
We hope that this blog post has been helpful and informative for you. If you have any questions or feedback, please feel free to leave a comment below. Happy coding!