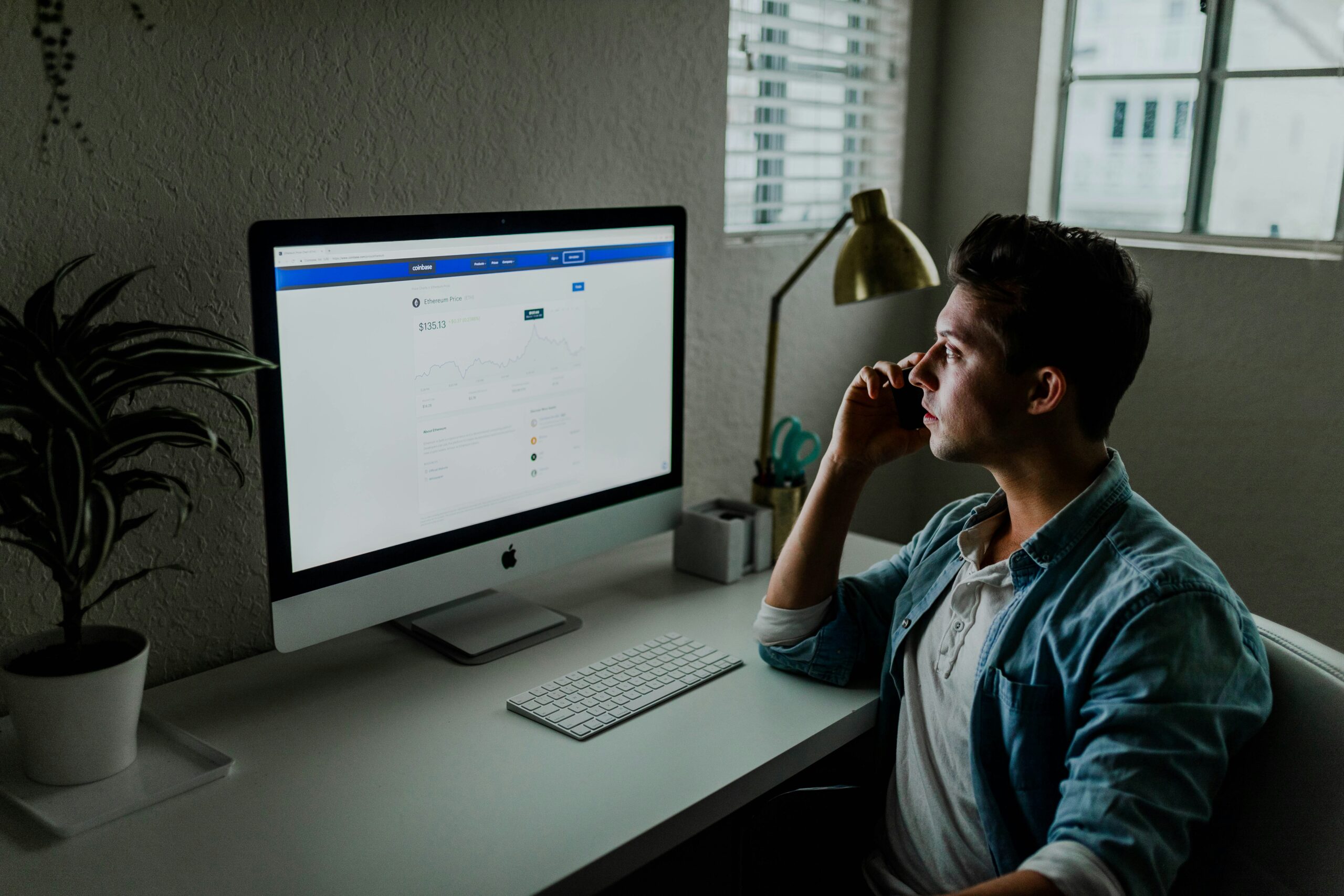
How to Improve Web App Performance Using the Web Worker API
Discover how to use the Web Worker API in JavaScript to improve web app performance. Learn how background threads can boost responsiveness and prevent UI freezes in your web applications.
In today’s fast-paced world of web development, performance is the key to delivering seamless user experiences. As web applications become more complex and data-intensive, developers often face the challenge of ensuring that their apps remain fast, responsive, and engaging. One powerful solution to improve performance and handle heavy tasks in the background is the Web Worker API in JavaScript.
In this guide, we’ll explore how Web Workers can help enhance your web app’s performance, prevent UI freezes, and allow you to run intensive tasks in the background without blocking the main thread. Whether you’re a beginner or an experienced developer, you’ll learn how to use this API effectively.
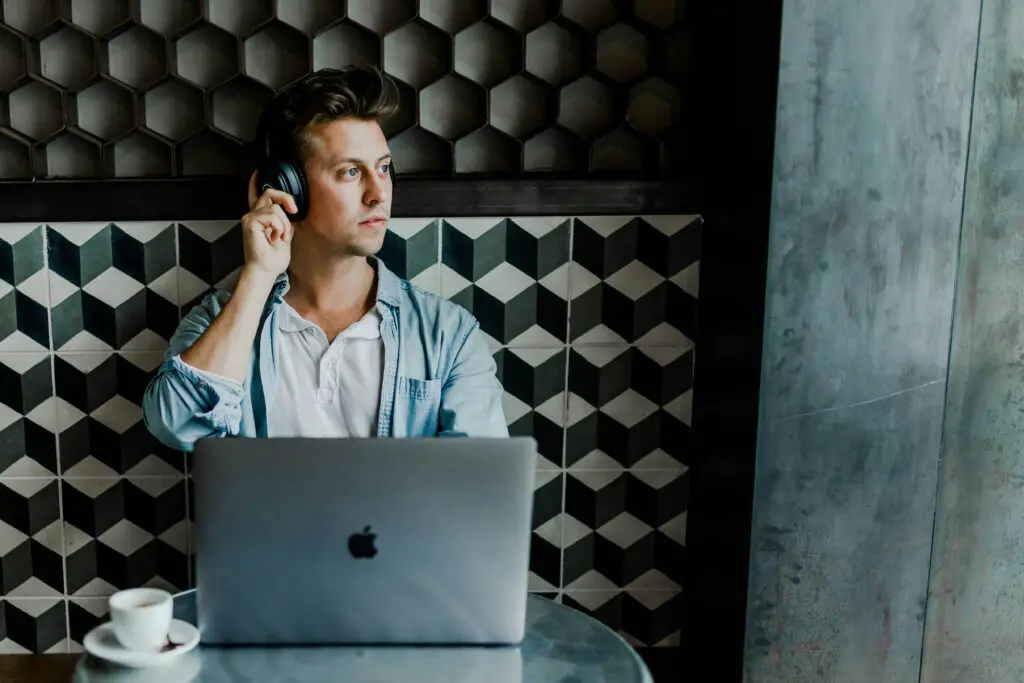
What is the Web Worker API in JavaScript?
The Web Worker API in JavaScript allows you to execute scripts on a background thread, separate from the main UI thread. This means you can offload CPU-intensive operations, like complex calculations, data processing, or image manipulation, to a separate thread while keeping your web app’s UI responsive.
By running tasks in parallel on background threads, Web Workers prevent the main thread from being blocked, thus avoiding UI freezes and enhancing the overall performance of your web applications.
Why Should You Use the Web Worker API?
1. Prevent UI Freezes
The most significant advantage of using Web Workers is that they prevent UI freezes. Long-running tasks like processing large amounts of data or performing complex calculations can block the main thread and make your app unresponsive. By using Web Workers, these tasks can run on separate threads, ensuring the UI remains interactive and smooth.
2. Boost Web App Performance
Web Workers help you improve performance by offloading resource-intensive tasks to background threads. This frees up the main thread, allowing it to handle UI rendering, user inputs, and interactions. This results in a faster and more responsive app.
3. Parallel Processing
With Web Workers, you can enable multi-threading in JavaScript, allowing tasks to run in parallel. This is ideal for CPU-intensive tasks that require processing large datasets or performing complex computations.
4. Better User Experience
The ultimate goal is to provide users with a responsive and fast web app. With Web Workers, your app can run heavy computations in the background, ensuring a smooth and responsive user experience.
How to Use the Web Worker API in JavaScript
Step 1: Creating a Web Worker
To begin using the Web Worker API, you need to create a new worker. You can do this by using the Worker
constructor and passing the script file to run in the background.
javascriptCopyEditconst worker = new Worker('worker.js');
This will create a new Web Worker and run the worker.js
file in the background.
Step 2: Send Messages to the Worker
To send data to the Web Worker, use the postMessage()
method. You can send any kind of data such as numbers, objects, or arrays.
javascriptCopyEditworker.postMessage({ data: 'Hello from main thread!' });
Step 3: Receive Messages from the Worker
To receive data back from the worker, listen for messages using the onmessage
event.
javascriptCopyEditworker.onmessage = function(event) {
console.log('Message from worker:', event.data);
};
Step 4: Terminate the Worker
After the task is completed, it’s essential to terminate the worker to free up resources.
javascriptCopyEditworker.terminate();
Example: Heavy Computation with Web Workers
Here’s an example where a heavy computation (such as summing a large range of numbers) is offloaded to a Web Worker.
Main Script (main.js)
javascriptCopyEditconst worker = new Worker('worker.js');
worker.postMessage({ number: 1000000 }); // Send data to worker
worker.onmessage = function(event) {
console.log('Result from worker:', event.data);
};
Worker Script (worker.js)
javascriptCopyEditself.onmessage = function(event) {
const number = event.data.number;
let result = 0;
// Simulate heavy computation
for (let i = 0; i < number; i++) {
result += i;
}
self.postMessage(result); // Send result back to main thread
};
In this example, the main script sends a large number to the worker. The worker performs a computation (summing all numbers up to 1,000,000) and sends the result back to the main thread, allowing the UI to remain responsive.
Limitations of Web Workers
While Web Workers are incredibly useful, they come with a few limitations that developers should keep in mind:
- No Direct DOM Access Web Workers run in a separate thread and don’t have access to the DOM or the
window
object. To interact with the DOM, you need to send messages between the worker and the main thread usingpostMessage()
. - Limited Browser Support Although most modern browsers support Web Workers, some older browsers may not. Be sure to check for compatibility if your app needs to support legacy browsers.
- Performance Overhead Creating too many workers can lead to performance overhead. It’s essential to use Web Workers judiciously to avoid excessive resource consumption. Too many active workers can slow down your app rather than enhance it.
Best Practices for Using Web Workers
- Use Web Workers for Heavy Computations: Offload tasks like complex calculations, large data processing, or image manipulation to background threads to keep your app responsive.
- Limit the Number of Workers: Avoid creating excessive Web Workers. If you need to process large datasets, consider using a limited number of workers or batch the tasks.
- Communicate Efficiently: Use
postMessage()
to communicate between the main thread and the worker. This helps in sending and receiving data without freezing the UI.
Web Worker API in JavaScript
The Web Worker API in JavaScript is a game-changer for web development. It allows you to run heavy tasks in the background without blocking the main thread, resulting in a smoother, more responsive web application. By embracing parallel processing, you can offload complex operations and improve overall performance.
As web apps become more dynamic and data-driven, using Web Workers will ensure that your application remains fast and efficient, offering a great user experience. So, take advantage of Web Workers and start optimizing your web app performance today!